视图 (View)
视图控件 (View) 是一种切换更为便利的容器控件,通过事件响应(点击以及四个方向的滑动等)可以实时创建任一视图控件并可选择多种切换效果。切换过程中内存中会存在两个view,切换完成后会自动清理未显示的view,可以有效降低内存消耗。
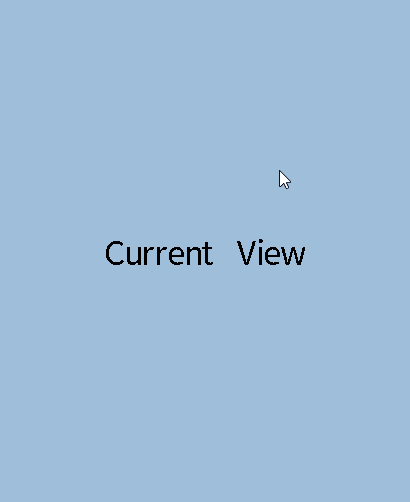
用法
注册视图控件描述子
使用 gui_view_descriptor_register()
函数传入视图控件描述子地址将其注册在描述子列表中供其它视图控件读取使用,作为创建视图控件的参数,其中 gui_view_descriptor
结构体定义如下:
typedef struct gui_view_descriptor
{
const char *name;
gui_view_t **pView;
void (* on_switch_in)(gui_view_t *view); // callback function when view is switched in and created
void (* on_switch_out)(gui_view_t
*view); // callback function when view is switched out and destroyed
uint8_t keep : 1;
} gui_view_descriptor_t; // if keep is true, the view will not be destroyed when switch to other view and will be created when register view
获得视图控件描述子
使用 gui_view_descriptor_get()
函数通过传入字符串获取对应 name
的视图控件描述子。
创建视图控件
使用 gui_view_create()
函数可以根据描述子创建一个视图控件。
设置视图切换事件
使用 gui_view_switch_on_event()
设置视图切换事件,对某一个事件可以重复设置,会使用最新的描述子。具体的事件类型包括 GUI_EVENT_TOUCH_CLICKED
、 GUI_EVENT_KB_SHORT_CLICKED
、 GUI_EVENT_TOUCH_MOVE_LEFT
、 GUI_EVENT_TOUCH_MOVE_RIGHT
等。具体的切换风格请参考下列枚举:
typedef enum
{
VIEW_STILL = 0x0000, ///< Overlay effect with new view transplate in
VIEW_TRANSPLATION = 0x0001, ///< Transplate from the slide direction
VIEW_REDUCTION = 0x0002, ///< Zoom in from the slide direction
VIEW_ROTATE = 0x0003, ///< Rotate in from the slide direction
VIEW_CUBE = 0x0004, ///< Rotate in from the slide direction like cube
VIEW_ANIMATION_NULL = 0x0005,
VIEW_ANIMATION_1, ///< Recommended for startup
VIEW_ANIMATION_2, ///< Recommended for startup
VIEW_ANIMATION_3, ///< Recommended for startup
VIEW_ANIMATION_4, ///< Recommended for startup
VIEW_ANIMATION_5, ///< Recommended for startup
VIEW_ANIMATION_6, ///< Recommended for shutdown
VIEW_ANIMATION_7, ///< Recommended for shutdown
VIEW_ANIMATION_8, ///< Recommended for shutdown
} VIEW_SWITCH_STYLE;
立即切换视图
使用 gui_view_switch_direct()
立即切换视图,可以配合视图控件中子控件的事件或动画使用,注意切换风格仅限于动画风格,不可设置滑动风格。
获取当前显示的视图控件指针
使用 gui_view_get_current_view()
函数可以获取当前显示的视图控件指针,可以搭配 gui_view_switch_direct()
使用,将当前view切换。
示例
视图控件
以下是三个单独的C文件,每个C文件中包含view控件的描述子以及design函数。
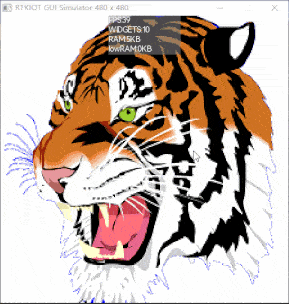
API
Defines
-
EVENT_NUM_MAX
Enums
-
enum VIEW_SWITCH_STYLE
Values:
-
enumerator VIEW_STILL
Overlay effect with new view transplate in.
-
enumerator VIEW_TRANSPLATION
Transplate from the slide direction.
-
enumerator VIEW_REDUCTION
Zoom in from the slide direction.
-
enumerator VIEW_ROTATE
Rotate in from the slide direction.
-
enumerator VIEW_CUBE
Rotate in from the slide direction like cube.
-
enumerator VIEW_ANIMATION_NULL
-
enumerator VIEW_ANIMATION_1
Recommended for startup.
-
enumerator VIEW_ANIMATION_2
Recommended for startup.
-
enumerator VIEW_ANIMATION_3
Recommended for startup.
-
enumerator VIEW_ANIMATION_4
Recommended for startup.
-
enumerator VIEW_ANIMATION_5
Recommended for startup.
-
enumerator VIEW_ANIMATION_6
Recommended for shutdown.
-
enumerator VIEW_ANIMATION_7
Recommended for shutdown.
-
enumerator VIEW_ANIMATION_8
Recommended for shutdown.
-
enumerator VIEW_STILL
Functions
-
gui_view_t *gui_view_create(void *parent, const gui_view_descriptor_t *descriptor, int16_t x, int16_t y, int16_t w, int16_t h)
Create a view widget.
- 参数:
parent – The father widget it nested in.
descriptor – Pointer to a descriptor that defines the new view to switch to.
x – The X-axis coordinate relative to parent widget
y – The Y-axis coordinate relative to parent widget
w – Width
h – Height
- 返回:
return the widget object pointer.
-
void gui_view_descriptor_register(const gui_view_descriptor_t *descriptor)
Register view’s descriptor.
- 参数:
descriptor – Pointer to a descriptor that defines the new view to switch to.
-
const gui_view_descriptor_t *gui_view_descriptor_get(const char *name)
Get target view’s descriptor by name.
- 参数:
name – View descriptor’s name that can used to find target view.
-
void gui_view_switch_on_event(gui_view_t *_this, const gui_view_descriptor_t *descriptor, VIEW_SWITCH_STYLE switch_out_style, VIEW_SWITCH_STYLE switch_in_style, gui_event_t event)
Switches the current GUI view to a new view based on the specified event.
This function handles the transition between GUI views. It takes the current view context and switches it to a new view as described by the
descriptor
. The transition is triggered by a specified event and can be customized with different switch styles for the outgoing and incoming views.- 参数:
_this – Pointer to the current GUI view context that is being manipulated.
descriptor – Pointer to a descriptor that defines the new view to switch to.
switch_out_style – Style applied to the outgoing view during the switch.
switch_in_style – Style applied to the incoming view during the switch.
event – The event that triggers the view switch.
-
void gui_view_switch_direct(gui_view_t *_this, const gui_view_descriptor_t *descriptor, VIEW_SWITCH_STYLE switch_out_style, VIEW_SWITCH_STYLE switch_in_style)
Switches directly the current GUI view to a new view through animation.
This function handles the transition between GUI views. It takes the current view context and switches it to a new view as described by the
descriptor
. The transition animation can be customized with different animation switch styles for the outgoing and incoming views.- 参数:
_this – Pointer to the current GUI view context that is being manipulated.
descriptor – Pointer to a descriptor that defines the new view to switch to.
switch_out_style – Style applied to the outgoing view during the switch.
switch_in_style – Style applied to the incoming view during the switch.
-
gui_view_t *gui_view_get_current_view(void)
Get current view pointer.
- 返回:
return current view pointer.
-
struct gui_view_id_t
-
struct gui_view_t
Public Members
-
gui_obj_t base
-
int16_t release_x
-
int16_t release_y
-
gui_animate_t *animate
-
gui_view_id_t cur_id
-
VIEW_SWITCH_STYLE style
-
const struct gui_view_descriptor *descriptor
-
uint32_t view_switch_ready
-
uint32_t event
-
uint32_t moveback
-
uint32_t view_tp
-
uint32_t view_left
-
uint32_t view_right
-
uint32_t view_up
-
uint32_t view_down
-
uint32_t view_click
-
uint32_t view_touch_long
-
uint32_t view_button
-
uint32_t view_button_long
-
struct gui_view_on_event **on_event
-
uint8_t on_event_num
-
uint8_t checksum
-
gui_obj_t base
-
struct gui_view_descriptor_t
Public Members
-
const char *name
-
gui_view_t **pView
-
void (*on_switch_in)(gui_view_t *view)
-
void (*on_switch_out)(gui_view_t *view)
-
uint8_t keep
-
const char *name
-
struct gui_view_on_event_t
Public Members
-
const gui_view_descriptor_t *descriptor
-
VIEW_SWITCH_STYLE switch_out_style
-
VIEW_SWITCH_STYLE switch_in_style
-
gui_event_t event
-
const gui_view_descriptor_t *descriptor