PBAP
PBAP defines the protocols and procedures that shall be used by devices for the retrieval of phone book objects. It is based on a client-server interaction model where the client device pulls phone book objects from the server device. This profile is especially tailored for the Hands-Free usage case. It provides numerous capabilities that allow for advanced handling of phone book objects, as needed in the car environment. This manual describes the PBAP API definitions and how to use these APIs to pull phone book objects from the server device.
Terminology and Concepts
PBAP Profile Stack
Figure below shows the protocols and entities used in this profile.
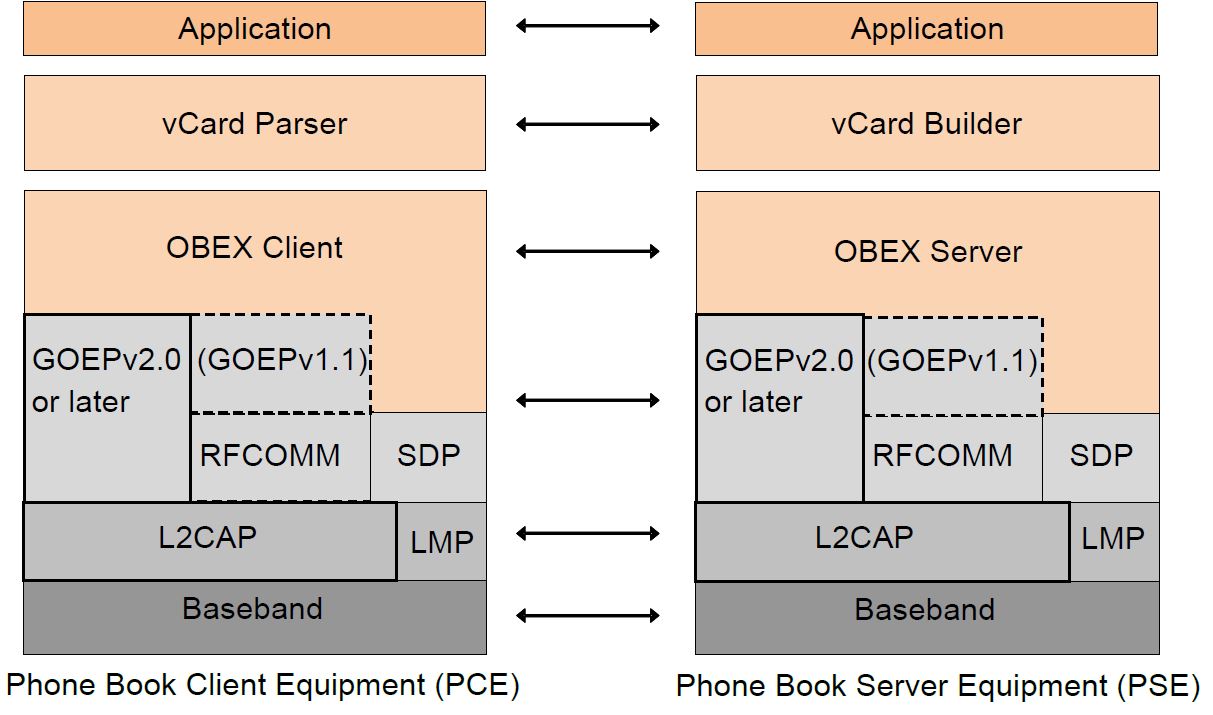
PBAP
The Baseband, LMP and L2CAP are the physical and data link layers of the Bluetooth protocols. RFCOMM is the Bluetooth serial port emulation entity. SDP is the Bluetooth Service Discovery Protocol.
PBAP Roles
PBAP Repositories
There might be several repositories for phone book objects. t_bt_pbap_repository
defines a typical example, one phone book is stored locally in the phone’s memory, and another phone book is stored on the phone’s SIM card.
PBAP Objects
t_bt_pbap_phone_book
defines seven types of phone book objects.
The main phone book object (pb) corresponds to the user phone book of the current repository.
The Incoming Calls History object (ich) corresponds to a list of the most recently received calls.
The Outgoing Calls History object (och) corresponds to a list of the most recently made calls.
The Missed Calls History object (mch) corresponds to a list of the most recently missed calls.
The Combined Calls History object (cch) corresponds to the combination of ich, och and mch.
The Speed-Dial object (spd) corresponds to the list of speed dial entries on the PSE.
The Favorite Contacts object (fav) corresponds to a list of favorites on the PSE.
PBAP Virtual Folders Structure
Phone book information is organized under the virtual folders architecture described below.
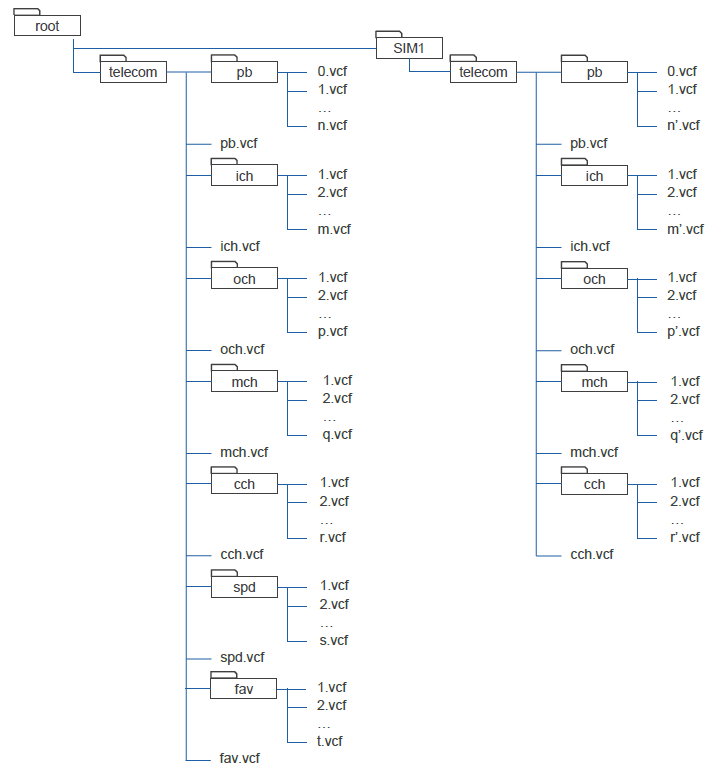
PBAP Folder
The local phone book information is located under the telecom folder.
When the PSE also contains a SIM card, SIM card phone book information shall be located under the SIM1\telecom\folder
.
PBAP SDP Record
PBAP local record pbap_pce_sdp_record
should be registered by bt_sdp_record_add()
.
const uint8_t pbap_pce_sdp_record[] =
{
SDP_DATA_ELEM_SEQ_HDR,
0x36,
//attribute SDP_ATTR_SRV_CLASS_ID_LIST
SDP_UNSIGNED_TWO_BYTE,
(uint8_t)(SDP_ATTR_SRV_CLASS_ID_LIST >> 8),
(uint8_t)SDP_ATTR_SRV_CLASS_ID_LIST,
SDP_DATA_ELEM_SEQ_HDR,
0x03,
SDP_UUID16_HDR,
(uint8_t)(UUID_PBAP_PCE >> 8),
(uint8_t)(UUID_PBAP_PCE),
//attribute SDP_ATTR_BROWSE_GROUP_LIST
SDP_UNSIGNED_TWO_BYTE,
(uint8_t)(SDP_ATTR_BROWSE_GROUP_LIST >> 8),
(uint8_t)SDP_ATTR_BROWSE_GROUP_LIST,
SDP_DATA_ELEM_SEQ_HDR,
0x03,
SDP_UUID16_HDR,
(uint8_t)(UUID_PUBLIC_BROWSE_GROUP >> 8),
(uint8_t)UUID_PUBLIC_BROWSE_GROUP,
//attribute SDP_ATTR_PROFILE_DESC_LIST
SDP_UNSIGNED_TWO_BYTE,
(uint8_t)(SDP_ATTR_PROFILE_DESC_LIST >> 8),
(uint8_t)SDP_ATTR_PROFILE_DESC_LIST,
SDP_DATA_ELEM_SEQ_HDR,
0x08,
SDP_DATA_ELEM_SEQ_HDR,
0x06,
SDP_UUID16_HDR,
(uint8_t)(UUID_PBAP >> 8),
(uint8_t)UUID_PBAP,
SDP_UNSIGNED_TWO_BYTE,
0x01,//version 1.2
0x02,
//attribute SDP_ATTR_SRV_NAME
SDP_UNSIGNED_TWO_BYTE,
(uint8_t)((SDP_ATTR_SRV_NAME + SDP_BASE_LANG_OFFSET) >> 8),
(uint8_t)(SDP_ATTR_SRV_NAME + SDP_BASE_LANG_OFFSET),
SDP_STRING_HDR,
0x14,
0x50, 0x68, 0x6f, 0x6e, 0x65, 0x62, 0x6f, 0x6f, 0x6b, 0x20, 0x41, 0x63, 0x63, 0x65, 0x73, 0x73, 0x20, 0x50, 0x43, 0x45 //"Phonebook Access PCE"
};
Class of Device Field
There is no obvious correlation between the COD field and the support for PBAP since it is expected that many different types of devices will implement PBAP.
Note
The PSE may implement policies that prevent the device from accessing the phonebook privileges of the phone. Making the COD to Car Audio or Hands-Free may be practicable.
Feature Set
The functions provided by PBAP API are as below.
Connecting and Disconnecting: Including functions to connect/disconnect PBAP.
Getting phone book: Including functions to retrieve the phone book object from PSE.
Provided APIs
This figure shows the relationship between application, PBAP and Bluetooth Protocol Stack. Above the horizon line is developed by the application. Below the horizon line is developed by Realtek.
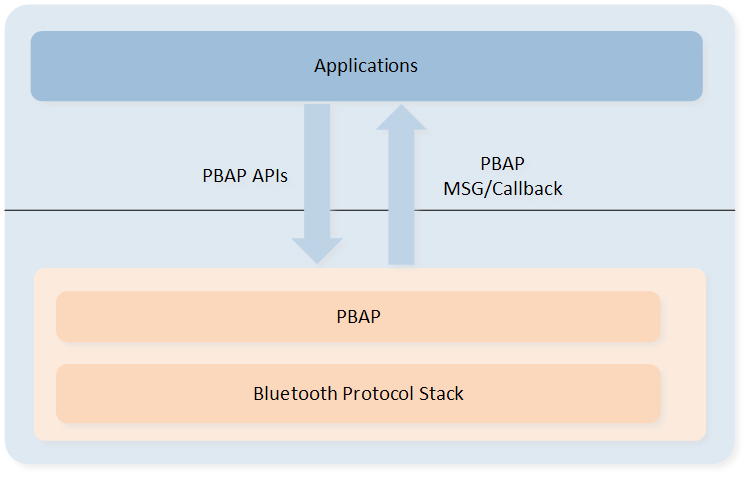
PBAP Struct
The table below shows a simple description of PBAP APIs. PBAP related APIs are provided in the sdk\inc\framework\bt\bt_pbap.h
.
Header File |
Description |
API Reference |
---|---|---|
|
Connect PBAP, get phone book etc. |
Functions
PBAP Initialization
bt_pbap_init()
is invoked when the application is powered on or the chip is reset.
int main(void)
{
//board init
bt_pbap_init();
//task create
return 0;
}
PBAP Service Discovery
Service discovery procedure is shown below:
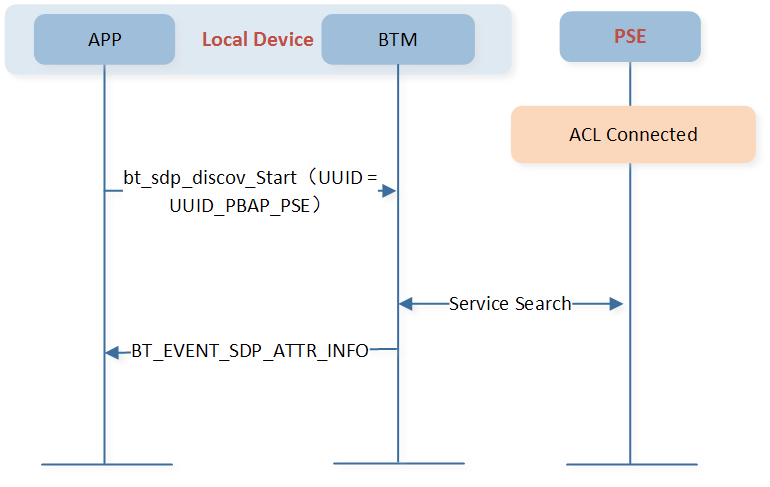
PBAP Service Discovery Procedure
Note
UUID_PBAP_PSE
shall be used to do service discovery. It is not recommended to use UUID_PBAP
, as the SDP record of PCE and PSE may both be obtained from the PSE device.
PBAP Connect
OBEX connections in PBAP shall always be initiated by the PCE device using bt_pbap_connect_over_rfc_req()
or bt_pbap_connect_over_l2c_req()
, as shown below:
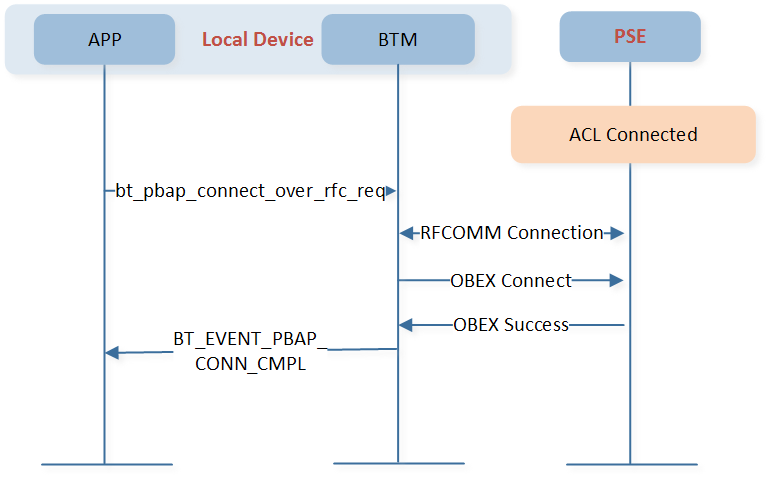
PBAP Connect over RFCOMM
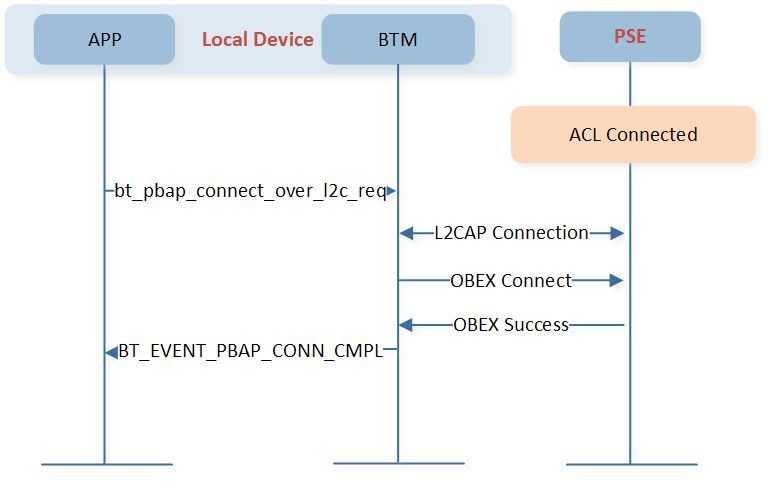
PBAP Connect over L2CAP
The authorization process, which requires user participation, takes time to complete. So do not initiate a PBAP connection immediately after pairing with the phone. It is recommended to establish a PBAP connection when actually needed.
void app_pbap_bt_cback(T_BT_EVENT event_type, void *event_buf, uint16_t buf_len)
{
switch (event_type)
{
case BT_EVENT_SDP_ATTR_INFO:
{
T_BT_SDP_ATTR_INFO *sdp_info = ¶m->sdp_attr_info.info;
if (sdp_info->srv_class_uuid_data.uuid_16 == UUID_PBAP_PSE)
{
bt_pbap_connect_req(param->sdp_attr_info.bd_addr, sdp_info->server_channel,
sdp_info->pbap_supported_feat);
}
}
break;
case BT_EVENT_PBAP_CONN_CMPL:
break;
}
}
PBAP Pull
Call bt_pbap_phone_book_size_get()
if PCE wants to know the number of used indexes in the phone book of interest.
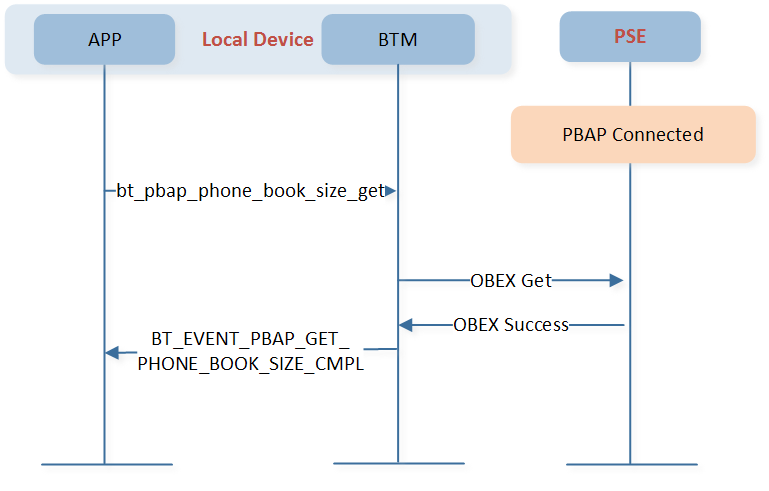
PBAP Phone Book Size Get
bt_pbap_phone_book_pull()
and bt_pbap_pull_continue()
are usually used together to retrieve an entire phone book object from the PSE device.
Call bt_pbap_pull_abort()
if the phonebook pull procedure needs to be interrupted, while the PBAP connection need to be maintained.
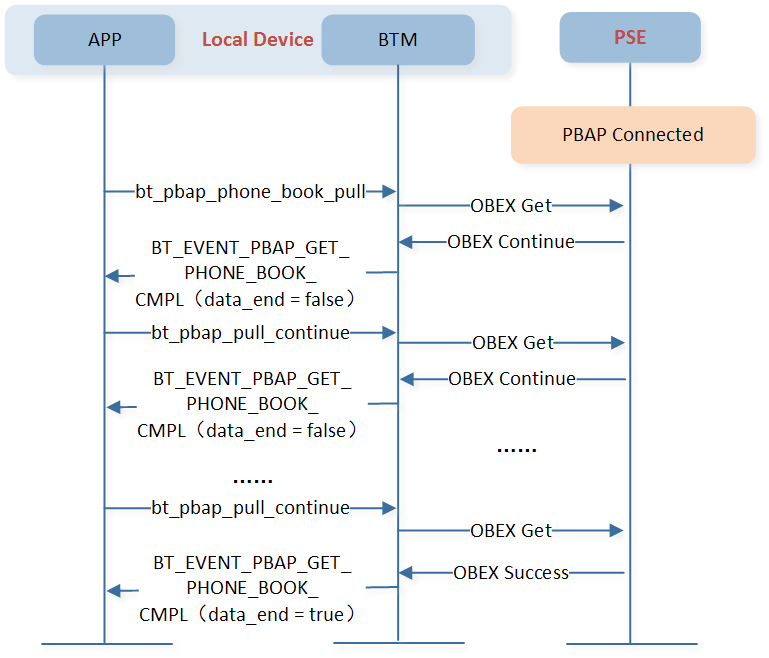
PBAP Phone Book Pull
void app_pbap_bt_cback(T_BT_EVENT event_type, void *event_buf, uint16_t buf_len)
{
T_BT_EVENT_PARAM *param = event_buf;
switch (event_type)
{
case BT_EVENT_PBAP_GET_PHONE_BOOK_SIZE_CMPL:
{
int size;
size = param->pbap_get_phone_book_size_cmpl.pb_size;
}
break;
case BT_EVENT_PBAP_SET_PHONE_BOOK_CMPL:
{
uint64_t filter = BT_PBAP_PROPERTY_VERSION | BT_PBAP_PROPERTY_FN |
BT_PBAP_PROPERTY_N | BT_PBAP_PROPERTY_TEL;
bt_pbap_phone_book_pull(param->pbap_conn_cmpl.bd_addr, BT_PBAP_REPOSITORY_LOCAL,
BT_PBAP_PHONE_BOOK_PB, 0, 0xFFFF, filter);
}
break;
case BT_EVENT_PBAP_GET_PHONE_BOOK_CMPL:
{
//deal with data
if (!param->pbap_get_phone_book_cmpl.data_end)
{
bt_pbap_pull_continue();
}
}
break;
}
}
bt_pbap_phone_book_set()
is used for the PCE to select the phone book object of interest.
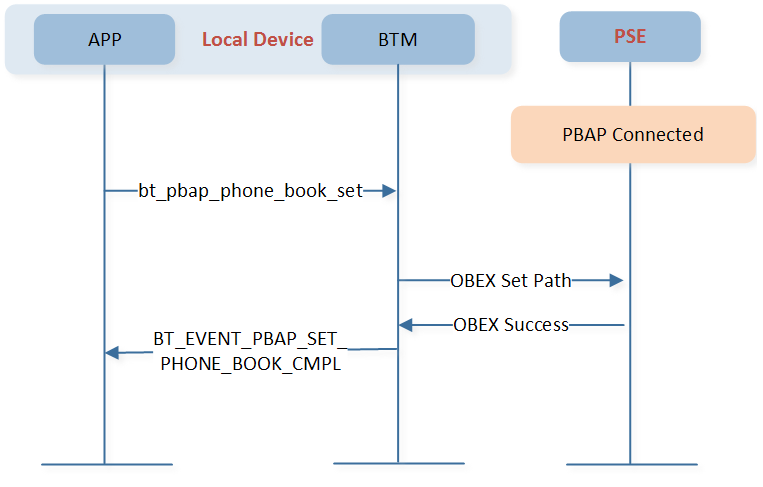
PBAP Phone Book Set
bt_pbap_vcard_listing_by_number_pull()
is used to acquire the caller’s name when a call is coming.
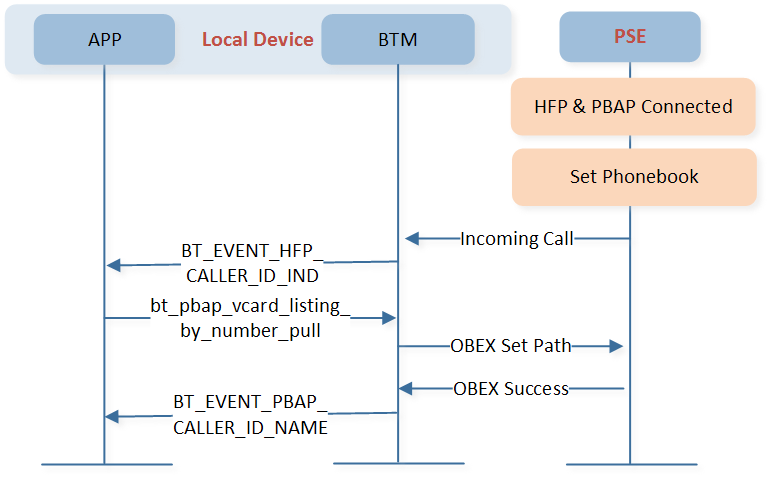
PBAP Listing by Number Pull
This function will retrieve the vCard Listing object of the current folder. It is recommended to set the folder to root\telecom\pb
after PBAP connection is established.
void app_hfp_bt_cback(T_BT_EVENT event_type, void *event_buf, uint16_t buf_len)
{
T_BT_EVENT_PARAM *param = event_buf;
switch (event_type)
{
case BT_EVENT_HFP_CALLER_ID_IND:
{
char *number;
number = (char *)param->hfp_caller_id_ind.number;
bt_pbap_vcard_listing_by_number_pull(param->hfp_caller_id_ind.bd_addr, number);
}
break;
}
}
void app_pbap_bt_cback(T_BT_EVENT event_type, void *event_buf, uint16_t buf_len)
{
T_BT_EVENT_PARAM *param = event_buf;
switch (event_type)
{
case BT_EVENT_PBAP_CONN_CMPL:
{
bt_pbap_phone_book_set(param->pbap_conn_cmpl.bd_addr, BT_PBAP_REPOSITORY_LOCAL, BT_PBAP_PATH_PB);
}
break;
case BT_EVENT_PBAP_SET_PHONE_BOOK_CMPL:
break;
case BT_EVENT_PBAP_CALLER_ID_NAME:
if (param->pbap_caller_id_name.name_len)
{
uint8_t *data;
uint16_t len;
len = param->pbap_caller_id_name.name_len + 3;
data = os_mem_alloc(RAM_TYPE_DATA_ON, len);
if (data != NULL)
{
data[0] = 0;
data[1] = CALLER_ID_TYPE_NAME;
data[2] = param->pbap_caller_id_name.name_len;
memcpy(data + 3, param->pbap_caller_id_name.name_ptr, param->pbap_caller_id_name.name_len);
app_report_event(CMD_PATH_SPP, EVENT_CALLER_ID, 0, data, len);
os_mem_free(data);
}
}
break;
}
}
PBAP Disconnect
When PBAP connection is no longer needed, call bt_pbap_disconnect_req()
to terminate the PBAP connection.
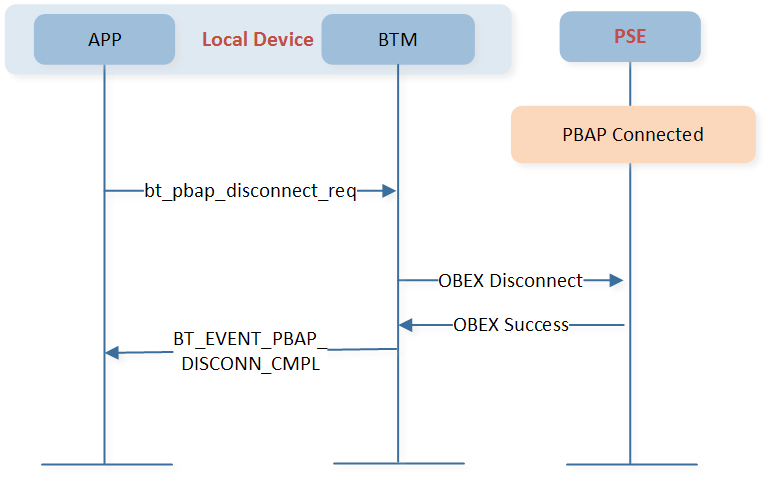
PBAP Disconnect
Sample Projects
SDK provides a corresponding demo application for developers’ reference in development. The BR/EDR PBAP gives a simple example of how to use PBAP as PCE role.