Image Layout
This section primarily introduces Flash Layout and Image Format.
Flash Layout
The Flash Layout is as follows, consisting of Reserved, OEM Header (storage space for Config File), Bank0 Boot Patch, Bank1 Boot Patch, OTA Bank0, OTA Bank1, Secure APP, OTA TMP, FTL, and APP Defined Section. The starting address for accessing Flash is 0x04000000.
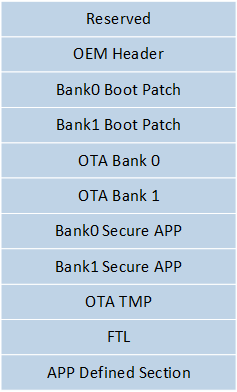
Flash Layout
OTA Bank layout and description for each part is shown below.
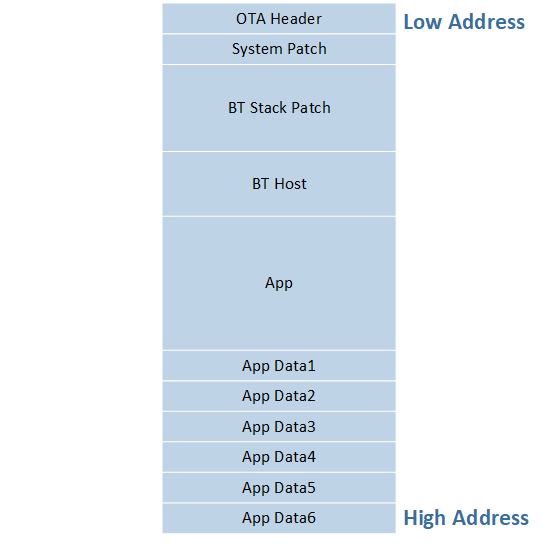
Layout of OTA Bank
Memory Segment |
Starting Address |
Size (Bytes) |
Functions |
Support DFU or not |
---|---|---|---|---|
OTA Header |
Determined in the OEM Config region |
4KB |
This region contains the OTA Header version and start address and size of the images in the bank |
Yes, when using supporting Bank switching scheme No, when using non-supporting Bank switching scheme |
System Patch |
Determined in the OTA Header region |
Variable |
This region contains the code that optimizes and extends the system in non-secure ROM |
Yes |
BT Stack Patch |
Determined in the OTA Header region |
Variable |
Bluetooth controller protocol stack optimization and extension code |
Yes |
BT Host |
Determined in the OTA Header region |
Variable |
Implements part of the code for HCI and above Bluetooth protocol stack |
Yes |
APP |
Determined in the OTA Header region |
Variable |
This region contains project code |
Yes |
APP Config File |
Determined in the OTA Header region |
Variable |
APP Config information |
Yes |
APP Data1 |
Determined in the OTA Header region |
Variable |
Application’s customized area |
Yes |
APP Data2 |
Determined in the OTA Header region |
Variable |
Application’s customized area |
Yes |
APP Data3 |
Determined in the OTA Header region |
Variable |
Application’s customized area |
Yes |
APP Data4 |
Determined in the OTA Header region |
Variable |
Application’s customized area |
Yes |
APP Data5 |
Determined in the OTA Header region |
Variable |
Application’s customized area |
Yes |
APP Data6 |
Determined in the OTA Header region |
Variable |
Application’s customized area |
Yes |
Flash Layout Adjustment
Flash layout settings are divided into two levels:
High Level Flash map —— determined by the OEM Header
OTA Bank map —— determined by the OTA Header file
Users can use the Config Set option in MP Tool to load a customized Flash layout file and generate a Config File.
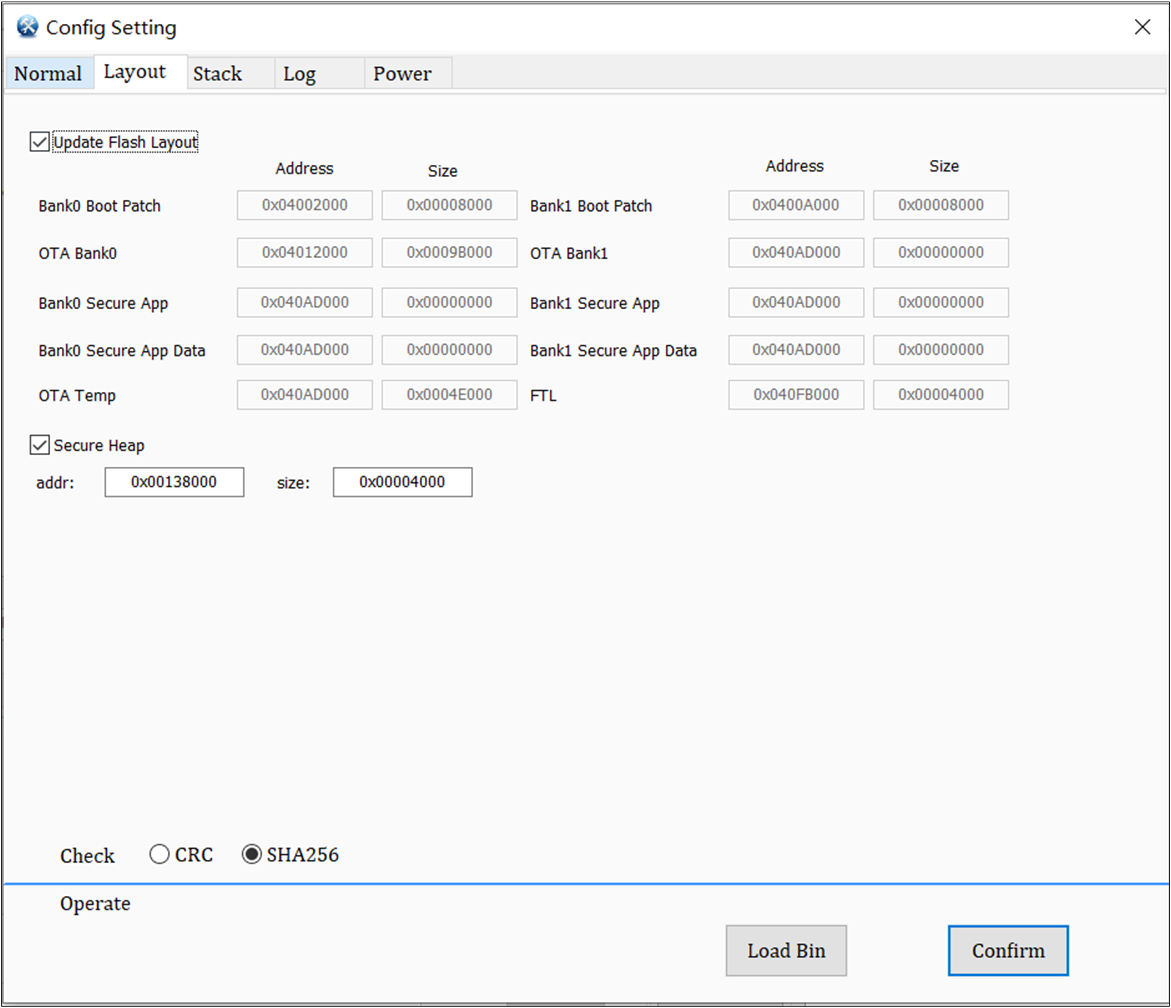
Generate Config File through MP Tool
For the convenience of users, Realtek provides Flash Map Generate Tool for Flash layout files. The explanation is as follows:
flash map.ini
file —— can be imported into MP Tool to generate Config File and OTA header.flash_map.h
file —— needs to be copied to the APP project directory to compile a correct and runnable APP image.
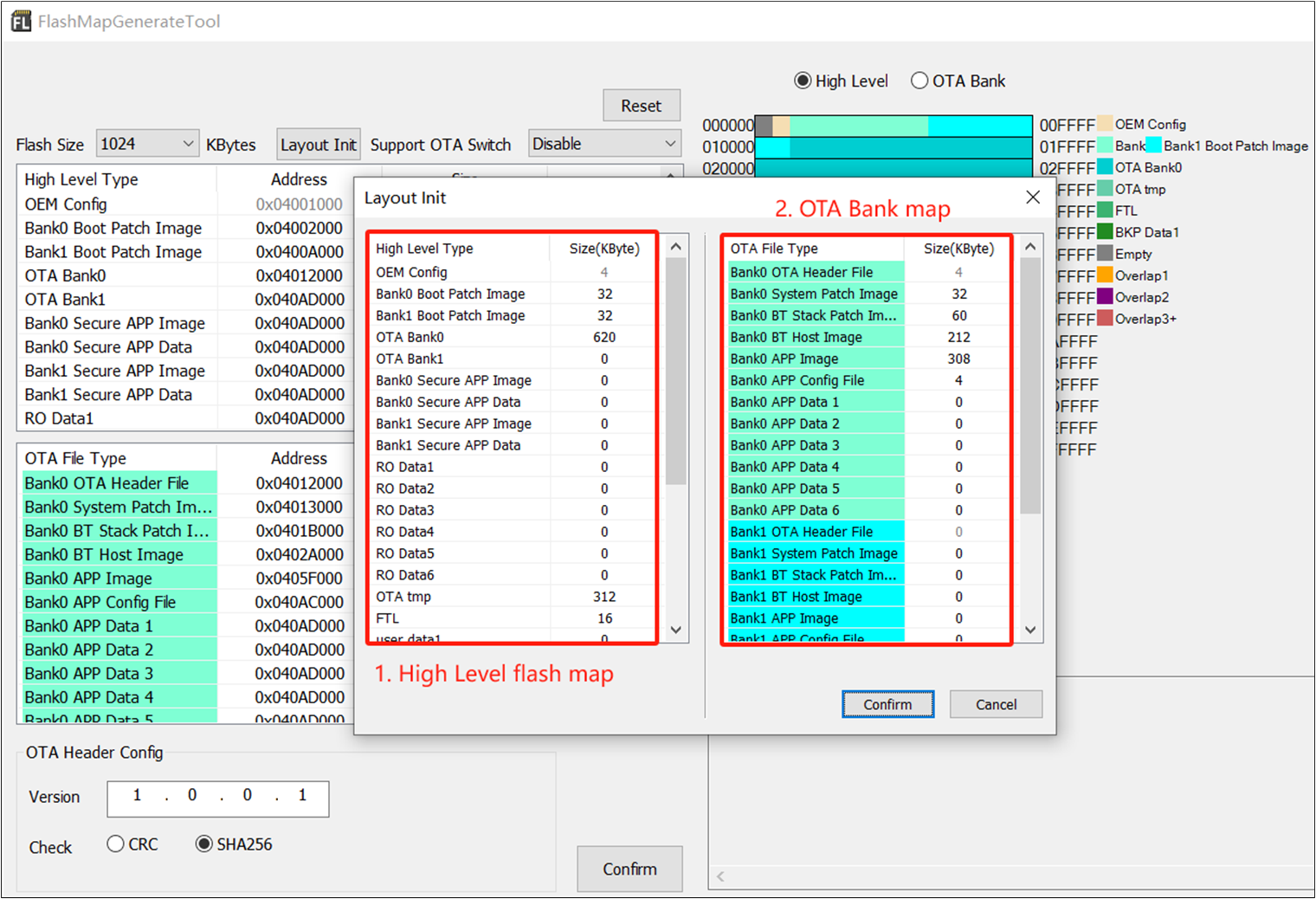
Flash Map Generate Tool configures Flash Map
But at the same time, the following principles must be followed to adjust the flash layout.
If choosing the supporting Bank switching scheme, ensure that the size of OTA Bank0 is equal to the size of OTA Bank1, and set the size of the OTA TMP area to 0.
If choosing the non-supporting Bank switching scheme, set the size of OTA Bank1 to 0, and the size of the OTA TMP area should not be smaller than the size of the largest image in OTA Bank0.
In order to lock all the code areas to prevent unexpected flash write and erase operations, align the offset of the end address of OTA Bank1 relative to the start address of the flash to the actually selected flash address as much as possible. Please refer to Software Block Protect.
Because the data stored in the APP Data1, APP Data2, APP Data3, APP Data4, APP Data5, or APP Data6 area in the OTA Bank may fall within the locked range of the flash, this area is generally stored as read-only data. If it is necessary to store data that needs to be rewritten, it should be placed in the “APP Defined Section” area.
Flash Layout Example
Based on the Flash Layout Adjustment , this section provides examples of the flash layout under Supporting Bank Switching Scheme and Non-supporting Bank Switching Scheme .
Supporting Bank Switching Scheme
Sample Layout for flash (total size = 2MB) |
Size(Bytes) |
Start Address |
---|---|---|
Reserved |
4K |
0x04000000 |
OEM Header |
4K |
0x04001000 |
Bank0 Boot Patch |
32K |
0x04002000 |
Bank1 Boot Patch |
32K |
0x0400A000 |
OTA Bank0 |
596K |
0x04012000 |
|
4K |
0x04012000 |
|
32K |
0x04013000 |
|
60K |
0x0401B000 |
|
212K |
0x0402A000 |
|
284K |
0x0405F000 |
|
4K |
0x040A6000 |
|
0K |
0x040A7000 |
|
0K |
0x040A7000 |
|
0K |
0x040A7000 |
|
0K |
0x040A7000 |
|
0K |
0x040A7000 |
|
0K |
0x040A7000 |
OTA Bank1 |
596K |
0x040A7000 |
|
4K |
0x040A7000 |
|
32K |
0x040A8000 |
|
60K |
0x040B0000 |
|
212K |
0x040BF000 |
|
284K |
0x040F4000 |
|
4K |
0x0413B000 |
|
0K |
0x0413C000 |
|
0K |
0x0413C000 |
|
0K |
0x0413C000 |
|
0K |
0x0413C000 |
|
0K |
0x0413C000 |
|
0K |
0x0413C000 |
Bank0 Secure APP code |
0K |
0x0413C000 |
Bank0 Secure APP Data |
0K |
0x0413C000 |
Bank1 Secure APP code |
0K |
0x0413C000 |
Bank1 Secure APP Data |
0K |
0x0413C000 |
OTA Temp |
32K |
0x0413C000 |
FTL |
16K |
0x04148000 |
APP Defined Section |
736K |
0x040FF000 |
Note
Flash Layout should be determined based on the actual size of the image and data.
Non-supporting Bank Switching Scheme
Sample Layout for flash (total size = 1MB) |
Size (Bytes) |
Start Address |
---|---|---|
Reserved |
4K |
0x04000000 |
OEM Header |
4K |
0x04001000 |
Bank0 Boot Patch |
32K |
0x04002000 |
Bank1 Boot Patch |
32K |
0x0400A000 |
OTA Bank0 |
612K |
0x04012000 |
|
4K |
0x04012000 |
|
32K |
0x04013000 |
|
60K |
0x0401B000 |
|
212K |
0x0402A000 |
|
304K |
0x0405F000 |
|
4K |
0x040AC000 |
|
0K |
0x040AD000 |
|
0K |
0x040AD000 |
|
0K |
0x040AD000 |
|
0K |
0x040AD000 |
|
0K |
0x040AD000 |
|
0K |
0x040AD000 |
OTA Bank1 (Equal to 0) |
0K |
0x040AD000 |
Bank0 Secure APP code |
0K |
0x040AD000 |
Bank0 Secure APP Data |
0K |
0x040AD000 |
Bank1 Secure APP code |
0K |
0x040AD000 |
Bank1 Secure APP Data |
0K |
0x040AD000 |
OTA Temp |
312K |
0x040AD000 |
FTL |
16K |
0x040FB000 |
Note
The space for APP data is not allocated in this sample. Flash Layout should be distributed based on the actual size of image and data.
Image Format
All images that support upgrades in OTA Bank Flash Segmentation are composed of a 1280-byte image header and a variable-length payload.
The image header contains the image type, size, version number, hash value, signature information, etc. The definitions of each field in the image header are as follows:
Memory Segment |
Size(Bytes) |
Functions |
---|---|---|
image_signature |
384 |
Signature of the image, used for authentication when secure boot is enabled |
image_hash |
32 |
Hash check for the image, used for verifying the integrity of the image |
image_control_header |
12 |
Control information of the image, for details please refer to image_control_header format |
uuid |
16 |
UUID value of the image |
exe_base |
4 |
Execution address of the image |
load_src |
4 |
Starting address of the code loaded into RAM |
load_len |
4 |
Size of the code loaded into RAM |
image_base |
4 |
Flash address of the image |
dev_id |
2 |
Not used |
flash_layout_size_4k |
2 |
Size of the flash layout (unit: 4KB) |
magic_pattern |
4 |
Pattern information of the image |
dec_key |
16 |
Not used |
load_dst |
4 |
Starting address of the code loaded into Flash |
ex_info |
24 |
Information of the heap and the RAM |
git_ver |
16 |
Version information of the image |
RSA Public Key |
388 |
Used for authentication when secure boot is enabled |
flash_sec_cfg |
20 |
Flash security configuration information |
image_info |
344 |
For the OTA Header, image_info represents the starting address and size of each image in the OTA Bank. For other images, image_info is reserved. |
image_control_header format in image header is shown as follows:
typedef struct _IMG_CTRL_HEADER_FORMAT
{
uint16_t crc16;
uint8_t ic_type;
uint8_t secure_version;
union
{
uint16_t value;
struct
{
uint16_t xip: 1; // payload is executed on flash
uint16_t enc: 1; // all the payload is encrypted
uint16_t load_when_boot: 1; // load image when boot
uint16_t enc_load: 1; // encrypt load part or not
uint16_t enc_key_select: 3; // referenced to ENC_KEY_SELECT
uint16_t not_ready: 1; //for copy image in ota
uint16_t not_obsolete: 1; //for copy image in ota
uint16_t integrity_check_en_in_boot: 1; // enable image integrity check in boot flow
uint16_t compressed_not_ready: 1;
uint16_t compressed_not_obsolete: 1;
uint16_t rsvd: 1;
uint16_t image_type: 3; /*for app 000b: normal image, 001b:compressed image, other for more types
for patch in temp bank consist of 001b: patch+fsbl, 010b: patch+app, 011b: patch+fsbl+app*/
};
} ctrl_flag;
uint16_t image_id;
uint32_t payload_len;
} T_IMG_CTRL_HEADER_FORMAT;
Parameter description:
- ic_type:
indicates IC type, the value for RTL87x2G IC type is 15.
- secure_version:
indicates the version of the boot security check image.
- image_id:
identifies different types of image, among which SCCD, OCCD cannot be updated through DFU.
typedef enum { IMG_SCCD = 0x379D, IMG_OCCD = 0x379E, IMG_BOOTPATCH = 0x379F, /*!< KM4 boot patch */ IMG_DFU_FIRST = IMG_BOOTPATCH, IMG_OTA = 0x37A0, /*!< OTA header */ IMG_SECUREMCUAPP = 0x37A2, /*!< KM4 secure app */ IMG_SECUREMCUAPPDATA = 0x37A3, /*!< KM4 secure app data */ IMG_BT_STACKPATCH = 0x37A6, /*!< BT stack patch */ IMG_BANK_FIRST = IMG_BT_STACKPATCH, IMG_MCUPATCH = 0x37A7, /*!< KM4 non-secure rom patch */ IMG_UPPERSTACK = 0x37A8, /*!< KM4 non-secure Upperstack */ IMG_MCUAPP = 0x37A9, /*!< KM4 non-secure app */ IMG_MCUCFGDATA = 0x37AA, /*!< KM4 MCUConfigData */ IMG_MCUAPPDATA1 = 0x37AE, IMG_MCUAPPDATA2 = 0x37AF, IMG_MCUAPPDATA3 = 0x37B0, IMG_MCUAPPDATA4 = 0x37B1, IMG_MCUAPPDATA5 = 0x37B2, IMG_MCUAPPDATA6 = 0x37B3, IMG_ZIGBEESTACK = 0x37B4, /*!< KM4 Zigbee stack */ IMG_MAX = 0x37B5, IMG_DFU_MAX = IMG_MAX, IMG_RO_DATA1 = 0x3A81, IMG_RO_DATA2 = 0x3A82, IMG_RO_DATA3 = 0x3A83, IMG_RO_DATA4 = 0x3A84, IMG_RO_DATA5 = 0x3A85, IMG_RO_DATA6 = 0x3A86, IMG_USER_DATA8 = 0xFFF7, /*!< the image data only support unsafe single bank ota */ IMG_USER_DATA_FIRST = IMG_USER_DATA8, IMG_USER_DATA7 = 0xFFF8, /*!< the image data only support unsafe single bank ota */ IMG_USER_DATA6 = 0xFFF9, /*!< the image data only support unsafe single bank ota */ IMG_USER_DATA5 = 0xFFFA, /*!< the image data only support unsafe single bank ota */ IMG_USER_DATA4 = 0xFFFB, /*!< the image data only support unsafe single bank ota */ IMG_USER_DATA3 = 0xFFFC, /*!< the image data only support unsafe single bank ota */ IMG_USER_DATA2 = 0xFFFD, /*!< the image data only support unsafe single bank ota */ IMG_USER_DATA1 = 0xFFFE, /*!< the image data only support unsafe single bank ota */ IMG_USER_DATA_MAX = 0xFFFF, /*!< the image data only support unsafe single bank ota */ } IMG_ID;
Parameter description:
- payload_len:
indicates the size of the image, not including the 1280 bytes of the image header. The total length of the image is the payload_len plus 1280 bytes.
- crc16:
indicates the crc16 checksum of the image. By default, the image uses SHA256 for verification, and the checksum is stored in image_hash. The crc16 can be ignored.
- ctrl_flag:
the only bit fields related to DFU are not_ready and not_obsolete.
not_ready: indicates whether the image is valid. By default, the not_ready field in the image header of files published, compiled, or generated by tools is all 0.
There is a risk of power outage when transferring the image to the backup area via Bluetooth. Therefore, before writing to the backup area, it is necessary to change not_ready to 1 and then write to the flash.
Only when the image transfer is complete and the image integrity check is successful, modify the not_ready in the backup area image to 0, indicating the backup area image is valid.
After the system restarts, the activation process of the backup image is completed by the BootLoader.
not_obsolete: indicates if the image should be abandoned and its default value is 1.
When bank switching is supported, not_obsolete is invalid during the DFU process.
When bank switching is not supported, after the DFU upgrade is complete, the boot code determines that not_ready is 0 and not_obsolete is 1. At this time, the image will be transferred from the OTA TMP area to the corresponding image run area in OTA Bank0. After the transfer is successful, write the not_obsolete in the OTA TMP area image to 0 to prevent repeated transfers. This ensures that the upgraded image is properly installed and run, and the old image is marked as obsolete to avoid being used again.