BR/EDR PBAP
PBAP defines the protocols and procedures that shall be used by devices for the retrieval of phone book objects. The purpose of this document is to give an overview of the PBAP demo. More knowledge about PBAP profile can be found in PBAP. The PBAP demo project gives a simple example of how to use PBAP. This project implements the client role for PBAP.
Requirements
The sample supports the following development kits:
Hardware Platforms |
Board Name |
Build Target |
---|---|---|
RTL87x3E EVB |
|
|
RTL87x3E EVB |
|
|
RTL87x3D HDK |
RTL87x3D EVB |
|
Note
To purchase EVB, please visit https://www.realmcu.com/en/Home/Shop.
This sample project can be found under board\evb\bt_pbap_demo
in SDK folder structure. Users can choose the project according to the Board Name
and choose the Build Target
according to the flash map.
When built for an xxx_4M_xxx
build target, the sample is configured to build and run with a 4M flash map.
When built for an xxx_8M_xxx
build target, the sample is configured to build and run with an 8M flash map.
When built for an xxx_16M_xxx
build target, the sample is configured to build and run with a 16M flash map.
The sample also requires a phone which supports PBAP.
To quickly set up the development environment, please refer to the detailed instructions provided in Quick Start.
Configurations
-
UART receiver/transmitter PINMUX is configured by the macro definition
PBAP_DEMO_UART_RX
andPBAP_DEMO_UART_TX
in the filesdk\src\sample\bt_pbap_demo\app_bt_pbap_demo_main.c
. -
DLPS is configured by the macro definition
F_DLPS_EN
in the filesdk\src\sample\bt_pbap_demo\app_flags.h
. If testing with DLPS enabled is needed, please enable the macro definitionF_DLPS_EN
.void pwr_mgr_init(void) { #if F_DLPS_EN bt_power_mode_set(BTPOWER_DEEP_SLEEP); power_mode_set(POWER_DLPS_MODE); #endif }
Building and Downloading
Take the project rtl87x3e_pbap_demo.uvprojx
and target bt_pbap_demo_4M_bank0
as an example, to build and run the sample with Keil development environment, follow the steps listed below.
Open
rtl87x3e_pbap_demo.uvprojx
.Choose the build target
bt_pbap_demo_4M_bank0
.
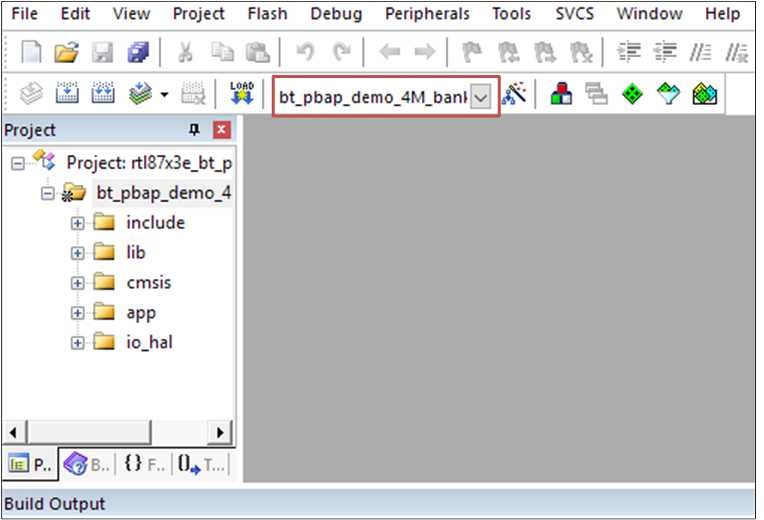
PBAP Demo Project Target
Build the target. After a successful build, the APP bin file
bt_pbap_demo_bank0_MP-v0.0.0.0-xxx.bin
will be generated in the directorybin\rtl87x3e\flash_4M_dualbank\bank0
.
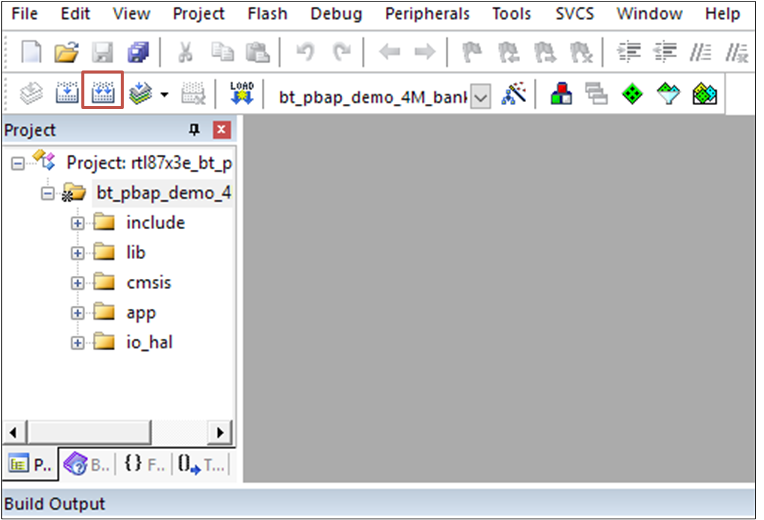
PBAP Demo Project Build
Download APP bin into EVB board.
Press Reset button on the EVB board, and it will be connectable.
Experimental Verification
This section describes the testing process and expected test results of PBAP demo. The user needs to perform the following preparations before testing PBAP demo functions.
One evolution board shall be prepared, and PBAP demo application shall be downloaded.
This test requires the phone’s Bluetooth to be turned on.
The pins of UART is defined in
pbap_demo_main.c
.
Note
The pins needs to be wired in reverse. Therefore, the TX of UART is connected to P3_0, and RX is connected to P3_1, so as to connect with the serial port.
Debug Analyzer Tool is used to get the evolution board log.
To implement the complex functions, the user needs to refer to the manuals and source codes provided by SDK for development.
As iPhone can’t establish only PBAP connection, the user should use an Android device as the remote device.
Then, following the steps below to continue the test procedure.
UART Command to Input |
Output of UART Response |
Description |
---|---|---|
pbap connect 11 22 33 44 55 66 |
PBAP Connected! |
Connect PBAP with remote device. |
pbap set_phone_book local telecom |
PBAP set phone book complete! |
Set phone book. |
pbap pull_caller_id_name 13512345678 |
The caller id name |
Get name with phone number. |
Code Overview
Source Code Directory
Project directory:
sdk\board\evb\pbap_demo
.Project source code directory:
sdk\src\sample\pbap_demo
.
Source files in the sample project are currently categorized into several groups as below.
└── Project: bt_pbap_demo_16M_bank0
├── include ROM UUID header files. Users do not need to modify it.
├── Lib Includes all binary symbol files that user application is built on.
├── cmsis The cmsis source code. Users do not need to modify it.
└── APP The application source code.
├── app_bt_pbap_demo_app.c
├── app_bt_pbap_demo_console.c
├── app_bt_pbap_demo_gap.c
├── app_bt_pbap_demo_link.c
├── app_bt_pbap_demo_main.c
├── app_bt_pbap_demo_sdp.c
├── app_bt_pbap_demo_task.c
├── app_console_msg.c
├── app_dlps.c
├── app_io_msg.c
├── console_uart.c
Initialization
When the application is powered on or the chip is reset, PBAP profile should be initialized.
int main(void)
{
board_init();
driver_init();
task_init();
framework_init();
app_bt_gap_init();
pbap_demo_gap_init();
pbap_demo_sdp_init();
pbap_demo_app_init();
app_pbap_cmd_register();
os_sched_start();
return 0;
}
Application should call bt_pbap_init()
to initialize PBAP profile.
void pbap_demo_app_init(uint8_t role)
{
bt_pbap_init();
...
}
PBAP SDP Record
Application should call bt_sdp_record_add()
to add PBAP SDP record.
const uint8_t pbap_demo_pce_sdp_record[] =
{
SDP_DATA_ELEM_SEQ_HDR,
0x36,
//attribute SDP_ATTR_SRV_CLASS_ID_LIST
SDP_UNSIGNED_TWO_BYTE,
(uint8_t)(SDP_ATTR_SRV_CLASS_ID_LIST >> 8),
(uint8_t)SDP_ATTR_SRV_CLASS_ID_LIST,
SDP_DATA_ELEM_SEQ_HDR,
0x03,
SDP_UUID16_HDR,
(uint8_t)(UUID_PBAP_PCE >> 8),
(uint8_t)(UUID_PBAP_PCE),
//attribute SDP_ATTR_BROWSE_GROUP_LIST
SDP_UNSIGNED_TWO_BYTE,
(uint8_t)(SDP_ATTR_BROWSE_GROUP_LIST >> 8),
(uint8_t)SDP_ATTR_BROWSE_GROUP_LIST,
SDP_DATA_ELEM_SEQ_HDR,
0x03,
SDP_UUID16_HDR,
(uint8_t)(UUID_PUBLIC_BROWSE_GROUP >> 8),
(uint8_t)UUID_PUBLIC_BROWSE_GROUP,
//attribute SDP_ATTR_PROFILE_DESC_LIST
SDP_UNSIGNED_TWO_BYTE,
(uint8_t)(SDP_ATTR_PROFILE_DESC_LIST >> 8),
(uint8_t)SDP_ATTR_PROFILE_DESC_LIST,
SDP_DATA_ELEM_SEQ_HDR,
0x08,
SDP_DATA_ELEM_SEQ_HDR,
0x06,
SDP_UUID16_HDR,
(uint8_t)(UUID_PBAP >> 8),
(uint8_t)UUID_PBAP,
SDP_UNSIGNED_TWO_BYTE,
0x01,//version 1.2
0x02,
//attribute SDP_ATTR_SRV_NAME
SDP_UNSIGNED_TWO_BYTE,
(uint8_t)((SDP_ATTR_SRV_NAME + SDP_BASE_LANG_OFFSET) >> 8),
(uint8_t)(SDP_ATTR_SRV_NAME + SDP_BASE_LANG_OFFSET),
SDP_STRING_HDR,
0x14,
0x50, 0x68, 0x6f, 0x6e, 0x65, 0x62, 0x6f, 0x6f, 0x6b, 0x20, 0x41, 0x63, 0x63, 0x65, 0x73, 0x73, 0x20, 0x50, 0x43, 0x45 //"Phonebook Access PCE"
};
void pbap_demo_sdp_init(void)
{
bt_sdp_record_add((void *)pbap_demo_pce_sdp_record);
}
PBAP Callback Message
Applications can call bt_mgr_cback_register()
to register different callback functions to handle different messages.
void pbap_demo_gap_init(void)
{
...
bt_mgr_cback_register(pbap_demo_gap_bt_cback);
}
void pbap_demo_app_init(void)
{
...
bt_mgr_cback_register(pbap_demo_app_bt_cback);
}
User Command
This section provides a detailed description of the usage of user commands for the PBAP demo application. The PBAP demo application requires inputting commands through the Data UART in the host terminal to perform interaction. This allows various actions to be executed and the application to be controlled.
PBAP Connect
The flow of the pbap connect command is to connect PBAP with a remote device.
pbap connect [remote_device_bluetooth_address]
User can input the command above to connect PBAP. The address in the command is the remote Bluetooth device. The console will print PBAP Connected! to inform the user that PBAP is connected.
Information |
Description |
---|---|
Command |
pbap connect |
Parameters |
Remote Bluetooth Address |
Usage |
Connect PBAP with a remote device. |
Example |
pbap connect 11 22 33 44 55 66 |
The reference API can be found in bt_pbap_connect_over_rfc_req()
or bt_pbap_connect_over_l2c_req()
, and the PBAP connect flow is shown below.
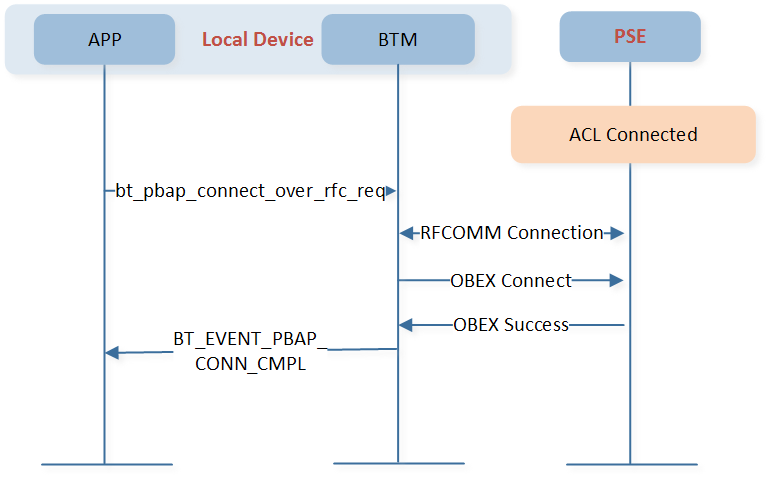
PBAP Connect over RFCOMM Flow
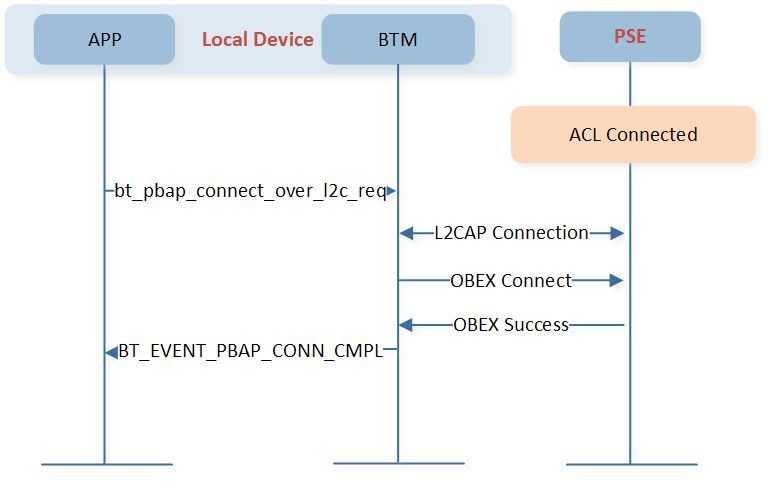
PBAP Connect over L2CAP Flow
PBAP Disconnect
The flow of the pbap disconnect command is to disconnect PBAP from the remote device.
pbap disconnect
User can input the command above to disconnect PBAP. The console will print PBAP Disconnected! to inform the user that PBAP is disconnected.
Information |
Description |
---|---|
Command |
pbap disconnect |
Usage |
Disconnect PBAP with remote device. |
Example |
pbap disconnect |
The reference API can be found in bt_pbap_disconnect_req()
, and the PBAP disconnect flow is shown below.
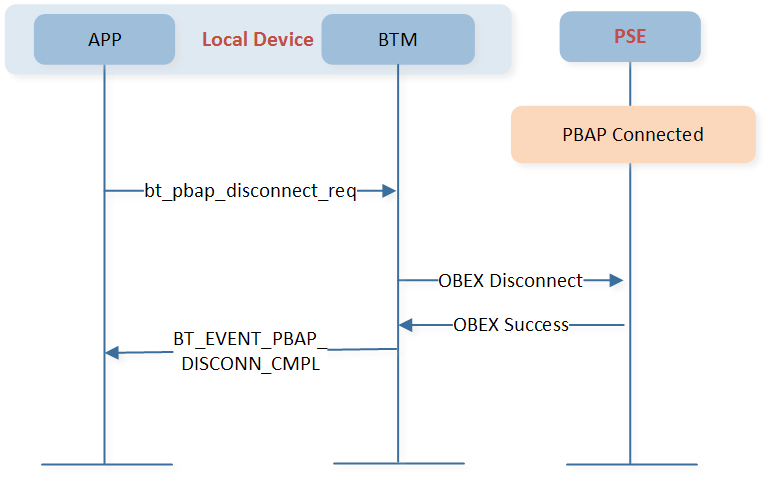
PBAP Disconnect Flow
PBAP Set Phone Book
The flow of the pbap set_phone_book command is to set the phone book.
pbap set_phone_book [phone_book_object]
User can input the command above to set the phone book. The parameter in the command is the phone book object. The console will print PBAP set phone book complete! to inform the user that the phone book is set complete.
Information |
Description |
---|---|
Command |
pbap set_phone_book |
Parameters |
Phone Book Object |
Usage |
Set phone book of remote device. |
Example |
pbap set_phone_book local telecom |
The reference API can be found in bt_pbap_phone_book_set()
, and the PBAP phone book setting flow is shown below.
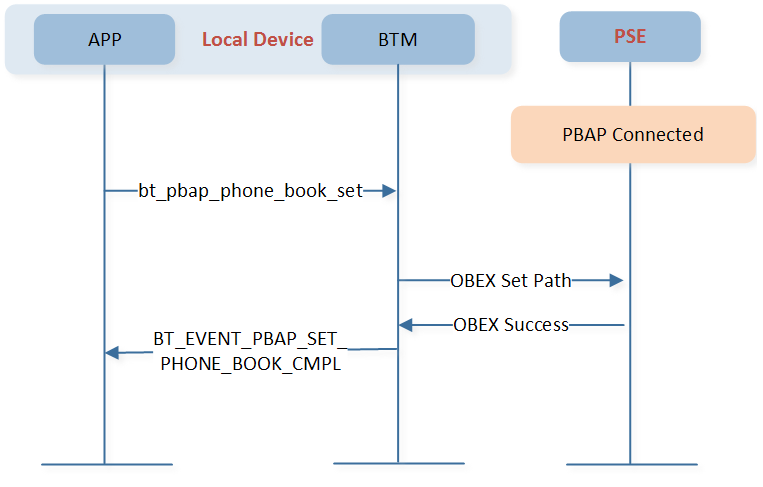
PBAP Phone Book Setting Flow
PBAP Pull Caller ID Name
The flow of the pbap pull_caller_id_name command is to pull caller identifier name.
pbap pull_caller_id_name [caller_id]
User can input the command above to pull caller identifier name. The parameter in the command is the caller identifier.
Information |
Description |
---|---|
Command |
pbap pull_caller_id_name |
Parameters |
Phone Number |
Usage |
Get name with phone number. |
Example |
pbap pull_caller_id_name 13512345678 |
The reference API can be found in bt_pbap_vcard_listing_by_number_pull()
, and the PBAP pull caller identifier name flow is shown below.
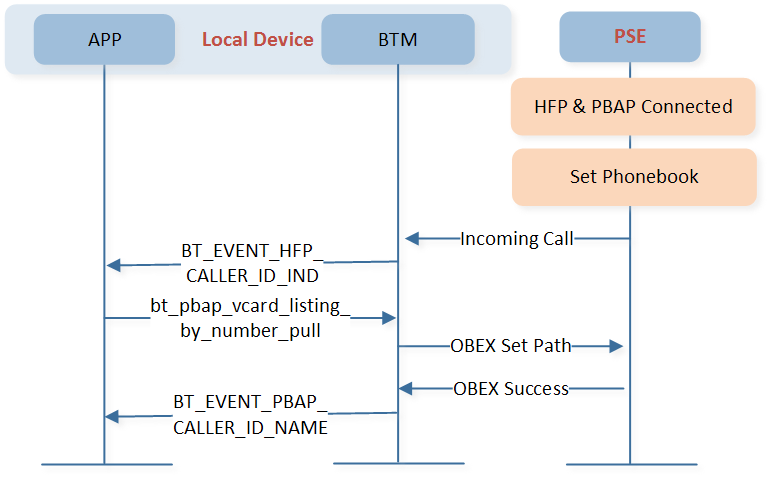
PBAP Pull Caller ID Name Flow