开关控件
开关控件是一个自定义的开关按钮控件,常用于启用或禁用某些功能或选项,允许开发者通过切换按钮的状态来控制应用程序的行为。
用法
创建控件
开发者可以调用 gui_switch_t * gui_switch_create(parent, x, y, w, h, off_pic, on_pic) 或者 gui_switch_create_from_ftl(void *parent, int16_t x, int16_t y, int16_t w, int16_t h, void *off_pic, void *on_pic)从内存地址创建开关控件,使用gui_switch_t * gui_switch_create_frome_fs(parent, x, y, w, h, off_pic, on_pic) 从文件系统创建开关控件。 可以参考gui_switch_t中的具体参数,例如base、switch_picture、on_pic_addr、off_pic_addr等。
开关控件的参数
struct gui_switch
{
gui_obj_t base;
gui_img_t *switch_picture;
void *on_pic_addr;
void *off_pic_addr;
void *on_hl_pic_addr;
void *off_hl_pic_addr;
void *long_touch_state_pic_addr;
void *long_touch_state_hl_pic_addr;
void *data;
gui_animate_t *animate; //!< can set animation
IMG_SOURCE_MODE_TYPE src_mode;
char style;
uint32_t ifon : 1;
uint32_t long_touch_state : 1;
uint32_t long_touch_enable : 1;
uint32_t press_flag : 1;
uint32_t long_flag : 1;
uint32_t release_flag : 1;
uint32_t touch_disable : 1;
uint32_t checksum : 8;
};
开/关
开发者可以使用以下API来打开或关闭开关控件,并触发事件(由gui_obj_add_event_cb创建)。
gui_switch_turn_on(gui_switch_t *this) 或者 gui_switch_turn_off(gui_switch_t *this)。
更新开关状态
如果已经打开/关闭了开关控件,开发者可以通过以下API将状态更新为打开或关闭。
gui_switch_is_on(gui_switch_t *this) 或者 gui_switch_is_off(gui_switch_t *this)。
改变开关控件状态
开发者可以调用 gui_switch_change_state(gui_switch_t *this, bool ifon)来设置开关控件的状态并改变UI,但不会触发任何事件(由gui_obj_add_event_cb创建)。
示例
示例代码
#include "root_image_hongkong/ui_resource.h"
#include "gui_switch.h"
#include "gui_img.h"
static gui_img_t *img;
static void img_animate(gui_img_t *img)
{
gui_log("%f\n", img->animate->progress_percent);
if (img->animate->progress_percent < 0.5f)
{
GET_BASE(img)->y = img->animate->progress_percent * 2 * 100 - 100;
}
else if (img->animate->progress_percent >= 0.5f)
{
GET_BASE(img)->y = (1 - img->animate->progress_percent) * 2 * 100 - 100;
}
if (img->animate->progress_percent == 1.0f)
{
img->animate->current_repeat_count = 0;
img->base.not_show = true;
}
}
static void reset_animate()
{
img->animate->animate = true;
img->base.not_show = false;
img->animate->current_frame = 0;
img->animate->current_repeat_count = 0;
img->animate->progress_percent = 0;
}
static void callback_disturb_on()
{
reset_animate();
img->draw_img->data = WURAOKAI_BIN;
}
static void callback_disturb_off()
{
reset_animate();
img->draw_img->data = WURAOGUAN_BIN;
}
static void callback_mute_on()
{
reset_animate();
img->draw_img->data = JINGYINKAI_BIN;
}
static void callback_mute_off()
{
reset_animate();
img->draw_img->data = JINGYINGUAN_BIN;
}
static void callback_call_on()
{
reset_animate();
img->draw_img->data = DIANHUAKAI_BIN;
}
static void callback_call_off()
{
reset_animate();
img->draw_img->data = DIANHUAGUAN_BIN;
}
static void callback_bright_on()
{
reset_animate();
img->draw_img->data = LIANGDUKAI_BIN;
}
static void callback_bright_off()
{
reset_animate();
img->draw_img->data = LIANGDUGUAN_BIN;
}
static void callback_watch_on()
{
reset_animate();
img->draw_img->data = SHIZHONGKAI_BIN;
}
static void callback_watch_off()
{
reset_animate();
img->draw_img->data = SHIZHONGGUAN_BIN;
}
static void callback_set_on()
{
reset_animate();
img->draw_img->data = SHEZHIKAI_BIN;
}
static void callback_set_off()
{
reset_animate();
img->draw_img->data = SHEZHIGUAN_BIN;
}
void page_tb_control0(void *parent)
{
// gui_img_creat_from_mem(parent, "parent", CONTROLMENU_0_BIN, 0, 0, 0, 0);
gui_switch_t *sw_no_disturb = gui_switch_create(parent, 10, 108, 169, 98, NO_DISTURB_OFF_BIN,
NO_DISTURB_ON_BIN);
gui_switch_t *sw_mute = gui_switch_create(parent, 190, 108, 169, 98, MUTE_OFF_BIN,
MUTE_ON_BIN);
gui_switch_t *sw_call = gui_switch_create(parent, 10, 220, 169, 98, CALL_OFF_BIN,
CALL_ON_BIN);
gui_switch_t *sw_bright = gui_switch_create(parent, 190, 220, 169, 98, BRIGHT_OFF_BIN,
BRIGHT_ON_BIN);
gui_switch_t *sw_watch = gui_switch_create(parent, 10, 332, 169, 98, WATCH_OFF_BIN,
WATCH_ON_BIN);
gui_switch_t *sw_set = gui_switch_create(parent, 190, 332, 169, 98, SET_OFF_BIN,
SET_ON_BIN);
img = gui_img_create_from_mem(GET_BASE(parent)->parent, 0, WURAOKAI_BIN, 0, 0, 0, 0);
gui_img_set_animate(img, 1000, 1, img_animate, img);
img->animate->animate = false;
img->base.not_show = true;
gui_obj_add_event_cb(sw_no_disturb, (gui_event_cb_t)callback_disturb_on, GUI_EVENT_1, NULL);
gui_obj_add_event_cb(sw_no_disturb, (gui_event_cb_t)callback_disturb_off, GUI_EVENT_2, NULL);
gui_obj_add_event_cb(sw_mute, (gui_event_cb_t)callback_mute_on, GUI_EVENT_1, NULL);
gui_obj_add_event_cb(sw_mute, (gui_event_cb_t)callback_mute_off, GUI_EVENT_2, NULL);
gui_obj_add_event_cb(sw_call, (gui_event_cb_t)callback_call_on, GUI_EVENT_1, NULL);
gui_obj_add_event_cb(sw_call, (gui_event_cb_t)callback_call_off, GUI_EVENT_2, NULL);
gui_obj_add_event_cb(sw_bright, (gui_event_cb_t)callback_bright_on, GUI_EVENT_1, NULL);
gui_obj_add_event_cb(sw_bright, (gui_event_cb_t)callback_bright_off, GUI_EVENT_2, NULL);
gui_obj_add_event_cb(sw_watch, (gui_event_cb_t)callback_watch_on, GUI_EVENT_1, NULL);
gui_obj_add_event_cb(sw_watch, (gui_event_cb_t)callback_watch_off, GUI_EVENT_2, NULL);
gui_obj_add_event_cb(sw_set, (gui_event_cb_t)callback_set_on, GUI_EVENT_1, NULL);
gui_obj_add_event_cb(sw_set, (gui_event_cb_t)callback_set_off, GUI_EVENT_2, NULL);
}
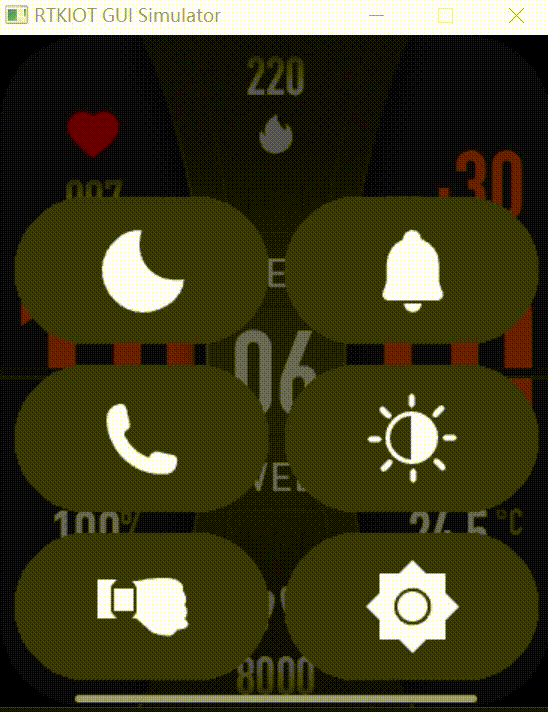
API
Defines
-
SWITCH_HIGHLIGHT_ARRAY
Enums
Functions
-
gui_switch_t *gui_switch_create(void *parent, int16_t x, int16_t y, int16_t w, int16_t h, void *off_pic, void *on_pic)
create a switch widget, file source is memory address.
- 参数:
parent – the father widget it nested in.
x – the X-axis coordinate of the widget.
y – the Y-axis coordinate of the widget.
w – the width of the widget.
h – the hight of the widget.
off_pic – off status image memory address.
on_pic – on status image memory address.
- 返回:
return the widget object pointer.
-
gui_switch_t *gui_switch_create_from_fs(void *parent, int16_t x, int16_t y, int16_t w, int16_t h, void *off_pic, void *on_pic)
create a switch widget, file source is filesystem.
- 参数:
parent – the father widget it nested in.
x – the X-axis coordinate of the widget.
y – the Y-axis coordinate of the widget.
w – the width of the widget.
h – the hight of the widget.
off_pic – off status image filepath.
on_pic – on status image filepath.
- 返回:
return the widget object pointer.
-
gui_switch_t *gui_switch_create_from_ftl(void *parent, int16_t x, int16_t y, int16_t w, int16_t h, void *off_pic, void *on_pic)
create a switch widget, file source is memory address
- 参数:
parent – the father widget it nested in.
x – the X-axis coordinate of the widget.
y – the Y-axis coordinate of the widget.
w – the width of the widget.
h – the hight of the widget.
off_pic – off status image filepath.
on_pic – on status image filepath.
- 返回:
return the widget object pointer.
-
void gui_switch_turn_on(gui_switch_t *this)
Turn on the switch, event will be triggered.
- 参数:
this –
-
void gui_switch_turn_off(gui_switch_t *this)
Turn off the switch, event will be triggered.
- 参数:
this –
-
void gui_switch_is_on(gui_switch_t *this)
If the switch has been turned on somehow, it can upadte the status to on status.
- 参数:
this –
-
void gui_switch_is_off(gui_switch_t *this)
If the switch has been turned off somehow, it can upadte the status to off status.
- 参数:
this –
-
void gui_switch_change_state(gui_switch_t *this, bool ifon)
set sw state and change ui, NO event will be trigered.
- 参数:
this –
ifon – set sw state
Variables
-
void (*turn_off)(gui_switch_t *sw)
-
void (*turn_on)(gui_switch_t *sw)
-
void (*on_turn_on)(gui_switch_t *this, void *cb, void *p)
-
void (*on_turn_off)(gui_switch_t *this, void *cb, void *p)
-
void (*on_press)(gui_switch_t *this, gui_event_cb_t event_cb, void *parameter)
-
void (*on_release)(gui_switch_t *this, gui_event_cb_t event_cb, void *parameter)
-
void (*ctor)(gui_switch_t *this, gui_obj_t *parent, int16_t x, int16_t y, int16_t w, int16_t h, void *off_pic, void *on_pic)
-
void (*animate)(gui_switch_t *this, uint32_t dur, int repeat_count, void *callback, void *p)
-
struct gui_switch
- #include <gui_switch.h>
Public Members
-
void *on_pic_addr
-
void *off_pic_addr
-
void *on_hl_pic_addr
-
void *off_hl_pic_addr
-
void *long_touch_state_pic_addr
-
void *long_touch_state_hl_pic_addr
-
void *data
-
gui_animate_t *animate
can set animation
-
IMG_SOURCE_MODE_TYPE src_mode
-
char style
-
uint32_t ifon
-
uint32_t long_touch_state
-
uint32_t long_touch_enable
-
uint32_t press_flag
-
uint32_t long_flag
-
uint32_t release_flag
-
uint32_t touch_disable
-
uint32_t checksum
-
void *on_pic_addr