LE Peripheral Extended ADV
The ble_bt5_peripheral sample demonstrates how to develop different Peripheral Role-based applications.
Supported features:
Send advertising events with LE Advertising Extensions.
Enable one or more advertising sets at the same time.
Enable advertising sets for a duration or the maximum number of extended advertising events.
Hold more data with extended advertising PDU s.
Can accept the establishment of an LE link and become a Peripheral role in the link on
LE 1M PHY
,LE 2M PHY
, orLE Coded PHY
.
Overview
Peripheral devices with LE Advertising Extensions can set the duration of the advertising or the maximum number of extended advertising events, and enable or disable one or more advertising sets at a time.
If extended advertising PDUs are used, the length of the advertising data or scan response data could be increased, and the distance that the connection can be established could be increased with the
LE Coded PHY
. More information about extended advertising PDUs and legacy advertising PDUs can be found in the chapter Configure Extended Advertising Related Parameters of LE Host.If the peripheral device uses LE Advertising Extensions, the developer shall take compatibility with peer devices of different Bluetooth versions into account. Compatibility with peer devices of different Bluetooth versions is shown below:
Bluetooth 5 Feature |
Advertising PDUs |
Bluetooth 4.0 |
Bluetooth 4.1 |
Bluetooth 4.2 |
Bluetooth 5.0 (Not Use LE Advertising Extensions) |
Bluetooth 5.0 (Use LE Advertising Extensions) |
---|---|---|---|---|---|---|
LE Advertising Extensions |
Extended advertising PDUs |
N |
N |
N |
N |
Y |
LE Advertising Extensions |
Legacy advertising PDUs |
Y |
Y |
Y |
Y |
Y |
Requirements
The sample supports the following development kits:
Hardware Platforms |
Board Name |
---|---|
RTL87x2G HDK |
RTL87x2G EVB |
To quickly set up the development environment, please refer to the detailed instructions provided in Quick Start.
Wiring
Please refer to RTL87x2G EVB Interfaces and Modules in Quick Start.
Configurations
Configurable Items
All contents that can be configured for the sample are in
samples\bluetooth\ble_bt5_peripheral\src\app_flags.h
,
developers can configure according to actual needs.
/** @brief Configure Advertising PHY */
#define ADVERTISING_PHY APP_PRIMARY_CODED_SECONDARY_CODED
/** @brief Configure coding scheme of LE Coded PHY: 0 - S = 2, 1 - S = 8 */
#define LE_CODED_PHY_S8 0
/** @brief Configure APP LE link number */
#define APP_MAX_LINKS 1
/** @brief Configure DLPS: 0 - Disable DLPS, 1 - Enable DLPS */
#define F_BT_DLPS_EN 1
Generating System Config File
Developers shall configure the following items through MP Tool:
Configurable Item |
Value |
---|---|
LE Slave Link Num |
≥ APP_MAX_LINKS |
For more information about MP Tool Configuration, please refer to Generating System Config File in Quick Start.
Building and Downloading
This sample can be found in the SDK folder:
Project file: samples\bluetooth\ble_bt5_peripheral\proj\rtl87x2g\mdk
Project file: samples\bluetooth\ble_bt5_peripheral\proj\rtl87x2g\gcc
To build and run the sample, follow the steps listed below:
Open project file.
To build the target, follow the steps listed in the Generating App Image in Quick Start.
After a successful compilation, the APP bin
app_MP_sdk_xxx.bin
will be generated in the directorysamples\bluetooth\ble_bt5_peripheral\proj\rtl87x2g\mdk\bin
.To download APP bin into the EVB, follow the steps listed in the MP Tool Download in Quick Start.
Press the reset button on the EVB and it will start running.
Experimental Verification
After downloading the sample bin to the EVB, developers can test it by using another kit that is running the LE Central Extended Scan sample.
Testing with Another Kit
For the test procedure, please refer to Testing with Another Kit in LE Central Extended Scan.
Code Overview
This chapter will be introduced according to the following several parts:
The directories of the project and source code files will be introduced in chapter Source Code Directory.
The Bluetooth Host will be introduced in chapter Bluetooth Host Overview.
The main function and configurable GAP parameters of this sample will be introduced in chapter Initialization.
The procedure of start extended advertising will be introduced in chapter Start Extended Advertising.
The GAP message handler of this sample will be introduced in chapter GAP Message Handler.
The GAP callback handler of this sample will be introduced in chapter GAP Callback Handler.
Source Code Directory
Project directory:
samples\bluetooth\ble_bt5_peripheral\proj
.Source code directory:
samples\bluetooth\ble_bt5_peripheral\src
.
Source files in ble_bt5_peripheral sample project are currently categorized into several groups as below.
└── Project: bt5_peripheral
└── secure_only_app
└── Device includes startup code
├── CMSE Library Non-secure callable library
├── Lib includes all binary symbol files that user application is built on
├── ROM_NS.lib
└── lowerstack.lib
└── rtl87x2g_sdk.lib
└── gap_utils.lib
├── peripheral includes all peripheral drivers and module code used by the application
├── Profile includes LE profiles or services used by the sample application
└── APP includes the ble_bt5_peripheral user application implementation
├── app_task.c
├── main.c
├── bt5_peripheral_stack_api.c
└── bt5_peripheral_app.c
The sample uses default GAP LIB that matches with bt_host_0_0
, please refer to Usage of GAP LIB in
Host Image
for more information.
Bluetooth Host Overview
The sample uses default Bluetooth Host image in bt_host_0_0
, please refer to
Host Image
for more information.
For the details of bluetooth technology features supported by the Bluetooth Host, please refer to the file
bin\rtl87x2g\bt_host_image\bt_host_0_0\bt_host_config.h
.
Initialization
main()
function is invoked when the EVB is powered on and the chip is reset,
and it performs the following initialization functions:
int main(void)
{
board_init();
le_gap_init(APP_MAX_LINKS);
gap_lib_init();
app_le_gap_init();
pwr_mgr_init();
task_init();
os_sched_start();
return 0;
}
le_gap_init()
function is used to initialize GAP and configure link number.app_le_gap_init()
function is used to initialize the GAP parameters. Developers can configure the following parameters: Device name and device appearance, GAP Bond Manager parameters, LE Advertising Extensions parameters (Please refer to the chapter Configure Device Name and Device Appearance, Configure Bond Manager Parameters, Configure LE Advertising Extensions Parameters, Configure Other Parameters of LE Host).
void app_le_gap_init(void)
{
/* Device name and device appearance */
......
/* GAP Bond Manager parameters */
......
/* LE Advertising Extensions parameters */
bool use_extended = true;
/* Set device name and device appearance */
le_set_gap_param(GAP_PARAM_DEVICE_NAME, GAP_DEVICE_NAME_LEN, device_name);
le_set_gap_param(GAP_PARAM_APPEARANCE, sizeof(appearance), &appearance);
le_set_gap_param(GAP_PARAM_SLAVE_INIT_GATT_MTU_REQ, sizeof(slave_init_mtu_req),
&slave_init_mtu_req);
le_set_gap_param(GAP_PARAM_DEFAULT_DATA_LEN_MAX_TX_OCTETS, sizeof(max_data_len_tx_oct),
&max_data_len_tx_oct);
le_set_gap_param(GAP_PARAM_DEFAULT_DATA_LEN_MAX_TX_TIME, sizeof(max_data_len_tx_time),
&max_data_len_tx_time);
/* Setup the GAP Bond Manager */
gap_set_param(GAP_PARAM_BOND_PAIRING_MODE, sizeof(auth_pair_mode), &auth_pair_mode);
gap_set_param(GAP_PARAM_BOND_AUTHEN_REQUIREMENTS_FLAGS, sizeof(auth_flags), &auth_flags);
gap_set_param(GAP_PARAM_BOND_IO_CAPABILITIES, sizeof(auth_io_cap), &auth_io_cap);
gap_set_param(GAP_PARAM_BOND_OOB_ENABLED, sizeof(auth_oob), &auth_oob);
le_bond_set_param(GAP_PARAM_BOND_FIXED_PASSKEY, sizeof(auth_fix_passkey), &auth_fix_passkey);
le_bond_set_param(GAP_PARAM_BOND_FIXED_PASSKEY_ENABLE, sizeof(auth_use_fix_passkey),
&auth_use_fix_passkey);
le_bond_set_param(GAP_PARAM_BOND_SEC_REQ_REQUIREMENT, sizeof(auth_sec_req_flags),
&auth_sec_req_flags);
/* Use LE Advertising Extensions */
le_set_gap_param(GAP_PARAM_USE_EXTENDED_ADV, sizeof(use_extended), &use_extended);
/* Initialize extended advertising-related parameters */
le_init_ext_adv_params_ext_conn();
/* Register gap message callback */
le_register_app_cb(app_gap_callback);
}
More information on LE GAP initialization and startup flow can be found in the chapter GAP Parameters Initialization of LE Host.
Start Extended Advertising
Due to support of the LE Advertising Extensions, the device can use one or more advertising sets. The sample needs to create an advertising handle to identify the advertising set, and extended advertising-related parameters are configured for a specified advertising set. Using LE Advertising Extensions, the sample can choose to send legacy advertising PDUs ( LE Peripheral sample can only send legacy advertising PDUs) or extended advertising PDUs by modifying advertising event properties.
The flow of starting extended advertising is shown as below. Optional Procedure is determined by advertising event properties.
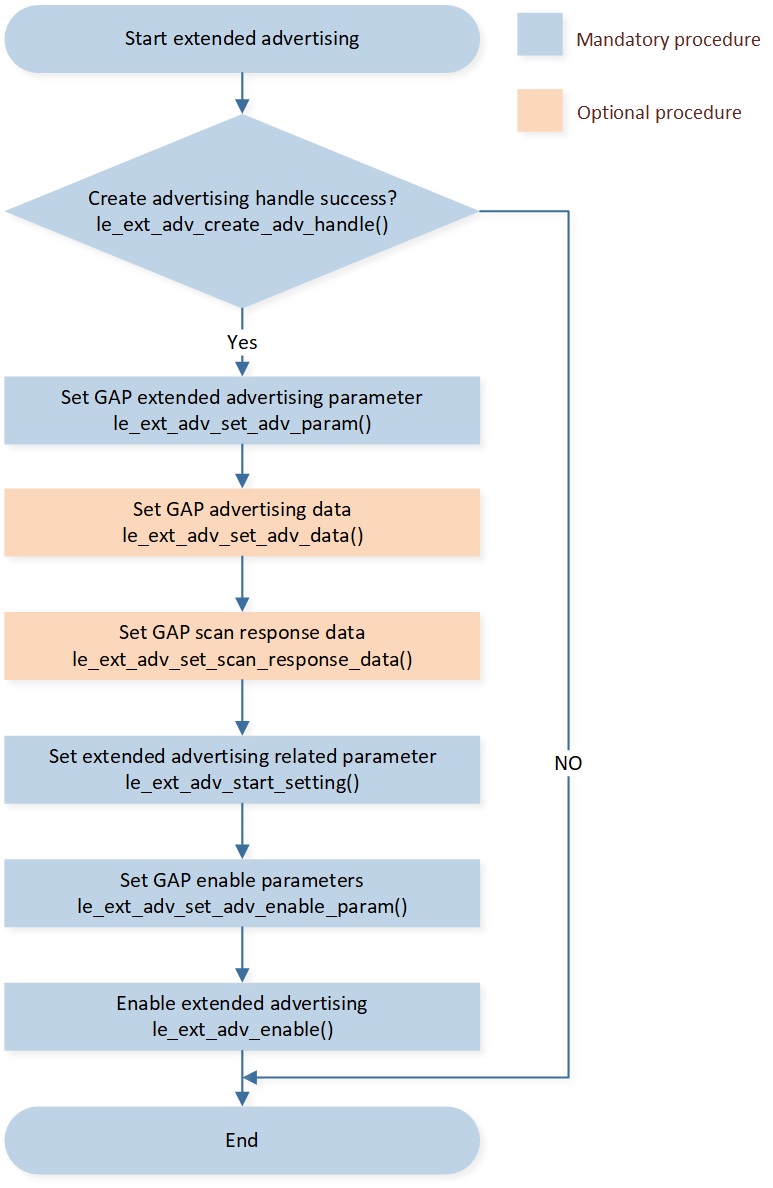
Start Extended Advertising Flow
GAP Message Handler
app_handle_gap_msg()
function is invoked whenever a GAP message is received from the Bluetooth Host.
More information on GAP messages can be found in the chapter Bluetooth LE GAP Message of LE Host.
void app_handle_gap_msg(T_IO_MSG *p_gap_msg)
{
T_LE_GAP_MSG gap_msg;
uint8_t conn_id;
memcpy(&gap_msg, &p_gap_msg->u.param, sizeof(p_gap_msg->u.param));
APP_PRINT_TRACE1("app_handle_gap_msg: subtype %d", p_gap_msg->subtype);
switch (p_gap_msg->subtype)
{
case GAP_MSG_LE_DEV_STATE_CHANGE:
{
app_handle_dev_state_evt(gap_msg.msg_data.gap_dev_state_change.new_state,
gap_msg.msg_data.gap_dev_state_change.cause);
}
break;
.......
}
void app_handle_dev_state_evt(T_GAP_DEV_STATE new_state, uint16_t cause)
{
APP_PRINT_INFO3("app_handle_dev_state_evt: init state %d, ADV state %d, cause 0x%x",
new_state.gap_init_state, new_state.gap_adv_state, cause);
if (gap_dev_state.gap_init_state != new_state.gap_init_state)
{
if (new_state.gap_init_state == GAP_INIT_STATE_STACK_READY)
{
APP_PRINT_INFO0("GAP stack ready");
/* Stack ready */
......
/* Set extended advertising related parameters */
le_ext_adv_start_setting(adv_handle, EXT_ADV_SET_AUTO);
}
}
gap_dev_state = new_state;
}
This sample will call le_ext_adv_start_setting()
to set extended advertising related parameters when it receives GAP_INIT_STATE_STACK_READY
message. EXT_ADV_SET_AUTO
means that extended advertising related parameters (including advertising parameters, advertising data and scan response data) for the specified advertising set will be set automatically according to advertising event properties. Otherwise, developers could set flags according to advertising event properties with Table Extended Advertising Parameters Setting with Legacy Advertising PDUs or Table Extended Advertising Parameters Setting with Extended Advertising PDUs.
void app_handle_dev_state_evt(T_GAP_DEV_STATE new_state, uint16_t cause)
{
APP_PRINT_INFO3("app_handle_dev_state_evt: init state %d, ADV state %d, cause 0x%x",
new_state.gap_init_state, new_state.gap_adv_state, cause);
if (gap_dev_state.gap_init_state != new_state.gap_init_state)
{
if (new_state.gap_init_state == GAP_INIT_STATE_STACK_READY)
{
APP_PRINT_INFO0("GAP stack ready");
/* Stack ready */
......
/* Set extended advertising related parameters */
le_ext_adv_start_setting(adv_handle, EXT_ADV_SET_AUTO);
}
}
gap_dev_state = new_state;
}
The sample will receive extended advertising state messages after le_ext_adv_enable()
is invoked in app_gap_callback()
.
More information about handling extended advertising state messages can be found in the chapter Extended Advertising State of LE Host. The sample will call le_ext_adv_enable()
to enable the specified advertising set when it receives GAP_CONN_STATE_DISCONNECTED
. After disconnection, the sample will be restored to a status that is discoverable and connectable again.
void app_handle_conn_state_evt(uint8_t conn_id, T_GAP_CONN_STATE new_state, uint16_t disc_cause)
{
APP_PRINT_INFO4("app_handle_conn_state_evt: conn_id %d old_state %d new_state %d, disc_cause 0x%x",
conn_id, gap_conn_state, new_state, disc_cause);
switch (new_state)
{
case GAP_CONN_STATE_DISCONNECTED:
{
if ((disc_cause != (HCI_ERR | HCI_ERR_REMOTE_USER_TERMINATE))
&& (disc_cause != (HCI_ERR | HCI_ERR_LOCAL_HOST_TERMINATE)))
{
APP_PRINT_ERROR1("app_handle_conn_state_evt: connection lost cause 0x%x", disc_cause);
}
/* Enable one advertising set */
le_ext_adv_enable(1, &adv_handle);
}
break;
......
}
gap_conn_state = new_state;
}
GAP Callback Handler
app_gap_callback()
function is used to handle GAP callback messages.
More information on GAP callback can be found in the chapter Bluetooth LE GAP Callback of LE Host.
le_ext_adv_start_setting()
is invoked after the Bluetooth Host is ready, then the result of setting the extended advertising parameters will be returned by the app_gap_callback()
with the cb_type GAP_MSG_LE_EXT_ADV_START_SETTING
. If the result is a success, the sample will enable extended advertising.
First, the sample sets the GAP extended advertising enable parameters which determine whether to stop extended advertising when the duration has expired or when the maximum number of extended advertising events has been reached. Then, the sample calls le_ext_adv_enable()
to enable extended advertising for one advertising set. Sample codes are listed below:
T_APP_RESULT app_gap_callback(uint8_t cb_type, void *p_cb_data)
{
T_APP_RESULT result = APP_RESULT_SUCCESS;
T_LE_CB_DATA *p_data = (T_LE_CB_DATA *)p_cb_data;
APP_PRINT_TRACE1("app_gap_callback: cb_type = 0x%x", cb_type);
switch (cb_type)
{
case GAP_MSG_LE_EXT_ADV_START_SETTING:
APP_PRINT_INFO3("GAP_MSG_LE_EXT_ADV_START_SETTING:cause 0x%x, flag 0x%x, adv_handle %d",
p_data->p_le_ext_adv_start_setting_rsp->cause, p_data->p_le_ext_adv_start_setting_rsp->flag,
p_data->p_le_ext_adv_start_setting_rsp->adv_handle);
if (p_data->p_le_ext_adv_start_setting_rsp->cause == GAP_CAUSE_SUCCESS)
{
/* Initialize enable parameters */
le_init_ext_adv_enable_params(p_data->p_le_ext_adv_start_setting_rsp->adv_handle);
/* Enable one advertising set */
le_ext_adv_enable(1, &p_data->p_le_ext_adv_start_setting_rsp->adv_handle);
}
break;
......
}
}
Extended advertising state message will be handled in app_handle_gap_msg()
function. The result of enabling advertising set will be returned by app_gap_callback()
with cb_type GAP_MSG_LE_EXT_ADV_ENABLE
. Sample codes are listed as below:
T_APP_RESULT app_gap_callback(uint8_t cb_type, void *p_cb_data)
{
T_APP_RESULT result = APP_RESULT_SUCCESS;
T_LE_CB_DATA *p_data = (T_LE_CB_DATA *)p_cb_data;
APP_PRINT_TRACE1("app_gap_callback: cb_type = 0x%x", cb_type);
switch (cb_type)
{
case GAP_MSG_LE_EXT_ADV_ENABLE:
APP_PRINT_INFO1("GAP_MSG_LE_EXT_ADV_ENABLE:cause 0x%x", p_data->le_cause.cause);
break;
......
}
}
When LE peripheral extended advertising sample project is run on the EVB, the LE device becomes discoverable and connectable. A remote device can scan the peripheral device and create a connection with the peripheral device.