Img
The image widget is the basic widget used to display images. Image widgets support moving, zooming, rotating, etc.
Usage
Create Widget
It is possible to use gui_img_create_from_mem()
to create an image widget from memory, or use gui_img_create_from_fs()
to create an image widget from a file. Alternatively, gui_img_create_from_ftl()
can be used to create an image widget from ftl.
If the width or height of the image widget is set to 0, the widget’s size will be set according to the size of the image source automatically.
Update Location
If it is necessary to update the location of an image widget, use gui_img_set_location()
to relocate.
Set Attribute
It is possible to use gui_img_set_attribute()
to set the attribute of an image widget, replace it with a new image, and set a new coordinate.
Get Height/Width
If you want to get the height/width of image widget, do so with gui_img_get_height()
or gui_img_get_width()
.
Refresh
Refresh the image size using gui_img_refresh_size()
.
Blend Mode
Set the image’s blend mode using gui_img_set_mode()
.
Translation
Use gui_img_translate()
to move the image widget.
It can move an image widget to a new coordinate without changing the original coordinate in the widget’s attribute.
Rotation
Rotate the image widget around the center of the circle with this API gui_img_rotation()
.
Zoom
You can adjust the size of the image widget to fit your requirements by this API gui_img_scale()
.
Opacity
The opacity value of the image is adjustable, and it can be set using gui_img_set_opacity()
.
Animation
The gui_img_set_animate()
can be used to set the animation effects for the image widget.
Quality
The image’s quality can be set using gui_img_set_quality()
.
Screenshot
The gui_img_tree_convert_to_img()
can be used to save a fullscreen screenshot. The saved image will be in RGB format.
Example
#include "root_image_hongkong/ui_resource.h"
#include "gui_img.h"
#include "gui_text.h"
#include "draw_font.h"
char *tb1_text = "gui_img_create_from_mem";
void page_tb1(void *parent)
{
static char array1[50];
static char array2[50];
gui_set_font_mem_resourse(24, TEST_FONT24_DOT_BIN, TEST_FONT24_TABLE_BIN);
gui_img_t *img_test = gui_img_create_from_mem(parent, "test", SET_ON_BIN, 0, 0, 0, 0);
gui_text_t *text1 = gui_text_create(parent, "text1", 10, 100, 300, 30);
gui_text_set(text1, tb1_text, GUI_FONT_SRC_BMP, 0xffffffff, strlen(tb1_text), 24);
gui_text_mode_set(text1, LEFT);
gui_text_t *text2 = gui_text_create(parent, "text2", 10, 130, 330, 30);
gui_text_set(text2, tb1_text, GUI_FONT_SRC_BMP, 0xffffffff, strlen(tb1_text), 24);
gui_text_mode_set(text2, LEFT);
sprintf(array1, "gui_img_get_height %d", gui_img_get_height(img_test));
text2->utf_8 = array1;
text2->len = strlen(array1);
gui_text_t *text3 = gui_text_create(parent, "text3", 10, 160, 330, 30);
gui_text_set(text3, tb1_text, GUI_FONT_SRC_BMP, 0xffffffff, strlen(tb1_text), 24);
gui_text_mode_set(text3, LEFT);
sprintf(array2, "gui_img_get_width %d", gui_img_get_width(img_test));
text3->utf_8 = array2;
text3->len = strlen(array2);
}
void page_tb2(void *parent)
{
gui_set_font_mem_resourse(24, TEST_FONT24_DOT_BIN, TEST_FONT24_TABLE_BIN);
gui_img_t *img_test = gui_img_create_from_mem(parent, "test", SET_ON_BIN, 0, 0, 0, 0);
gui_img_set_location(img_test, 50, 50);
gui_text_t *text2 = gui_text_create(parent, "text2", 10, 100, 330, 24);
gui_text_set(text2, "gui_img_set_location", GUI_FONT_SRC_BMP, 0xffffffff, 20, 24);
gui_text_mode_set(text2, LEFT);
}
void page_tb3(void *parent)
{
gui_img_t *img_test = gui_img_create_from_mem(parent, "test", SET_ON_BIN, 0, 0, 0, 0);
gui_img_set_attribute(img_test, "test", SET_OFF_BIN, 20, 20);
gui_text_t *text3 = gui_text_create(parent, "text3", 10, 100, 330, 24);
gui_text_set(text3, "gui_img_set_attribute", GUI_FONT_SRC_BMP, 0xffffffff, 21, 24);
gui_text_mode_set(text3, LEFT);
}
void page_tb4(void *parent)
{
gui_set_font_mem_resourse(24, TEST_FONT24_DOT_BIN, TEST_FONT24_TABLE_BIN);
gui_img_t *img_test = gui_img_create_from_mem(parent, "test", SET_ON_BIN, 0, 0, 0, 0);
gui_img_scale(img_test, 0.5, 0.5);
gui_text_t *text4 = gui_text_create(parent, "text4", 10, 100, 330, 24);
gui_text_set(text4, "gui_img_scale", GUI_FONT_SRC_BMP, 0xffffffff, 13, 24);
gui_text_mode_set(text4, LEFT);
}
void page_tb5(void *parent)
{
gui_set_font_mem_resourse(24, TEST_FONT24_DOT_BIN, TEST_FONT24_TABLE_BIN);
gui_img_t *img_test = gui_img_create_from_mem(parent, "test", SET_ON_BIN, 0, 0, 0, 0);
gui_img_translate(img_test, 100, 100);
gui_text_t *text5 = gui_text_create(parent, "text5", 10, 100, 330, 24);
gui_text_set(text5, "gui_img_translate", GUI_FONT_SRC_BMP, 0xffffffff, 17, 24);
gui_text_mode_set(text5, LEFT);
}
void page_tb6(void *parent)
{
gui_set_font_mem_resourse(24, TEST_FONT24_DOT_BIN, TEST_FONT24_TABLE_BIN);
gui_img_t *img_test = gui_img_create_from_mem(parent, "test", SET_ON_BIN, 0, 0, 0, 0);
gui_img_rotation(img_test, 10, 0, 0);
gui_text_t *text6 = gui_text_create(parent, "text6", 10, 100, 330, 24);
gui_text_set(text6, "gui_img_rotation", GUI_FONT_SRC_BMP, 0xffffffff, 16, 24);
gui_text_mode_set(text6, LEFT);
}
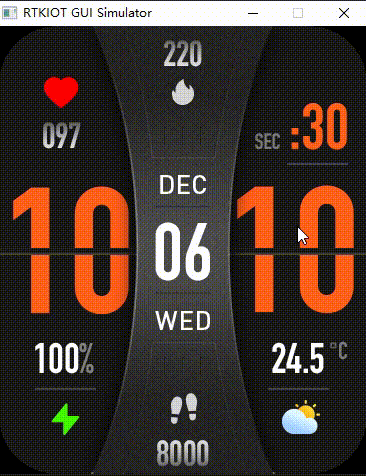
API
Functions
-
uint16_t gui_img_get_width(gui_img_t *_this)
load the image to read it’s width.
- Parameters:
_this – the image widget pointer.
- Returns:
uint16_t image’s width.
-
uint16_t gui_img_get_height(gui_img_t *_this)
load the image to read it’s hight.
- Parameters:
_this – the image widget pointer.
- Returns:
uint16_t image’s height.
-
void gui_img_refresh_size(gui_img_t *_this)
refresh the image size from src.
- Parameters:
_this – the image widget pointer.
-
void gui_img_set_location(gui_img_t *_this, uint16_t x, uint16_t y)
set the image’s location.
- Parameters:
_this – the image widget pointer.
x – the x coordinate.
y – the y coordinate.
-
void gui_img_set_mode(gui_img_t *_this, BLEND_MODE_TYPE mode)
set the image’s blend mode.
- Parameters:
_this – the image widget pointer.
mode – the enumeration value of the mode is BLEND_MODE_TYPE.
-
void gui_img_set_attribute(gui_img_t *_this, const char *name, void *addr, int16_t x, int16_t y)
set x,y and file path.
- Parameters:
_this – image widget.
name – change widget name.
addr – change picture address.
x – X-axis coordinate.
y – Y-axis coordinate.
-
void gui_img_rotation(gui_img_t *_this, float degrees, float c_x, float c_y)
rotate the image around the center of the circle.
- Parameters:
_this – the image widget pointer.
degrees – clockwise rotation absolute angle.
c_x – the X-axis coordinates of the center of the circle.
c_y – the Y-axis coordinates of the center of the circle.
-
void gui_img_scale(gui_img_t *_this, float scale_x, float scale_y)
change the size of the image, take (0, 0) as the zoom center.
- Parameters:
_this – the image widget pointer.
scale_x – scale in the x direction.
scale_y – scale in the y direction.
-
void gui_img_translate(gui_img_t *_this, float t_x, float t_y)
move image.
- Parameters:
_this – the image widget pointer.
t_x – new X-axis coordinate.
t_y – new Y-axis coordinate.
-
void gui_img_skew_x(gui_img_t *_this, float degrees)
skew image on X-axis.
- Parameters:
_this – the image widget pointer.
degrees – skew angle.
-
void gui_img_skew_y(gui_img_t *_this, float degrees)
skew image on Y-axis.
- Parameters:
_this – the image widget pointer.
degrees – skew angle.
-
void gui_img_set_opacity(gui_img_t *_this, unsigned char opacity_value)
add opacity value to the image.
- Parameters:
_this – the image widget pointer.
opacity_value – The opacity value ranges from 0 to 255, default 255.
-
gui_img_t *gui_img_create_from_mem(void *parent, const char *name, void *addr, int16_t x, int16_t y, int16_t w, int16_t h)
creat an image widget from memory address.
Note
creat an image widget and set attribute.
- Parameters:
parent – the father widget it nested in.
name – widget name.
addr – bin file address.
x – the X-axis coordinate of the widget.
y – the Y-axis coordinate of the widget.
w – the width of the widget.
h – the hight of the widget.
- Returns:
return the widget object pointer.
-
gui_img_t *gui_img_create_from_ftl(void *parent, const char *name, void *ftl, int16_t x, int16_t y, int16_t w, int16_t h)
creat an image widget from memory address.
Note
creat an image widget and set attribute.
- Parameters:
parent – the father widget it nested in.
name – widget name.
ftl – not xip address, use ftl address.
x – the X-axis coordinate of the widget.
y – the Y-axis coordinate of the widget.
w – the width of the widget.
h – the hight of the widget.
- Returns:
return the widget object pointer.
-
gui_img_t *gui_img_create_from_fs(void *parent, const char *name, void *file, int16_t x, int16_t y, int16_t w, int16_t h)
creat an image widget from filesystem.
- Parameters:
parent – the father widget it nested in.
name – image widget name.
file – image file path.
x – the X-axis coordinate of the widget.
y – the Y-axis coordinate of the widget.
w – the width of the widget.
h – the hight of the widget.
- Returns:
gui_img_t*.
-
void gui_img_set_animate(gui_img_t *_this, uint32_t dur, int repeat_count, void *callback, void *p)
set animate.
- Parameters:
_this – pointer.
dur – animation time cost in ms.
repeat_count – rounds to repeat.
callback – every frame callback.
p – callback’s parameter.
-
void gui_img_set_quality(gui_img_t *_this, bool high_quality)
set the image’s quality.
- Parameters:
_this – the image widget pointer.
high_quality – image drawn in high quality or not.
-
void gui_img_tree_convert_to_img(gui_obj_t *obj, gui_matrix_t *matrix, uint8_t *shot_buf)
convert a tree to a image data.
- Parameters:
obj – tree root.
matrix – null if no need to transform.
-
float gui_img_get_transform_scale_x(gui_img_t *img)
get the transform scale in the X direction for a GUI image.
- Parameters:
img – pointer to the GUI image object.
- Returns:
the scale in the X direction.
-
float gui_img_get_transform_scale_y(gui_img_t *img)
get the transform scale in the Y direction for a GUI image.
- Parameters:
img – pointer to the GUI image object.
- Returns:
the scale in the Y direction.
-
float gui_img_get_transform_degrees(gui_img_t *img)
get the rotation angle in degrees for a GUI image.
- Parameters:
img – pointer to the GUI image object.
- Returns:
the rotation angle in degrees.
-
float gui_img_get_transform_c_x(gui_img_t *img)
get the center X coordinate for rotate of a GUI image.
- Parameters:
img – pointer to the GUI image object.
- Returns:
the center X coordinate for transformations.
-
float gui_img_get_transform_c_y(gui_img_t *img)
get the center Y coordinate for rotate of a GUI image.
- Parameters:
img – pointer to the GUI image object.
- Returns:
the center Y coordinate for transformations.
-
float gui_img_get_transform_t_x(gui_img_t *img)
get the translation in the X direction for a GUI image.
- Parameters:
img – pointer to the GUI image object.
- Returns:
the translation in the X direction.
-
float gui_img_get_transform_t_y(gui_img_t *img)
get the translation in the Y direction for a GUI image.
- Parameters:
img – pointer to the GUI image object.
- Returns:
the translation in the Y direction.
-
void gui_img_set_image_data(gui_img_t *widget, const uint8_t *image_data_pointer)
Sets the image data for a specified image widget.
This function assigns the given image data to the specified image widget. The image data might correspond to various formats, and the format should be compatible with the handling of
gui_img_t
.- Parameters:
widget – The pointer to the image widget (
gui_img_t
) for which the image data is to be set.image_data_pointer – The pointer to the image data to be set to the widget. The data should persist as long as the widget needs it or until it is explicitly updated.
-
const uint8_t *gui_img_get_image_data(gui_img_t *widget)
Gets the image data from a specified image widget.
This function returns the current image data that is set in the specified image widget.
- Parameters:
widget – The pointer to the image widget (
gui_img_t
) from which the image data should be retrieved.- Returns:
A pointer to the image data currently set in the widget. If no image data is set, the result may be
NULL
.
-
struct gui_img_transform_t
image widget structure
Public Members
-
float degrees
float gui_img_get_transform_degrees(gui_img_t *img);
-
float c_x
center of image x; float gui_img_get_transform_c_x(gui_img_t *img);
-
float c_y
center of image y; float gui_img_get_transform_c_y(gui_img_t *img);
-
float scale_x
float gui_img_get_transform_scale_x(gui_img_t *img);
-
float scale_y
float gui_img_get_transform_scale_y(gui_img_t *img);
-
float t_x
translate of screen x; float gui_img_get_transform_t_x(gui_img_t *img);
-
float t_y
translate of screen y; float gui_img_get_transform_t_y(gui_img_t *img);
-
float t_x_old
-
float t_y_old
-
float degrees
-
struct gui_img_t
Public Members
-
gui_obj_t base
-
draw_img_t *draw_img
-
gui_img_transform_t *transform
-
void *data
-
void *filename
-
void *ftl
- union gui_img_t
-
gui_animate_t *animate
-
uint32_t opacity_value
-
uint32_t blend_mode
-
uint32_t src_mode
-
uint32_t high_quality
-
uint32_t press_flag
press to change picture to the highlighted
-
uint32_t release_flag
-
uint32_t need_clip
-
uint8_t checksum
-
uint8_t animate_array_length
-
gui_obj_t base