可缩放矢量图形
可缩放矢量图形(SVG)可以展示SVG格式的图像。
SVG是一种用于描述二维图形的 XML 标记语言,与位图图像不同,SVG图像以文本形式存储,并且可以缩放到任意大小而不会失真,因为它们基于数学描述而不是像素。
SVG图像可以直接打包到
root
文件夹。
用法
创建控件
使用gui_svg_create_from_mem(parent, name, addr, size, x, y, w, h)以从内存地址加载图像的方式创建一个SVG控件, 或者使用gui_svg_create_from_file(parent, name, filename, x, y, w, h)以从文件系统路径加载图像的方式创建一个SVG控件。
其中,w/h
是SVG控件的宽度和高度,而不是要绘制的SVG图像的宽高,size
是必须填写的SVG图像的绘制数据大小。
设置旋转
使用gui_svg_rotation(svg, degrees, c_x, c_y)将SVG图像旋转degree
角度。其中,(c_x, c_y)
是旋转中心。
设置缩放
如果需要更新SVG图像的大小,可以使用gui_svg_scale(svg, scale_x, scale_y)。
设置平移
使用gui_svg_translate(svg, t_x, t_y)平移SVG图像。
设置透明度
使用gui_svg_set_opacity(svg, opacity_value)设置SVG图像透明度。
示例
创建一个简单的 SVG
#include "gui_svg.h"
#include "root_image_hongkong/ui_resource.h"
void page_tb_svg(void *parent)
{
gui_svg_t* svg1=gui_svg_create_from_mem(parent,"svg1",ACTIVITY_SVG,5184,0,0,100,100);
}
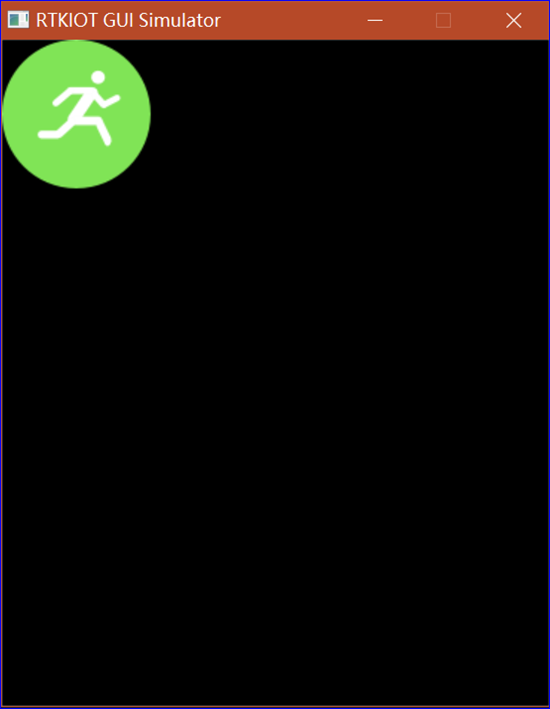
SVG 旋转
#include "gui_svg.h"
#include "root_image_hongkong/ui_resource.h"
void page_tb_svg(void *parent)
{
gui_svg_t* svg1=gui_svg_create_from_mem(parent,"svg1",ACTIVITY_SVG,5184,0,0,100,100);
gui_svg_rotation(svg1,90,50,50);
}

SVG 缩放
#include "gui_svg.h"
#include "root_image_hongkong/ui_resource.h"
void page_tb_svg(void *parent)
{
gui_svg_t* svg1=gui_svg_create_from_mem(parent,"svg1",ACTIVITY_SVG,5184,0,0,100,100);
gui_svg_scale(svg1,2,1);
}
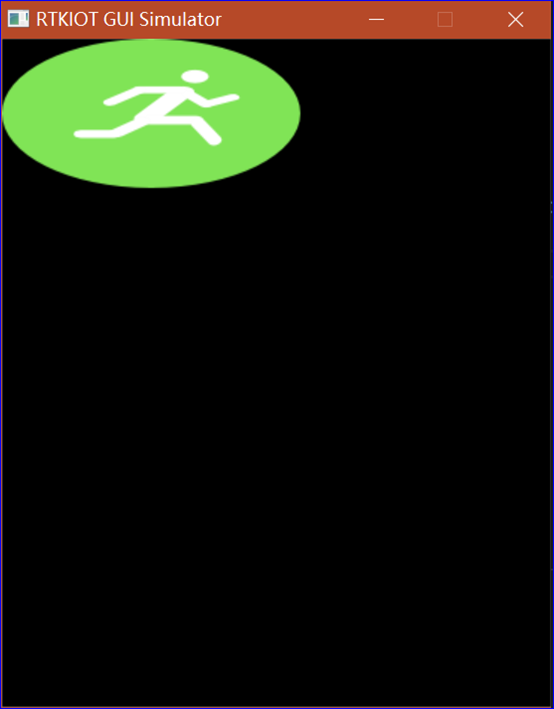
SVG 平移
#include "gui_svg.h"
#include "root_image_hongkong/ui_resource.h"
void page_tb_svg(void *parent)
{
gui_svg_t* svg1=gui_svg_create_from_mem(parent,"svg1",ACTIVITY_SVG,5184,0,0,100,100);
gui_svg_translate(svg1,100,100);
}
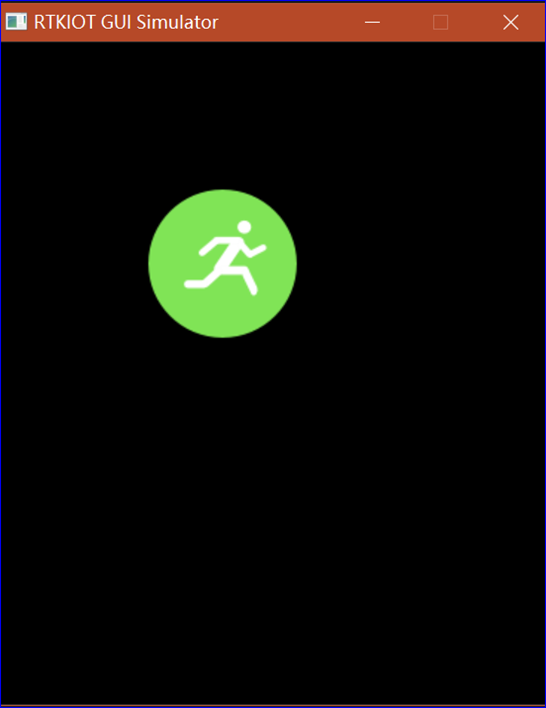
API
Functions
-
gui_svg_t *gui_svg_create_from_file(void *parent, const char *name, const char *filename, int16_t x, int16_t y, int16_t w, int16_t h)
create a svg widget from file,which should be display an SVG image.
- 参数:
parent – the father widget nested in
name – this svg widget’s name.
filename – this svg image’s filename
x – the X-axis coordinate relative to parent widget
y – the Y-axis coordinate relative to parent widget
w – width
h – height
- 返回:
gui_svg_t*
-
gui_svg_t *gui_svg_create_from_mem(void *parent, const char *name, uint8_t *addr, uint32_t size, int16_t x, int16_t y, int16_t w, int16_t h)
create a svg widget from memory,which should be display an SVG image
- 参数:
parent – the father widget nested in
name – this svg widget’s name.
addr – change svg address
size – the size of svg
x – the X-axis coordinate relative to parent widget
y – the Y-axis coordinate relative to parent widget
w – width
h – height
- 返回:
gui_svg_t*
-
void gui_svg_rotation(gui_svg_t *svg, float degrees, float c_x, float c_y)
set svg rotation angle
- 参数:
svg – the svg widget pointer
degrees – rotation angle
c_x – the X-axis coordinate of the rotation center
c_y – the Y-axis coordinate of the rotation center
-
void gui_svg_scale(gui_svg_t *svg, float scale_x, float scale_y)
scale the svg
- 参数:
svg – the svg widget pointer
scale_x – scale in the X-axis direction
scale_y – scale in the Y-axis direction
-
struct gui_svg_t
- #include <gui_svg.h>
svg structure