Console
This document provides information about the design philosophy and operating principle of the console subsystem. The console provides a universal and scalable command line interface for users and instruments. It offers several benefits for debugging, certification, and automating tests. The figure below shows the position of the console in the system. Console is above the transport adaptor layer and provides common send and receive interface for the application layer.
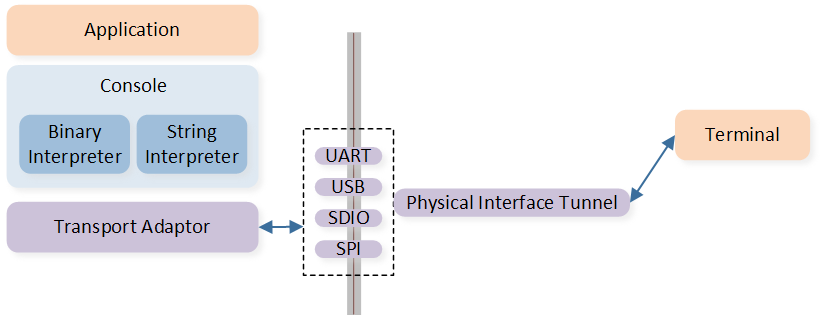
Console Subsystem
Console Library Introduction
The functions of console are provided by the console library. The library directory and header files directory are shown as follows.
IC Type |
Library Directory |
Header Files Directory |
---|---|---|
|
|
|
|
|
If application wants to use the console, the console.lib
and the header files shall be included in the project.
Console Mode
The console provides two switchable command processing modes, binary mode and string mode.
The application layer can set mode using console_set_mode()
.
Binary Mode
In binary mode, when the application layer registers the console parser using console_register_parser()
, the binary command which the user inputs can be parsed.
String Mode
In string mode, when the application layer registers the command passed using cli_cmd_register()
, the string command which the user inputs can be parsed.
Command line interface is a text-based user interface that enables interaction with a computer system. It allows users to input specific commands, each leading the device to execute corresponding operations. CLI provides a prompt where the user types a command, which is expressed as a sequence of characters. A command name is followed by some parameters, and the user presses the Enter key to execute that command.
Users can register a series of CLI commands as a complete module. The module should include a main command, which should be registered by cli_cmd_register()
, to enter the module and some sub-commands supported by that module. The format of the command is defined in T_CLI_CMD
in inc\framework\console\cli.h
.
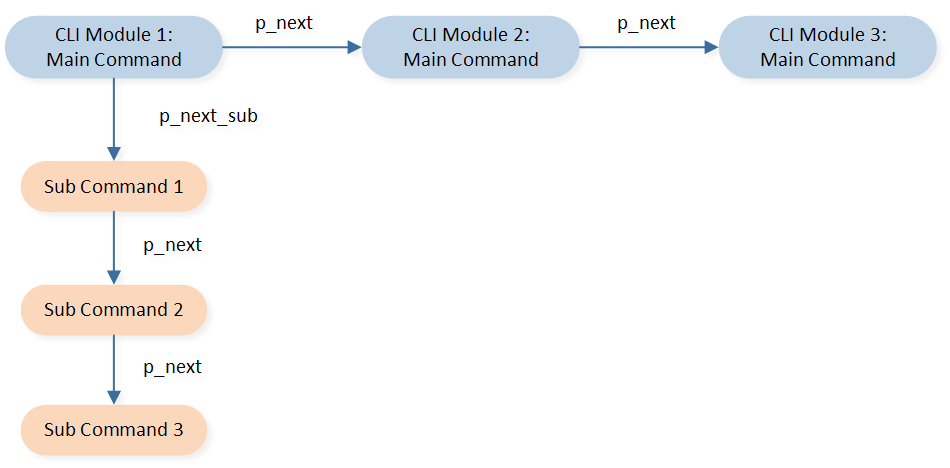
CLI Command Relatetion
typedef struct t_cli_cmd
{
const struct t_cli_cmd *p_next; /**< The next command to be linked. */
const struct t_cli_cmd *p_next_sub; /**< The next subcommand to be linked. */
const char *const p_cmd; /**< The command that causes p_callback to be executed. */
const char *const p_help; /**< String that describes how to use the command. */
const P_CLI_CALLBACK p_callback; /**< The command callback that will generate output. */
int32_t param_num; /**< The command expects a fixed number of parameters. */
const P_CLI_MATCH p_match; /**< The command pattern matching function;
NULL if using default match pattern. */
} T_CLI_CMD;
The
p_next
field refers to the next command, which is set to null for the main command and points to the next sub-command for the sub-command. The sub-commands are linked through thep_next
field.The
p_next_sub
field points to the first sub-command (usually the help command) for the main command. The main command and the first sub-command are linked through thep_next_sub
field.The
p_cmd
field contains the command text used in the serial communication software.The
p_help
field provides a description of the command’s functionality.The
p_callback
field points to the callback function associated with the command, which would be executed when the command is invoked.The
param_num
field represents the number of parameters supported by the command.The
p_match
field is a matching function used to match the input text with the command. In most cases, setting it to null will use the default matching method.
Console API Introduction
Console Init
static void app_console_init(void)
{
T_CONSOLE_PARAM console_param;
T_CONSOLE_OP console_op;
console_param.tx_buf_size = 512;
console_param.rx_buf_size = 512;
console_param.tx_wakeup_pin = MAP_DEMO_UART_TX;
console_param.rx_wakeup_pin = MAP_DEMO_UART_RX;
console_op.init = console_uart_init;
console_op.tx_wakeup_enable = NULL;
console_op.rx_wakeup_enable = NULL;
console_op.write = console_uart_write;
console_op.wakeup = console_uart_wakeup;
console_init(&console_param, &console_op);
console_set_mode(CONSOLE_MODE_STRING);
}