对象控件
对象控件实现了屏幕上控件的基本属性。屏幕控件是控件树的根节点。极坐标的原点在 Y 轴的负方向,极坐标的正方向为顺时针方向,屏幕坐标系设置如下所示。
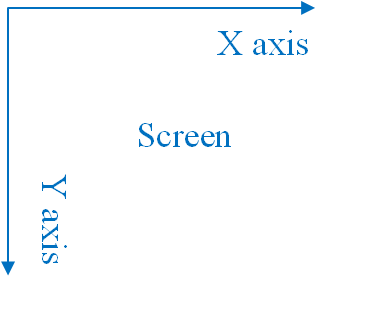
用法
API
Enums
-
enum T_OBJ_TYPE
Values:
-
enumerator SCREEN
-
enumerator WINDOW
-
enumerator TABVIEW
-
enumerator TAB
-
enumerator CURTAINVIEW
-
enumerator CURTAIN
-
enumerator IMAGE
-
enumerator BUTTON
-
enumerator ICONLIST
-
enumerator ICON
-
enumerator IMAGE_FROM_MEM
-
enumerator TEXTBOX
-
enumerator SCROLLTEXTBOX
-
enumerator SEEKBAR
-
enumerator PROGRESSBAR
-
enumerator CLICKSWITCH
-
enumerator PAGE
-
enumerator SCROLL_WHEEL
-
enumerator PAGEBAR
-
enumerator RETURNWIDGET
-
enumerator RECTANGLE
-
enumerator WINDOWWITHBORDER
-
enumerator CANVAS
-
enumerator VG_LITE_CLOCK
-
enumerator VG_LITE_CUBE
-
enumerator GRID
-
enumerator RADIO
-
enumerator RADIOSWITCH
-
enumerator ARC
-
enumerator JAVASCRIPT
-
enumerator MOVIE
-
enumerator IMAGE_SCOPE
-
enumerator CARDVIEW
-
enumerator CARD
-
enumerator PAGELIST
-
enumerator PAGELISTVIEW
-
enumerator MACRO_ANIMATETRANSFORM
-
enumerator HONEYCOMB_LIST
-
enumerator WHEEL_LIST
-
enumerator QRCODE
-
enumerator GALLERY
-
enumerator TURN_TABLE
-
enumerator KEYBOARD
-
enumerator MACRO_MOTORIZED_CURTAIN
-
enumerator MULTI_LEVEL
-
enumerator U8G2
-
enumerator MACRO_ONCLICK
-
enumerator MACRO_BACKICON
-
enumerator CANVAS_IMG
-
enumerator SCREEN
Functions
-
void gui_obj_ctor(gui_obj_t *this_widget, gui_obj_t *parent, const char *name, int16_t x, int16_t y, int16_t w, int16_t h)
- 参数:
this_widget –
parent – switching events
filename – how to trigger events
x – left
y – top
w – width
h – height
- 返回:
void Example usage
static void app_main_task(void *parent, const char *name, int16_t x, int16_t y, int16_t w, int16_t h) { gui_obj_t *base = (gui_obj_t *)this_widget; gui_obj_ctor(base, parent, name, x, y, w, h); }
-
gui_obj_t *gui_obj_create(void *parent, const char *name, int16_t x, int16_t y, int16_t w, int16_t h)
- 参数:
parent –
filename –
x –
y –
w –
h –
- 返回:
gui_obj_t*
-
void gui_obj_add_event_cb(void *obj, gui_event_cb_t event_cb, gui_event_t filter, void *user_data)
create event widget
- 参数:
obj –
event_cb – switching events
filter – how to trigger events
user_data –
- 返回:
void Example usage
static void app_main_task(void *parent) { gui_win_t *clock = gui_win_create(parent, "clock", 0, 84, 320, 300); gui_obj_add_event_cb(clock, (gui_event_cb_t)show_clock, GUI_EVENT_TOUCH_CLICKED, NULL); }
-
void gui_obj_event_set(gui_obj_t *obj, gui_event_t event_code)
this_widget API only for Widget, not for Application
- 参数:
obj –
event_code –
- 返回:
void Example usage
static void app_main_task(gui_obj_t *obj) { gui_obj_event_set(obj, GUI_EVENT_TOUCH_CLICKED); }
-
void gui_obj_tree_free(void *obj)
free the widget tree recursively,from the root to the leaves.Preorder traversal.
- 参数:
obj – the root of the widget tree.
- 返回:
void Example usage
static void app_main_task(gui_app_t *app) { gui_obj_tree_free(&app->screen); }
-
void gui_obj_tree_print(gui_obj_t *obj)
print the widget tree recursively,from the root to the leaves.Preorder traversal.
- 参数:
obj – the root of the widget tree.
- 返回:
void Example usage
static void app_main_task(gui_app_t *app) { gui_obj_tree_print(&app->screen); }
-
void gui_obj_tree_count_by_type(gui_obj_t *obj, T_OBJ_TYPE type, int *count)
get count of one type on tree
- 参数:
obj – the root of the widget tree.
type – widget type.
count – count result.
- 返回:
void
-
void gui_obj_tree_show(gui_obj_t *obj, bool enable)
show or hide the widget
- 参数:
obj – the root of the widget tree.
enable – true for show, false for hide.
- 返回:
void
-
void gui_obj_show(void *obj, bool enable)
object show or not
- 参数:
obj –
enable –
- 返回:
void
Example usage
static void app_main_task(gui_app_t *app) { gui_img_t *hour; gui_obj_show(hour,false); gui_obj_show(hour,true); }
-
gui_obj_t *gui_obj_tree_get_root(gui_obj_t *obj)
show the root of this_widget tree
- 参数:
obj – the root of the widget tree.
- 返回:
gui_obj_t*
-
gui_obj_t *gui_obj_get_child_handle(gui_obj_t *obj, T_OBJ_TYPE child_type)
get child type
- 参数:
obj – the root of the widget tree.
child_type –
- 返回:
gui_obj_t*
-
bool gui_obj_in_rect(gui_obj_t *obj, int16_t x, int16_t y, int16_t w, int16_t h)
judge the obj if in range of this_widget rect
- 参数:
obj –
x –
y –
w –
h –
- 返回:
true
- 返回:
false
-
void gui_obj_skip_all_parent_left_hold(gui_obj_t *obj)
skip all left slide hold actions of the parent object
- 参数:
obj – the root of the widget tree.
-
void gui_obj_skip_all_child_left_hold(gui_obj_t *obj)
skip all left slide hold actions of the child object
- 参数:
obj – the root of the widget tree.
-
void gui_obj_skip_other_left_hold(gui_obj_t *obj)
skip all left slide hold actions of the other object
- 参数:
obj – the root of the widget tree.
-
void gui_obj_skip_all_parent_right_hold(gui_obj_t *obj)
skip all right slide hold actions of the parent object
- 参数:
obj – the root of the widget tree.
-
void gui_obj_skip_all_child_right_hold(gui_obj_t *obj)
skip all right slide hold actions of the child object
- 参数:
obj – the root of the widget tree.
-
void gui_obj_skip_other_right_hold(gui_obj_t *obj)
skip all right slide hold actions of the other object
- 参数:
obj –
-
void gui_obj_skip_all_parent_down_hold(gui_obj_t *obj)
skip all down slide hold actions of the parent object
- 参数:
obj – the root of the widget tree.
-
void gui_obj_skip_all_child_down_hold(gui_obj_t *obj)
skip all down slide hold actions of the child object
- 参数:
obj – the root of the widget tree.
-
void gui_obj_skip_other_down_hold(gui_obj_t *obj)
skip all down slide hold actions of the other object
- 参数:
obj – the root of the widget tree.
-
void gui_obj_skip_all_parent_up_hold(gui_obj_t *obj)
skip all up slide hold actions of the parent object
- 参数:
obj – the root of the widget tree.
-
void gui_obj_skip_all_child_up_hold(gui_obj_t *obj)
skip all up slide hold actions of the child object
- 参数:
obj – the root of the widget tree.
-
void gui_obj_skip_other_up_hold(gui_obj_t *obj)
skip all up slide hold actions of the other object
- 参数:
obj – the root of the widget tree.
-
void gui_obj_skip_all_child_short(gui_obj_t *obj)
skip all short click actions of the child object
- 参数:
obj – the root of the widget tree.
-
void gui_obj_skip_other_short(gui_obj_t *obj)
skip all short click actions of the other object
- 参数:
obj – the root of the widget tree.
-
void gui_obj_get_area(gui_obj_t *obj, int16_t *x, int16_t *y, int16_t *w, int16_t *h)
get the area of this_widget obj
- 参数:
obj –
x –
y –
w –
h –
-
bool gui_obj_point_in_obj_rect(gui_obj_t *obj, int16_t x, int16_t y)
judge the point if in range of this_widget obj rect
- 参数:
obj –
x –
y –
- 返回:
true
- 返回:
false
-
uint8_t gui_obj_checksum(uint8_t seed, uint8_t *data, uint8_t len)
- 参数:
seed –
data –
len –
- 返回:
uint8_t
-
void gui_obj_tree_get_widget_by_name(gui_obj_t *obj, const char *name, gui_obj_t **output)
get widget in tree by name
- 参数:
obj – tree
name – widget name
output – widget
- 返回:
uint8_t
-
void gui_obj_tree_get_widget_by_type(gui_obj_t *root, T_OBJ_TYPE type, gui_obj_t **output)
get widget in tree by type
- 参数:
obj – tree
type – widget type
output – widget
- 返回:
uint8_t
-
void animate_frame_update(gui_animate_t *animate, gui_obj_t *obj)
update animate on every frame
- 参数:
animate –
obj – widget
-
gui_animate_t *gui_obj_set_animate(gui_animate_t *animate, uint32_t dur, int repeat_count, void *callback, void *p)
set animate
- 参数:
animate – pointer
dur – animation time cost in ms
repeat_count – rounds to repeat
callback – every frame callback
p – callback’s parameter
-
struct gui_obj_t
- #include <gui_obj.h>
Public Members
-
const char *name
-
struct _gui_obj_t *parent
-
int16_t x
-
int16_t y
-
int16_t w
-
int16_t h
-
gui_list_t child_list
-
gui_list_t brother_list
-
void (*obj_cb)(struct _gui_obj_t *obj, T_OBJ_CB_TYPE cb_type)
-
T_OBJ_TYPE type
-
uint32_t active
-
uint32_t not_show
-
uint32_t skip_tp_left_hold
-
uint32_t skip_tp_right_hold
-
uint32_t skip_tp_up_hold
-
uint32_t skip_tp_down_hold
-
uint32_t skip_tp_short
-
uint32_t create_done
-
uint32_t flag_3d
-
uint32_t gesture
-
uint32_t event_dsc_cnt
-
uint32_t opacity_value
-
uint32_t has_input_prepare_cb
-
uint32_t has_prepare_cb
-
uint32_t has_draw_cb
-
uint32_t has_end_cb
-
uint32_t has_destroy_cb
-
gui_event_dsc_t *event_dsc
-
gui_matrix_t *matrix
-
const char *name