BR/EDR MAP
The Message Access Profile defines the features and procedures that shall be used by devices that exchange message objects. It is based on a Client-Server interaction model where the client initiates the transactions. The purpose of this document is to give an overview of the BR/EDR MAP demo application. More knowledge about MAP Profile can be found in MAP. The MAP demo project gives a simple example on how to use MAP. This project implements MAP MCE role.
Requirements
The sample supports the following development kits:
Hardware Platforms |
Board Name |
Build Target |
---|---|---|
RTL87x3E EVB |
|
|
RTL87x3E EVB |
|
|
RTL87x3D HDK |
RTL87x3D EVB |
|
Note
To purchase EVB, please visit https://www.realmcu.com/en/Home/Shop.
This sample project can be found under board\evb\bt_map_demo
in SDK folder structure. Developers can choose the project according to the Board Name
and choose the Build Target
according to the flash map.
When built for an xxx_4M_xxx
build target, the sample is configured to build and run with a 4M flash map.
When built for an xxx_8M_xxx
build target, the sample is configured to build and run with an 8M flash map.
When built for an xxx_16M_xxx
build target, the sample is configured to build and run with a 16M flash map.
The sample also requires a phone which supports MAP.
To quickly set up the development environment, please refer to the detailed instructions provided in Quick Start.
Configurations
Building and Downloading
Take the project rtl87x3e_map_demo.uvprojx
and target bt_map_demo_4M_bank0
as an example, to build and run the sample with Keil development environment, follow the steps listed below:
Open
rtl87x3e_map_demo.uvprojx
.Choose the build target
bt_map_demo_4M_bank0
.
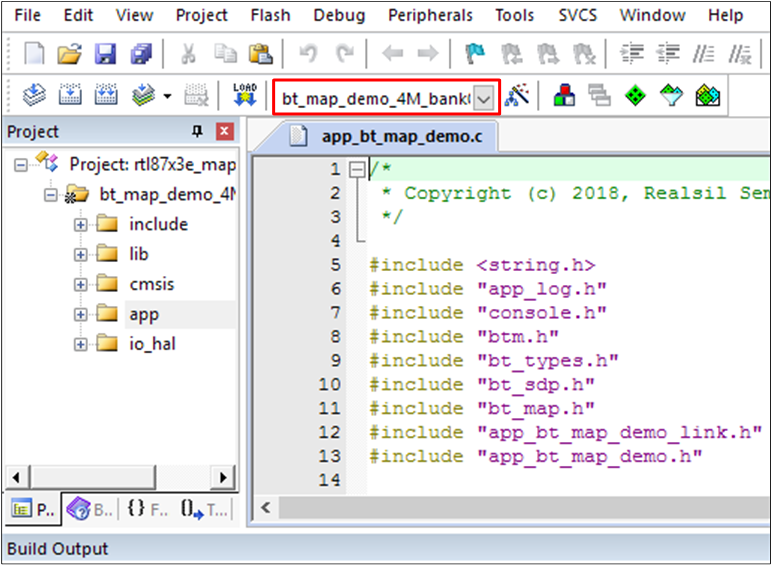
MAP Demo Project Target
Build the target. After a successful build, the APP bin file
bt_map_demo_bank0_MP-v0.0.0.0-xxx.bin
will be generated in the directorybin\rtl87x3e\flash_4M_dualbank\bank0
.
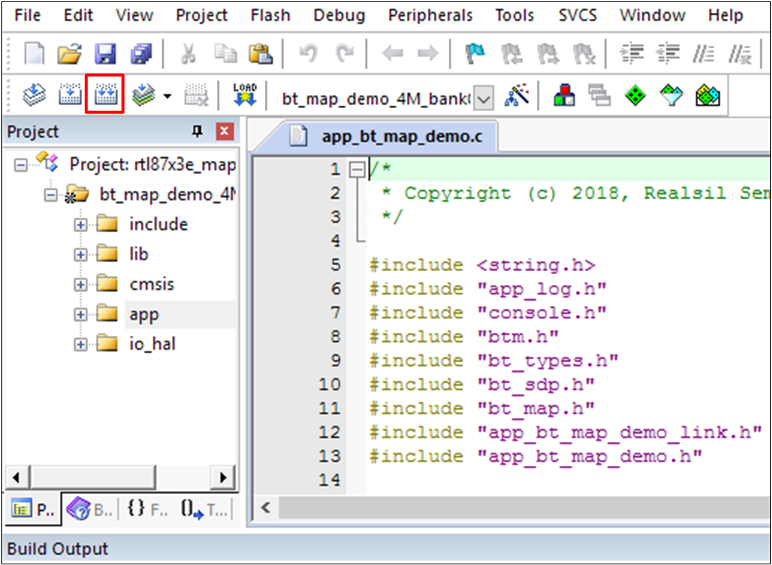
MAP Demo Project Build
Download APP bin into EVB board.
Press the reset button on the EVB board and it will be connectable.
Experimental Verification
Some basic functions of the MAP demo application are demonstrated below. To implement some complex functions, the user needs to refer to the manuals and source codes provided by SDK for development. Please use Debug Analyzer to get the log.
One evolution board shall be prepared, and the MAP demo application shall be downloaded.
This test requires the phone’s Bluetooth to be turned on.
Check the phone’s Bluetooth address.
Then connect to the phone through map connect.
Note
As the MAP demo application is MCE, it must initiate the connection to the phone.
UART Command to Input |
Output of UART Response |
Description |
---|---|---|
map connect 0x31 0x82 0x25 0x2B 0x35 0x48 |
MAP Connected! |
Connect MAP with remote device. |
Input the command map get_folder_listing to get the folder listing of the phone.
UART Command to Input |
Output of UART Response |
Description |
---|---|---|
map get_folder_listing |
MAP Get Folder Listing Success! |
MAP get phone’s message folder listing. |
Input the command map get_message_listing to get the message listing of the phone folder.
UART Command to Input |
Output of UART Response |
Description |
---|---|---|
map get_msg_listing |
MAP Get Message Listing Success! |
MAP get phone’s message listing in current folder. |
The user can input more corresponding commands referring to User Command.
UART Command to Input |
Output of UART Response |
Description |
---|---|---|
map mns_on |
MAP Message Notification ON! |
MAP message notification service On. |
map mns_off |
MAP Message Notification OFF! |
MAP message notification service Off. |
Code Overview
Source Code Directory
Project directory:
sdk\board\evb\bt_map_demo
.Project source code directory:
sdk\src\sample\bt_map_demo
.
Source files in the sample project are currently categorized into several groups as below.
└── Project: bt_map_demo_16M_bank0
├── include ROM UUID header files. Users do not need to modify it.
├── Lib Includes all binary symbol files that user application is built on.
├── cmsis The cmsis source code. Users do not need to modify it.
└── APP The application source code.
├── console_uart.c
├── app_bt_map_demo.c
├── app_bt_map_demo_console.c
├── app_bt_map_demo_gap.c
├── app_bt_map_demo_link.c
├── app_bt_map_demo_main.c
├── app_console_msg.c
├── app_dlps.c
├── app_io_msg.c
Initialization
Main function is invoked when the application is powered on or the chip resets and performs the following initialization functions.
int main(void)
{
board_init();
driver_init();
task_init();
bt_mgr_init();
app_bt_gap_init();
app_map_demo_init();
app_map_demo_gap_init();
app_map_demo_cmd_register();
os_sched_start();
return 0;
}
Profiles initialization flow:
bt_mgr_init()
- Contains Bluetooth Manager initialization.bt_map_init()
- Initialize MAP Profile.
Profile Message Callback
APP can register different callback functions to handle different Profiles.
void app_map_demo_init(void)
{
...
bt_mgr_cback_register(app_map_demo_cback);
}
User Command
This section provides a detailed description of the usage of user commands for the MAP demo application. The MAP demo application requires inputting commands through the Data UART in the host terminal to perform interaction. This allows various actions to be executed and the application to be controlled.
Connect
The flow of the map connect command is to connect MAP with a remote device. The user can input the command below to connect MAP. The address in the command is the remote Bluetooth device.
map connect [remote_device_bluetooth_address]
The console will print MAP Connected! to inform the user that MAP is connected.
Information |
Description |
---|---|
Command |
map connect [bd_addr] |
Usage |
Connect MAP with remote device. |
Example |
map connect 0x11 0x22 0x33 0x44 0x55 0x66 |
The reference API can be found in bt_map_mas_connect_over_rfc_req()
and bt_map_mas_connect_over_l2c_req()
.
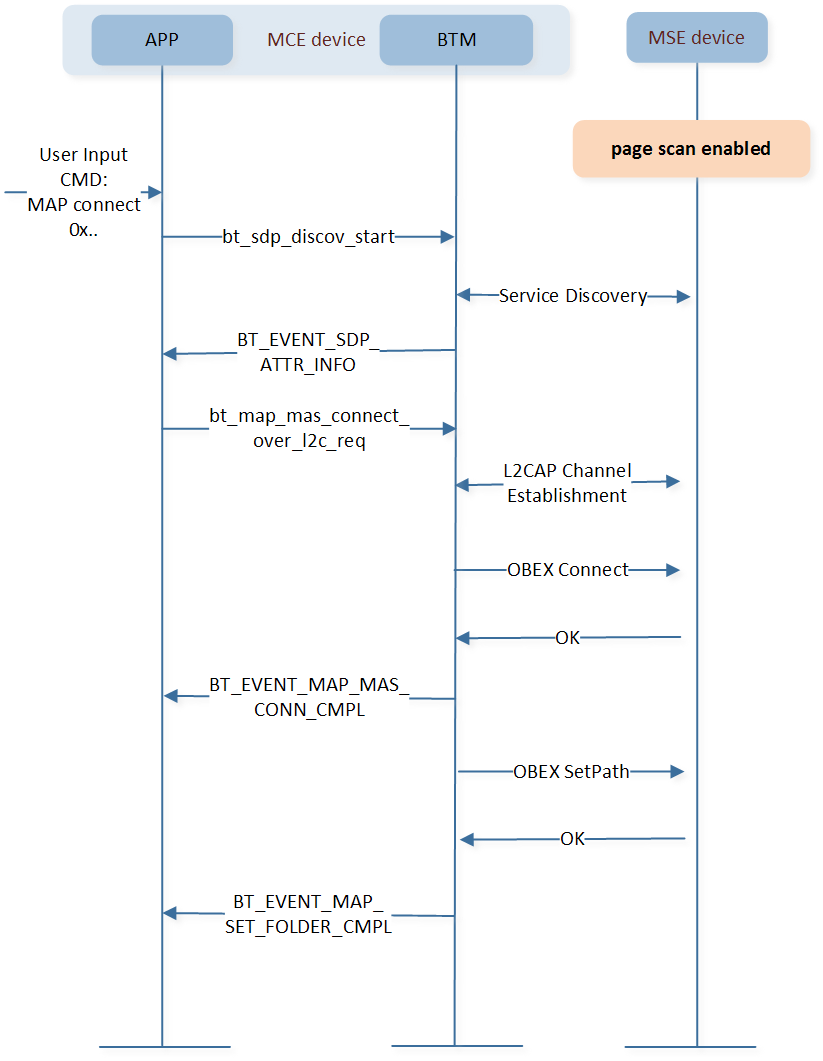
MAP Demo Connect Flow
Disconnect
The flow of the map disconnect command is to disconnect MAP with a remote device. The user can input the command bellow to disconnect MAP.
map disconnect
The console will print MAP Disconnected! to inform the user that MAP is disconnected.
Information |
Description |
---|---|
Command |
map disconnect |
Usage |
Disconnect MAP from the remote device. |
The reference API can be found in bt_map_mas_disconnect_req()
.
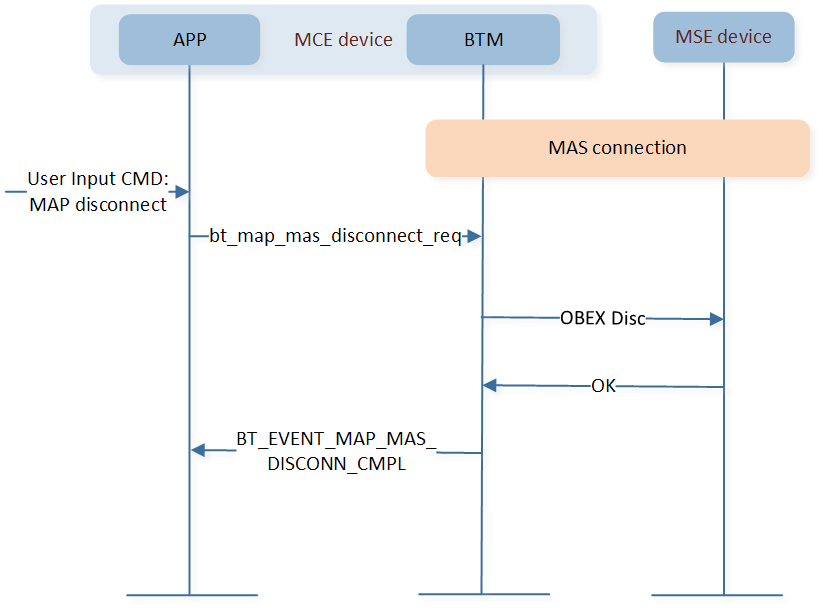
MAP Disconnect Flow
Set Message Notification Service
The flow of the map mns_on command is to turn on the message notification service. The user can input the command below to turn on the message notification service.
map mns_on
The console will print MAP Message Notification ON! to inform that the service is available.
Information |
Description |
---|---|
Command |
map mns_on |
Usage |
Turn on the message notification service. |
The reference API can be found in bt_map_mas_msg_notification_set()
.
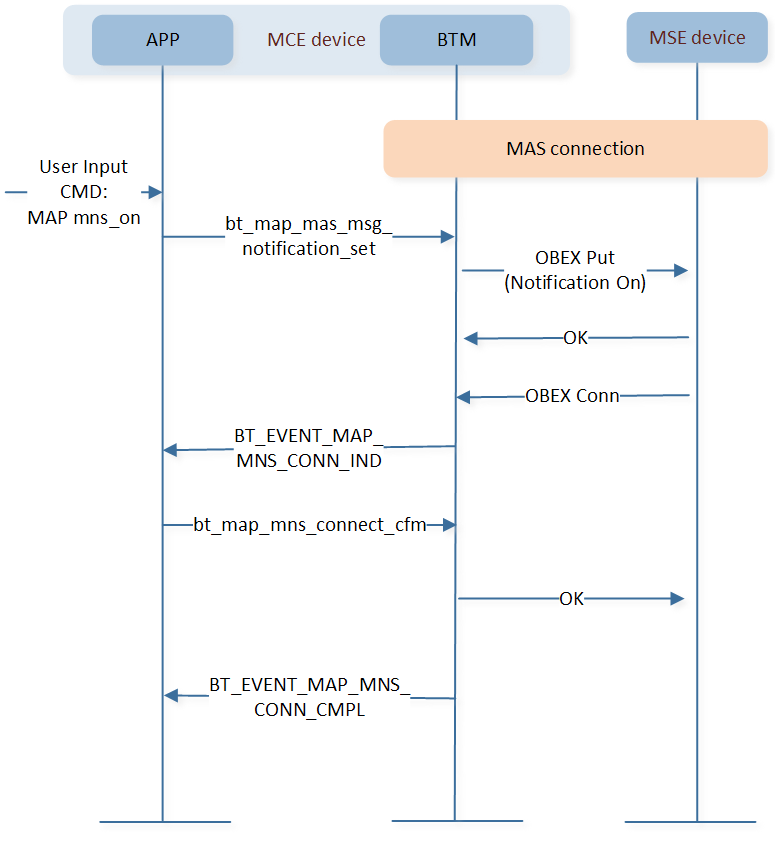
MAP Message Notification Service Enable Flow
The flow of the map mns_off command is to turn off the message notification service. The user can input the command below to turn off the message notification service.
map mns_off
The console will print MAP Message Notification OFF! to inform that the service is unavailable.
Information |
Description |
---|---|
Command |
map mns_off |
Usage |
Turn off the message notification service. |
The reference API can be found in bt_map_mas_msg_notification_set()
.
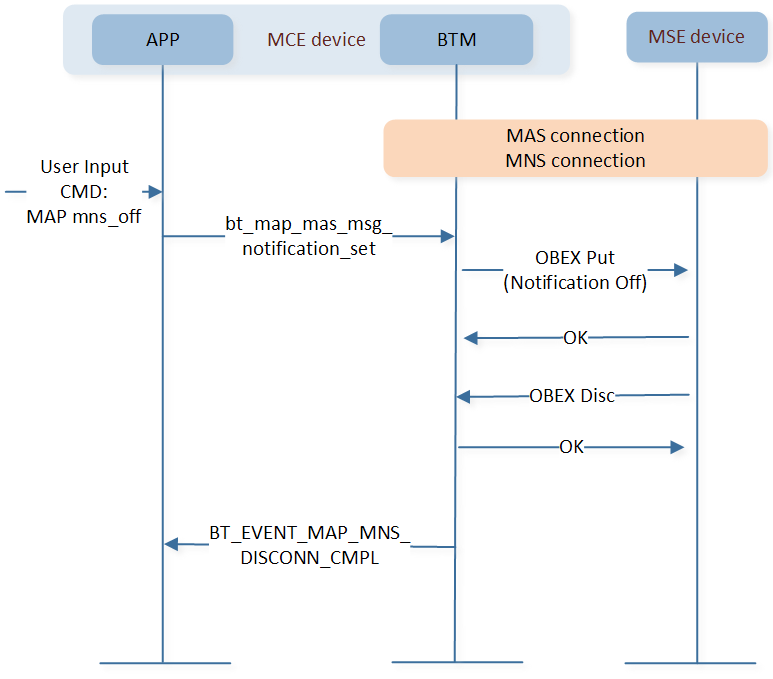
MAP Message Notification Service Disable Flow
Set Folder
The flow of the map set_folder command is to navigate the folders of MSE. The user can input the command below to set the folder of MSE.
map set_folder [t_bt_map_folder]
Information |
Description |
---|---|
Command |
map set_folder [t_bt_map_folder] |
Usage |
Navigate the folders of MSE. |
The reference API can be found in bt_map_mas_folder_set()
.
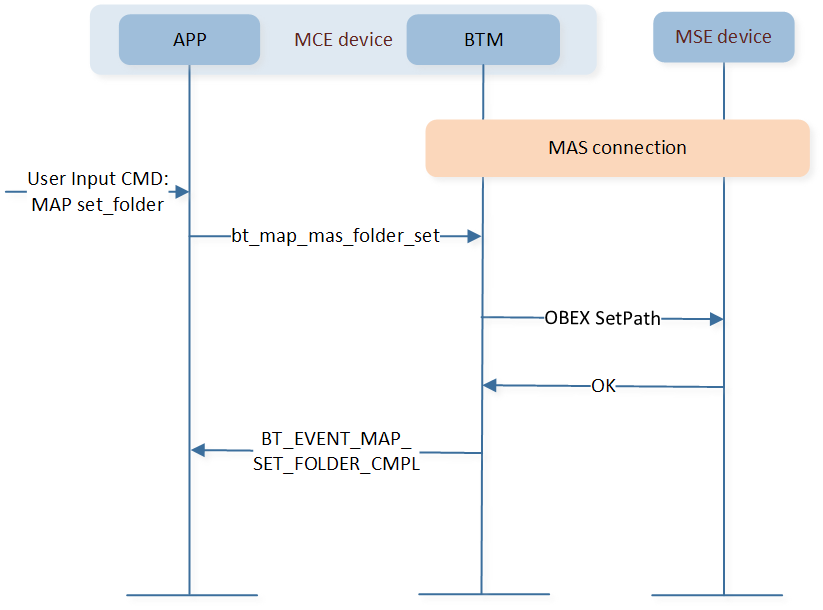
MAP Set Folder Flow
Get Folder Listing
The flow of the map get_folder_listing command is to get the folder listing of MSE. The user can input the command below to get the folder listing of MSE.
map get_folder_listing
The console will print MAP Get Folder Listing Success! to inform the user that the folder listing is obtained.
Information |
Description |
---|---|
Command |
map get_folder_listing |
Usage |
Get the folder listing of MSE. |
The reference API can be found in bt_map_mas_folder_listing_get()
.
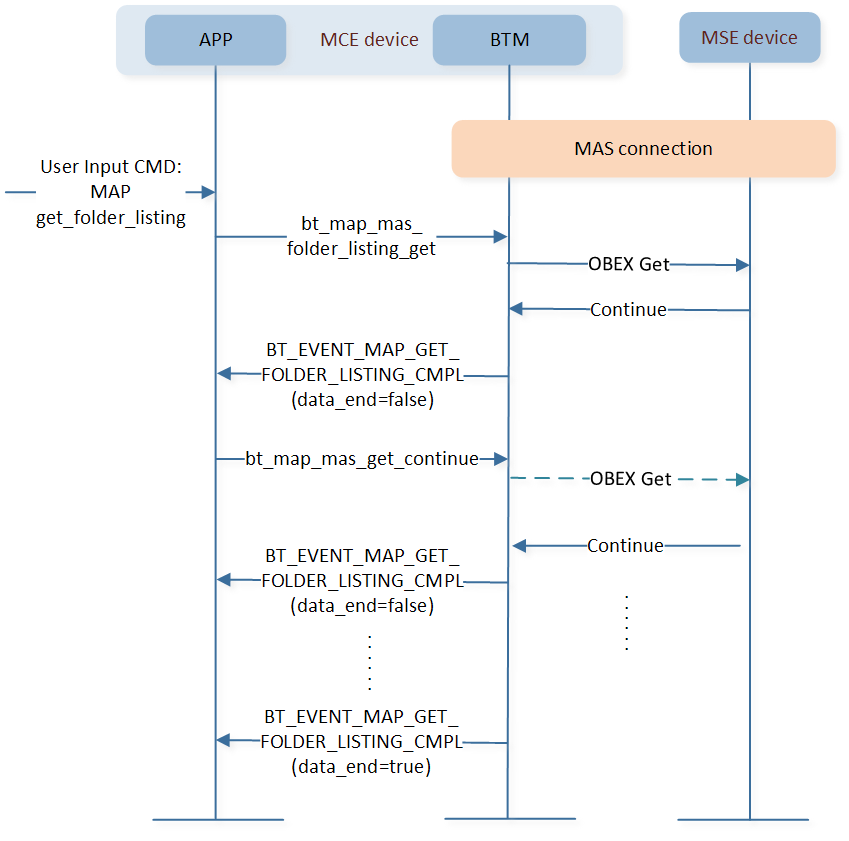
MAP Get Folder Listing Flow
Get Message Listing
The flow of the map get_msg_listing command is to get the message listing of MSE. The user can input the command below to get the message listing of MSE.
map get_msg_listing
The console will print MAP Get Message Listing Success! to inform the user that the message listing is retrieved.
Information |
Description |
---|---|
Command |
map get_msg_listing |
Usage |
Get the message listing of the MSE folder. |
The reference API can be found in bt_map_mas_msg_listing_get()
.
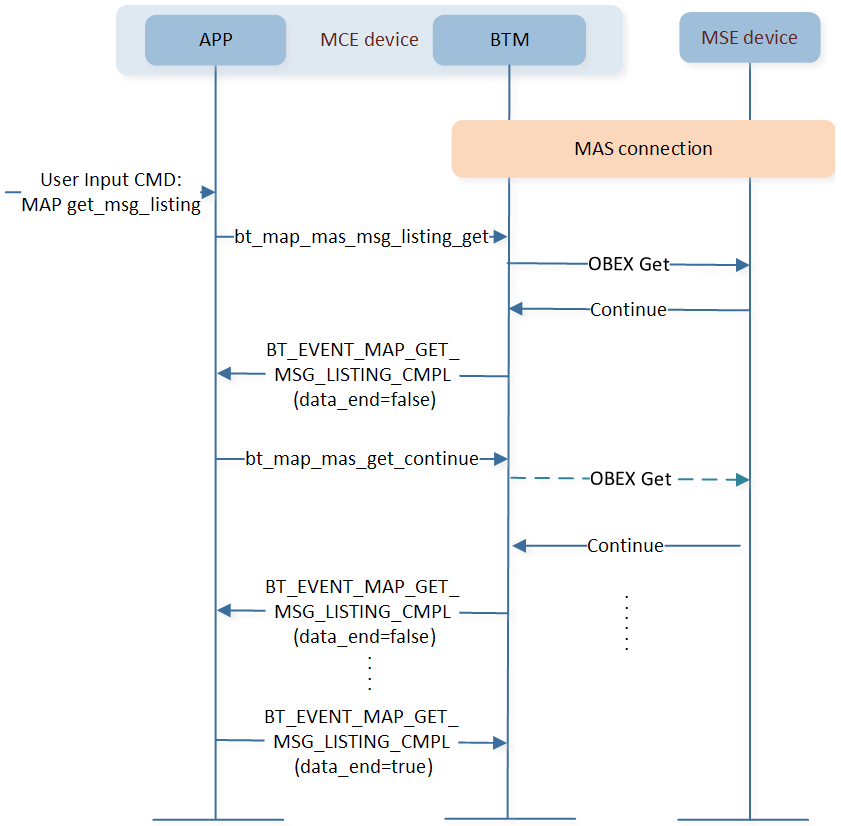
MAP Get Message Listing Flow