监视点渐变
监视点渐变是一个具有动态水滴效果的指针表盘控件。
用法
创建控件
使用 gui_watch_gradient_spot_create(parent, name, x, y, w, h) 函数创建一个具有动态水滴效果的指针表盘控件。
设置中心位置
控件的中心位置可以自由设置,使用gui_watch_gradient_spot_set_center(this, c_x, c_y) 函数改变中心位置,c_x, c_y 为中心位置的坐标点。
示例
code
#include "root_image_hongkong/ui_resource.h"
#include "gui_img.h"
#include "gui_win.h"
#include "gui_watch_gradient_spot.h"
#include "gui_text.h"
#include "gui_watchface_gradient.h"
#include "gui_cardview.h"
#include "gui_card.h"
#include "gui_tab.h"
#include "gui_app.h"
gui_win_t *win_watch;
gui_img_t *img;
gui_watch_gradient_spot_t *watch;
gui_text_t *rate;
static gui_watchface_gradient_t *canvas;
gui_tabview_t *tablist_tab;
void tablist_watch(void *parent)
{
gui_watch_gradient_spot_t *watch = gui_watch_gradient_spot_create(parent, "watchface", 0, 0, 221,
269);
gui_watch_gradient_spot_set_center(watch, 221, 269);
gui_img_t *rect = gui_img_create_from_mem(parent, "rect", RECT_221_269_BIN, 0, 0, 0, 0);
gui_img_set_mode(rect, IMG_SRC_OVER_MODE);
}
static void callback_touch_long(void *obj, gui_event_t e)
{
gui_log("win widget long touch enter cb\n");
gui_app_t *app = (gui_app_t *)get_app_hongkong();
gui_obj_t *screen = &(app->screen);
int idx = 0;
if (!GET_BASE(img)->not_show)
{
idx = 0;
}
if (!GET_BASE(watch)->not_show)
{
idx = 1;
}
if (!GET_BASE(canvas)->not_show)
{
idx = 2;
}
gui_obj_tree_free(screen);
gui_win_t *win = gui_win_create(screen, "win", 0, 0, 320, 320);
#if ENABLE_RTK_GUI_WATCHFACE_UPDATE
extern void create_tree_nest(char *xml, void *obj);
create_tree_nest("gui_engine\\example\\screen_448_368\\root_image_hongkong\\watch_face_update\\app\\wf\\wf.xml",
win);
return;
#endif
gui_obj_add_event_cb(win, (gui_event_cb_t)callback_time, GUI_EVENT_TOUCH_CLICKED, NULL);
tablist_tab = gui_tabview_create(win, "tabview", 59, 84, 250, 300);
gui_tabview_set_style(tablist_tab, CLASSIC);
gui_tab_t *tb_watchface = gui_tab_create(tablist_tab, "tb_watchface", 0, 0, 250, 0, 2, 0);
gui_tab_t *tb_watch = gui_tab_create(tablist_tab, "tb_watch", 0, 0, 250, 0, 1, 0);
gui_tab_t *tb_clock = gui_tab_create(tablist_tab, "tb_clock", 0, 0, 250, 0, 0, 0);
gui_tabview_jump_tab(tablist_tab, idx, 0);
extern void tablist_clock(void *parent);
tablist_clock(tb_clock);
extern void tablist_watch(void *parent);
tablist_watch(tb_watch);
extern void tablist_watchface(void *parent);
tablist_watchface(tb_watchface);
}
void page_ct_clock(void *parent)
{
win_watch = gui_win_create(parent, "win", 0, 0, 368, 448);
gui_obj_add_event_cb(win_watch, (gui_event_cb_t)callback_touch_long, GUI_EVENT_TOUCH_LONG, NULL);
img = gui_img_create_from_mem(parent, "page0", CLOCKN_BIN, 0, 0, 0, 0);
gui_img_set_mode(img, IMG_SRC_OVER_MODE);
watch = gui_watch_gradient_spot_create(win_watch, "watchface", 0, 0, 0, 0);
gui_watch_gradient_spot_set_center(watch, 368 / 2, 448 / 2);
canvas = gui_watchface_gradient_create(parent, "watchface_gradient", (368 - 368) / 2,
(448 - 448) / 2, 368, 448);
GET_BASE(watch)->not_show = true;
GET_BASE(canvas)->not_show = true;
}
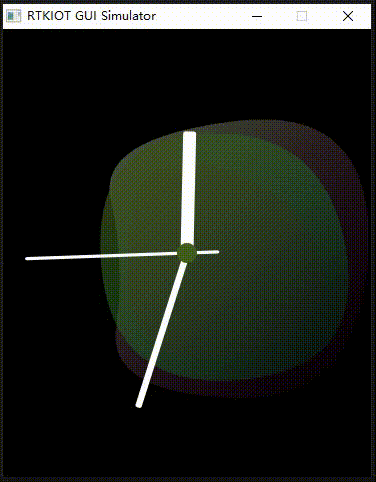
API
Functions
-
NVGcontext *nvgCreateAGGE(uint32_t w, uint32_t h, uint32_t stride, enum NVGtexture format, uint8_t *data)
-
void nvgDeleteAGGE(NVGcontext *ctx)
-
gui_watch_gradient_spot_t *gui_watch_gradient_spot_create(void *parent, const char *name, int16_t x, int16_t y, int16_t w, int16_t h)
create a watch widget
Example usage
void example_watch(void *parent) { win_watch = gui_win_create(parent, "win", 0, 0, 368, 448); gui_watch_gradient_spot_t *watch = gui_watch_gradient_spot_create(win_watch, "watchface", 0, 0, 0, 0); }
- 参数:
parent – parent widget
name – widget name
x – left
y – top
w – width
h – high
- 返回:
gui_watch_gradient_spot_t* widget pointer
-
void gui_watch_gradient_spot_set_center(gui_watch_gradient_spot_t *this, float c_x, float c_y)
set watch center
Example usage
void example_watch(void *parent) { win_watch = gui_win_create(parent, "win", 0, 0, 368, 448); gui_watch_gradient_spot_t *watch = gui_watch_gradient_spot_create(win_watch, "watchface", 0, 0, 0, 0); gui_watch_gradient_spot_t *watch = gui_watch_gradient_spot_set_center(watch, 368 / 2, 448 / 2); }
- 参数:
this – gui_watch_gradient_spot_t widget
c_x – left
c_y – top
- 返回:
void
-
struct gui_watch_gradient_spot_t
- #include <gui_watch_gradient_spot.h>
…