透视
透视控件是一个六面体形状的控件,由六个标签页作为六个面。当创建好透视控件后,该控件会自动旋转来显示不同的标签页,在旋转的同时也可以点击不同的标签页实现快速跳转到当前标签页。
用法
创建透视控件
使用 gui_perspective_t *gui_perspective_create(void *parent, const char *name, gui_perspective_imgfile_t *img_file, int16_t x, int16_t y) 函数创建透视控件。img_file
是一个包含六个标签页的图像资源的结构体。创建透视控件时,六个标签页的图片资源可以使用内存地址或文件路径,gui_perspective_imgfile_t
(参考如下结构体) 中的字段 src_mode 表明了图片资源是来自内存地址还是文件路径,如示例中展示,src_mode
为 IMG_SRC_MEMADDR
时表示图片资源来自内存地址。
typedef struct
{
IMG_SOURCE_MODE_TYPE src_mode[6]; //!< flag: indicate file src
union
{
char *img_path[6]; //!< images file path
void *data_addr[6]; //!< images memory address
};
} gui_perspective_imgfile_t;
设置透视控件的图片
使用 void gui_perspective_set_img(gui_perspective_t *perspective, gui_perspective_imgfile_t *img_file) 函数设置透视控件的图片。
设置图片混合模式
默认情况下,透视控件的图像混合模式为IMG_SRC_OVER_MODE
,调用 void gui_perspective_set_mode(gui_perspective_t *perspective, uint8_t img_index, BLEND_MODE_TYPE mode) 函数可以设置图像的混合模式。混合模式BLEND_MODE_TYPE
枚举类型如下:
typedef enum
{
IMG_BYPASS_MODE = 0,
IMG_FILTER_BLACK,
IMG_SRC_OVER_MODE, //S * Sa + (1 - Sa) * D
IMG_COVER_MODE,
IMG_RECT,
} BLEND_MODE_TYPE;
示例
#include "root_image_hongkong/ui_resource.h"
#include "gui_perspective.h"
#include "gui_canvas.h"
#include "gui_win.h"
#include "gui_obj.h"
#include "gui_app.h"
#include <gui_tabview.h>
#include "app_hongkong.h"
extern gui_tabview_t *tv;
gui_perspective_t *img_test;
static void canvas_cb_black(gui_canvas_t *canvas)
{
nvgRect(canvas->vg, 0, 0, 368, 448);
nvgFillColor(canvas->vg, nvgRGBA(0, 0, 128, 255));
nvgFill(canvas->vg);
}
static void callback_prism_touch_clicked()
{
gui_app_t *app = get_app_hongkong();
gui_obj_t *screen = &(app->screen);
int angle = img_test->release_x;
if (img_test->release_x < 0)
{
angle = img_test->release_x + 360;
}
angle = (angle % 360) / (360 / 6);
if (angle < 0 || angle > 5)
{
angle = 0;
}
gui_obj_tree_free(screen);
app->ui_design(get_app_hongkong());
gui_tabview_jump_tab(tv, angle, 0);
}
void callback_prism(void *obj, gui_event_t e)
{
gui_app_t *app = get_app_hongkong();
gui_obj_t *screen = &(app->screen);
gui_obj_tree_free(screen);
gui_win_t *win = gui_win_create(screen, "win", 0, 0, 368, 448);
gui_canvas_t *canvas = gui_canvas_create(win, "canvas", 0, 0, 0, 368, 448);
gui_canvas_set_canvas_cb(canvas, canvas_cb_black);
gui_perspective_imgfile_t imgfile =
{
.src_mode[0] = IMG_SRC_MEMADDR, .src_mode[1] = IMG_SRC_MEMADDR, .src_mode[2] = IMG_SRC_MEMADDR,
.src_mode[3] = IMG_SRC_MEMADDR, .src_mode[4] = IMG_SRC_MEMADDR, .src_mode[5] = IMG_SRC_MEMADDR,
.data_addr[0] = CLOCKN_BIN,
.data_addr[1] = WEATHER_BIN,
.data_addr[2] = MUSIC_BIN,
.data_addr[3] = QUICKCARD_BIN,
.data_addr[4] = HEARTRATE_BIN,
.data_addr[5] = ACTIVITY_BIN
};
img_test = gui_perspective_create(canvas, "test", &imgfile, 0, 0);
gui_obj_add_event_cb(win, (gui_event_cb_t)callback_prism_touch_clicked, GUI_EVENT_TOUCH_CLICKED,
NULL);
}
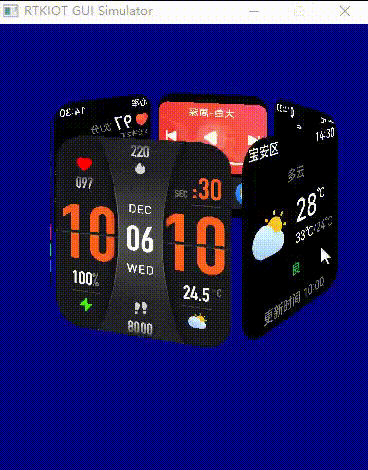
API
Defines
-
RAD(d)
angle to rad
Functions
-
void gui_perspective_set_mode(gui_perspective_t *perspective, uint8_t img_index, BLEND_MODE_TYPE mode)
set the perspective image’s blend mode
- 参数:
perspective – the perspective widget pointer
img_index – the perspective image’s index
mode – the enumeration value of the mode is BLEND_MODE_TYPE
-
void gui_perspective_set_img(gui_perspective_t *perspective, gui_perspective_imgfile_t *img_file)
set perspective image
- 参数:
perspective – the perspective widget pointer
img_file – the image file data, set flg_fs true when using filesystem
-
gui_perspective_t *gui_perspective_create(void *parent, const char *name, gui_perspective_imgfile_t *img_file, int16_t x, int16_t y)
create 3D perspective, images can be loaded from filesystem or memory address
Example usage
void perspctive_example(void *parent) { gui_perspective_imgfile_t imgfile = { .flg_fs = true, .img_path[0] = "Clockn.bin", .img_path[1] = "Weather.bin", .img_path[2] = "Music.bin", .img_path[3] = "QuickCard.bin", .img_path[4] = "HeartRate.bin", .img_path[5] = "Activity.bin" }; img_test = gui_perspective_create(canvas, "test", &imgfile, 0, 0); }
- 参数:
parent – parent widget
name – widget name
img_file – the image file data, set flg_fs true when using filesystem
x – left
y – top
- 返回:
gui_perspective_t* widget pointer
-
struct gui_perspective_imgfile_t
- #include <gui_perspective.h>
…
Public Members
-
IMG_SOURCE_MODE_TYPE src_mode[6]
flag: indicate file src
-
char *img_path[6]
images file path
-
void *data_addr[6]
images memory address
-
union gui_perspective_imgfile_t.[anonymous] [anonymous]
-
IMG_SOURCE_MODE_TYPE src_mode[6]
-
struct gui_perspective_t
- #include <gui_perspective.h>