Output Toggle
This example is based on the output function of GPIO, implementing periodic state toggle control of the LED.
Configure the GPIO as output mode. Within the while loop, toggle the input level every 1 second to drive the LED to blink.
Users can modify pin information through different macro configurations. For specific macro configurations, refer to Configurations.
Requirements
For requirements, please refer to the Requirements.
Wiring
Connect P1_0 to LED0. The LED driver circuit is shown below.
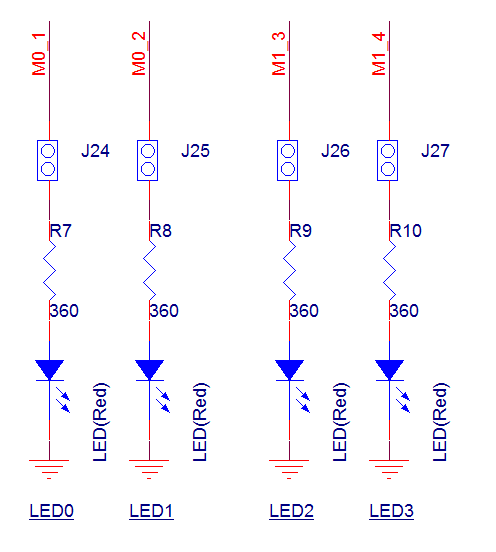
LED Driver Circuit Diagram
Configurations
The following macro can be configured to modify the pin definition.
#define OUTPUT_PIN P1_0
Building and Downloading
For building and downloading, please refer to the Building and Downloading.
Experimental Verification
After resetting the EVB, observe the log messages as shown in the Debug Analyzer.
Start gpio toggle test!
Observe that the LED is blinking.
Code Overview
This section mainly introduces the code and process description for initialization and corresponding function implementation in the example.
Source Code Directory
The directory for project file and source code are as follows:
Project directory:
sdk\samples\peripheral\gpio\output_toggle\proj
Source code directory:
sdk\samples\peripheral\gpio\output_toggle\src
Initialization
The initialization flow for peripherals can refer to Initialization Flow in General Introduction.
Call
Pad_Config()
andPinmux_Config()
to configure the PAD and PINMUX of the corresponding pins.void board_gpio_init(void) { Pad_Config(OUTPUT_PIN, PAD_PINMUX_MODE, PAD_IS_PWRON, PAD_PULL_NONE, PAD_OUT_ENABLE, PAD_OUT_HIGH); Pinmux_Config(OUTPUT_PIN, DWGPIO); }
Call
RCC_PeriphClockCmd()
to enable the GPIO clock.Initialize the GPIO peripheral:
Define the
GPIO_InitTypeDef
typeGPIO_InitStruct
, and callGPIO_StructInit()
to pre-fillGPIO_InitStruct
with default values.Modify the
GPIO_InitStruct
parameters as needed. The initialization parameter configurations for GPIO are shown in the table below. CallGPIO_Init()
to initialize the GPIO peripheral.
GPIO Hardware Parameters |
Setting in the |
GPIO |
---|---|---|
GPIO pin |
|
|
GPIO direction |
||
GPIO interrupt |
Functional Implementation
After initialization, call GPIO_WriteBit()
within the while loop to control the GPIO output high and low levels.
while (1)
{
/* Set GPIO_PIN_OUTPUT */
GPIO_WriteBit(GPIO_PORT, GPIO_PIN, (BitAction)(1));
platform_delay_ms(1000);
/* Reset GPIO_PIN_OUTPUT */
GPIO_WriteBit(GPIO_PORT, GPIO_PIN, (BitAction)(0));
platform_delay_ms(1000);
}