BR/EDR PAN
The purpose of this document is to provide an overview of the BR/EDR demo application. The BR/EDR PAN demo project offers a simple example on how to use PAN. This project implements PAN User role for PAN. For this profile, three roles are discussed: NAP, GN and PANU. More details are depicted in PAN Role section.
This demo takes lwIP as TCP/IP stack. TCP/IP acts as an application layer on BR/EDR PAN profile. Stack overview diagram is following:
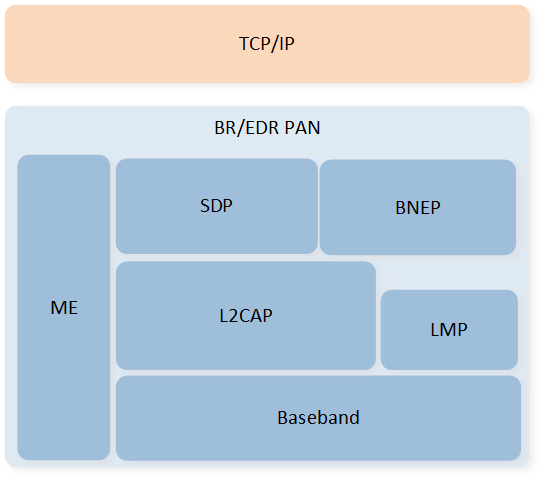
Stack Overview
Requirements
The sample supports the following development kits:
Hardware Platforms |
Board Name |
Build Target |
---|---|---|
RTL87x3E EVB |
|
|
RTL87x3D HDK |
RTL87x3D EVB |
|
Note
To purchase EVB, please visit https://www.realmcu.com/en/Home/Shop.
This sample project can be found under board\evb\bt_pan_demo
in SDK folder structure. Developers can choose the project according to the Board Name and choose the Build Target according to the flash map.
When built for an xxx_4M_xxx
build target, the sample is configured to build and run with a 4M flash map.
When built for an xxx_8M_xxx
build target, the sample is configured to build and run with an 8M flash map.
When built for an xxx_16M_xxx
build target, the sample is configured to build and run with a 16M flash map.
To quickly set up the development environment, please refer to the detailed instructions provided in Quick Start.
Note
You can directly reference Quick Start to avoid repetitive descriptions.
The TX and RX PINMUX of console is defined in app_bt_pan_demo_main.c
.
It is important to note that the pins need to be wired in reverse, which means that the TX of
console should be connected to P3_0
, and the RX should be connected to
P3_1
in order to establish a connection with the serial port.
#define BT_PAN_DEMO_UART_TX P3_1
#define BT_PAN_DEMO_UART_RX P3_0
Configurations
APP configurable functions are defined in app_flags.h
.
The user can easily change the value of the macro definition to switch the function.
Building and Downloading
Note
This section introduces sample compilation methods (such as Keil, GCC, etc.) and how to download them to EVB (J-Link, MPPG Tool, etc.).
Take the project rtl87x3e_bt_pan_demo.uvprojx
and target bt_pan_demo_4M_bank0
as an example, to build and run the sample with Keil development environment, follow the steps listed below:
Open
rtl87x3e_bt_pan_demo.uvprojx
.Choose the build target
bt_pan_demo_4M_bank0
.Choose Build Target
Building the target.
Building
Download APP bin into EVB board.
Note
Quick Start includes sections like Generating APP Image and Images Download. If they are consistent, please directly reference them.
Experimental Verification
Note
PAN profile uses lwIP as it’s TCP/IP layer Implementation. Further information can refer to lwIP. This section introduces the console commands and test procedure during the experimental process.
PAN Connect
User can input command pan connect [remote address]
command to connect PAN with remote device.
The console will print PAN Connected!
informing user that PAN is connected successfully.
Information |
Description |
---|---|
Command |
|
Parameters |
Remote Bluetooth Address |
Usage |
Connect PAN with Remote Device. |
Example |
|
The reference API can be found in bt_pan_connect_req
.
PAN connect flow is shown as follows.
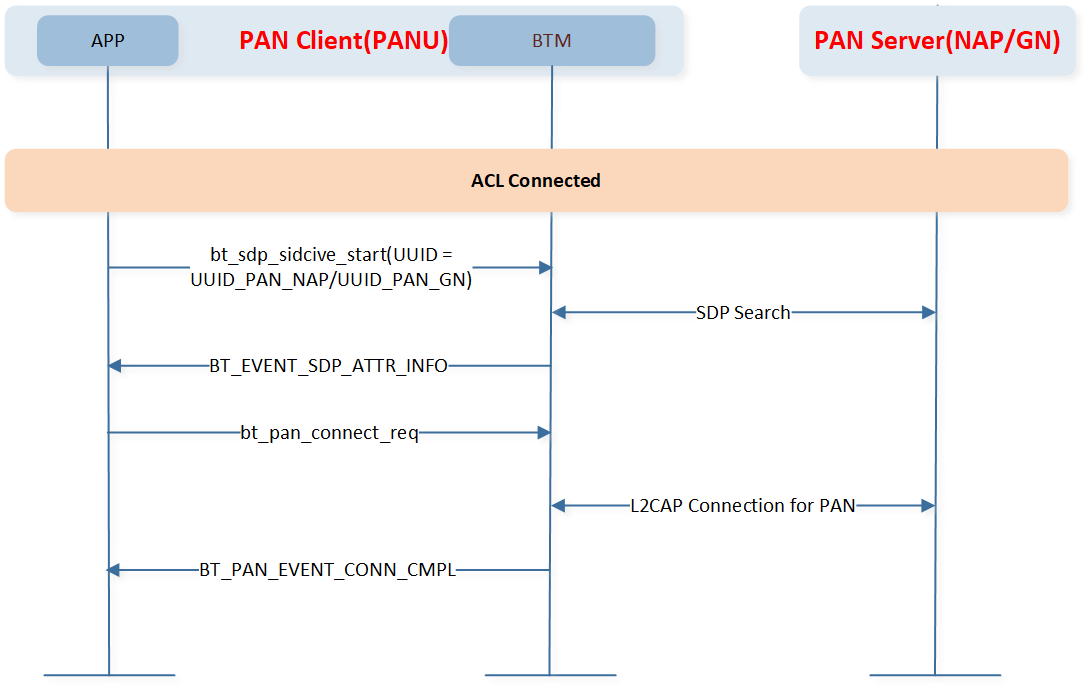
PAN Connect
PAN Disconnect
User can input command pan disconnect
to disconnect PAN with remote device.
The console will print PAN Disconnected!
informing user that PAN is disconnected.
Information |
Description |
---|---|
Command |
pan disconnect |
Usage |
Disconnect PAN with remote device. |
The reference API can be found in bt_pan_disconnect_req
.
PAN disconnect flow is shown as follows.
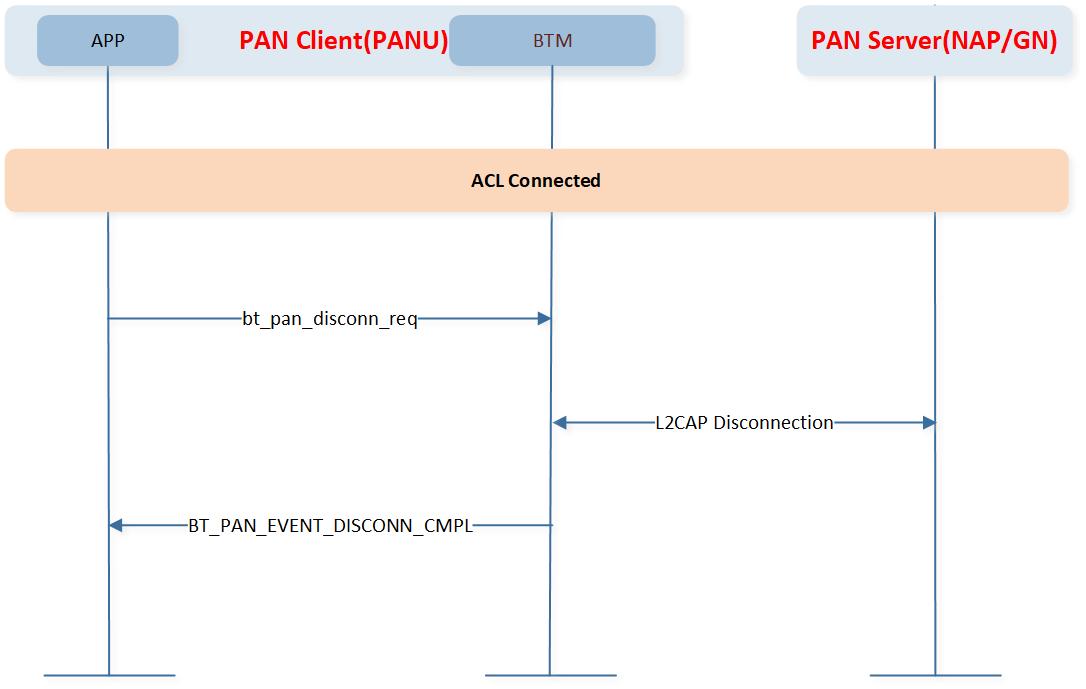
PAN Disconnect
Access Point Setup
It is convenient for testing to set up servers on PC. In order to access PC, it is necessary to take a phone as a switch (or router):
Connect and pair to phone by Bluetooth & other devices.
Phone As Switch Step1
Get into Devices and printers sub-page. Choose the phone device you paired and then click Access point.
Phone As Switch Step2
Check the connecting status in Bluetooth of phone. And in Paired Bluetooth device, Internet connection sharing should be enabled.
Phone As Switch Step3
PING
PING program is a basic diagnosis on IP layer. It is recommanded to run it preceding other test or demo. Ping.c
in group pan_test
is the source file and the main function of it is calling ping_init
and ping_send_now
.
User can input pan ping [server_name]
command to trigger the ping program where server_name
should not be a host name than an IP address. Then user can check it by ping: recv
in log.
TCP
In the project, sockets.c
in pan_test group is the source code for testing TCP client/server. Sockets.c
uses sockets API to act as client and server.
User can test client by procedures below:
PC connect to phone’s AP depicted in Access Point Setup.
Input
pan connect [phone_bd_addr]
to connect to phone.Start a TCP server on PC.
Input
pan sockets client [<]ip_addr] [port]
to start a the TCP client.
User can test server by procedures below:
PC connect to phone’s AP depicted in Access Point Setup.
Input
pan connect [phone_bd_addr]
to connect to phone.Input
pan sockets server [port]
to start a the TCP server which is waiting for a client connection now.Start a TCP client on PC.
HTTP
In the project, http.c
in pan_test group is the source code for testing HTTP client.
For testing convenience, users can set up a HTTP server on PC. It is described in lwIP HTTP. For BR/EDR PAN profile, phone should act as a switch and access point for PC described above. After connected, user should change the uri
and ip_str
according to the resource wanted.
If accessing website from WAN, user should replace httpc_get_file
with httpc_get_file_dns
to use domain name. These two APIs are the core function in HTTP test.
The detailed procedures for HTTP client test:
(Optional) Setup local HTTP server and take a phose as switch for PC.
Input
pan connect [phone_bd_addr]
establish a connection on PAN.Input
pan http [ip_addr] [port] [uri]
. Then check the received content by callback registered inhttpc_get_file
. For this demo, it isown_recv
. Ellisys Injection is also recommanded.
HTTPS
HTTPS test has its own group pan_test\https
:
Https_demo.c
is the main source file for HTTPS client.Mbedtls_port.c
is the Mbed TLS porting file. It provides network access for Mbed TLS. There is ambedtls_lib
project for the whole source code.
Server’s setup is depicted in lwIP HTTPS. Note that the certificate should correspond to the website that user accesses.
In function https_client
, certificates should be add to ca_cert
by mbedtls_x509_crt_parse_der
or mbedtls_x509_crt_parse
. Modify the parameters of mbedtls_net_connect to connect to expected website and resources, such as WEB_SERVER
and WEB_PORT
. WEB_URL
in GET_REQUEST
also needs to be set.
After preparation above, inputing pan connect
and pan https
can start this test. If successful, read xxx bytes:
and the following logs will show the HTTPS content:
[APP] [mbedtls]read 230 bytes:
[APP] [mbedtls]HTTP/1.1 200 OK
Date: Fri, 20 Sep 2024 08:00:30 GMT
Content-Type: application/json
Content-Length: 244
Connection: keep-alive
Server: gunicorn/19.9.0
Access-Control-Allow-Origin: *
Access-Control-Allow-Credentials: true
Note
SSL/TLS (for HTTPS) takes over 50KB heap memory which out of a simple demo, so there is a set of dedicated APP Config bin in
bin\rtl87x3e\app_demo_bin\mdk\bt_pan_demo
. This bin set disable LE stack to give about 10KB extra heap.Please equip with PSRAM or other external memory for actual product development.
Code Overview
Note
This chapter provides a brief analysis of important code.
The BR/EDR PAN demo application overview will be introduced according to the following parts:
The directories of the project and source code files will be introduced in chapter Source Code Directory.
The main function will be introduced in chapter Source Code Overview.
Source Code Directory
Project directory:
sdk\board\evb\bt_pan_demo
.Source code directory:
sdk\src\sample\bt_pan_demo
.
Source files in the sample project are currently categorized into several groups as below.
└── Project: bt_pan_demo
├── include
└── cmsis includes startup code
├── startup_rtl87x3e.c
└── system_rtl87x3.c
└── Lib includes all binary symbol files that user application is built on
├── lwip.lib
└── mbedtls.lib
└── APP includes the find my user application implementation
├── console_uart.c
├── app_console_msg.c
├── app_dlps.c
├── app_io_msg.c
├── app_bt_pan_demo_console.c
├── app_bt_pan_demo.c
├── app_bt_pan_demo_gap.c
├── app_bt_pan_demo_link.c
└── app_bt_pan_demo_main.c
└── pan_test includes test source files
├── http.c
├── sockets.c
└── ping.c
└── pan_test/https includes test source files for HTTPS client
├── https_demo.c
└── mbedtls_port.c
Source Code Overview
The main purpose of this chapter is to help APP developers familiarize with the development process related to BR/EDR HID demo.
Initialization
main()
is invoked when the application is powered on or the chip is reset, and it performs the following initialization functions:
int main(void)
{
board_init();
driver_init();
task_init();
framework_init();
app_bt_gap_init();
app_bt_profile_init();
app_bt_pan_demo_gap_init();
app_bt_pan_demo_cmd_register();
os_sched_start();
return 0;
}
Please refer to PAN demo source code for initialization function declaration.
More information on Profiles initialization can be found in PAN Init.
PAN Callback Message
APP can register PAN callback function to handle different PAN events.
void app_bt_pan_demo_init(void)
{
...
bt_pan_cback_register(app_bt_pan_demo_cback);
}
Interface with lwIP
lwIP is initialized in app_bt_pan_demo_init
with tcpip_init
and bnepif_init
. The former is TCP/IP stack’s main initialization and the latter initializes bnep netif.
lwIP acts as application layer for BR/EDR PAN. In turn, BR/EDR PAN acts as the MAC and PHY for it which can also be regarded as netif. In Bluetooth, profiles are application interoperability documents rather than a protocol layer, so that the netif, bnepif
, is named after bnep.
There lists three other interface point:
Under
case BT_PAN_EVENT_CONN_CMPL
,bnepif_netif_up
enable bnepif netif, whilebnepif_netif_down
disable bnepif netif undercase BT_PAN_EVENT_DISCONN_CMPL
.bnepif_low_level_input
receives packets from BR/EDR PAN to lwIP stack.bt_pan_send
sends packets from lwIP, which registered bybnepif_init
.