Obj
The Object implements the basic properties of widgets on a screen. The screen widget is the root node of a widget tree. The screen coordinate system is set as follows. The origin of the polar coordinates is the negative direction of the Y axis, and the positive direction of the polar coordinates is clockwise:
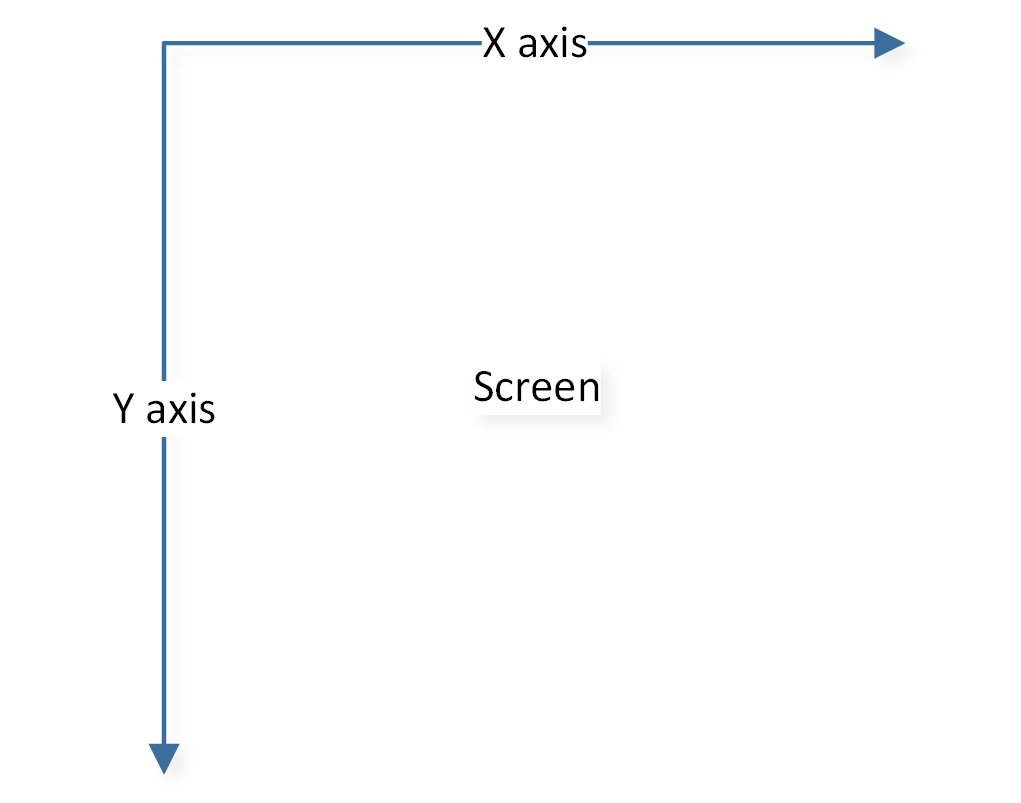
Usage
Description |
API |
---|---|
Get the root object |
|
Create object |
|
Add event |
|
Set event |
|
Free the widget tree recursively from the root to the leaves |
|
Print the widget tree recursively from the root to the leaves |
|
Get the count of one type of widget in the tree |
|
Hide/Show widget |
|
Enable object show or not |
|
Get the root object of tree |
|
Get the child object of tree |
|
Judge the object if in range of the rect |
|
Skip all actions of the parent object(left/right/down/up slide hold actions) |
|
Skip all actions of the child object(left/right/down/up slide hold actions) |
|
Skip actions of the other object(left/right/down/up slide hold actions) |
|
Get area of the object |
|
Point-in-Rectangle Range Check |
|
CRC check |
|
Get widget in tree by name |
|
Get widget in tree by type |
|
Update animate |
|
Set animate |
|
Print the tree in a breadth-first search manner |
|
API
Functions
-
gui_obj_t *gui_obj_get_root(void)
Get the root GUI object.
This function returns a pointer to the root GUI object in the widget tree.
- Returns:
A pointer to the root GUI object.
-
gui_obj_t *gui_obj_get_fake_root(void)
Get the fake_root GUI object, which would not be drawn.
This function returns a pointer to the fake_root GUI object in the widget tree.
- Returns:
A pointer to the fake_root GUI object.
-
gui_obj_t *gui_obj_create(void *parent, const char *name, int16_t x, int16_t y, int16_t w, int16_t h)
creat an obj widget.
- Parameters:
parent – the father widget it nested in.
filename – the obj widget name.
x – the X-axis coordinate of the widget.
y – the Y-axis coordinate of the widget.
w – the width of the widget.
h – the hight of the widget.
- Returns:
gui_obj_t*.
-
void gui_obj_show(void *obj, bool enable)
set object show or not.
- Parameters:
obj – the root of the widget tree.
enable – true for show, false for hide.
Example usage
static void app_main_task(gui_app_t *app) { gui_img_t *hour; gui_obj_show(hour,false); gui_obj_show(hour,true); }
-
bool gui_obj_out_screen(gui_obj_t *obj)
judge the obj if out of screen.
-
void gui_obj_get_clip_rect(gui_obj_t *obj, gui_rect_t *rect)
Calculate the clipping rectangle of a GUI object relative to its top-level ancestor.
- Parameters:
obj – The GUI object for which the clipping rectangle is calculated.
rect – The output rectangle that will contain the calculated clipping area.
-
bool gui_obj_in_rect(gui_obj_t *obj, int16_t x, int16_t y, int16_t w, int16_t h)
judge the obj if in range of this_widget rect.
- Parameters:
obj – pointer to the GUI object.
x – the X-axis coordinate of the widget.
y – the Y-axis coordinate of the widget.
w – the width of the widget.
h – the hight of the widget.
- Returns:
true.
- Returns:
false.
-
void gui_obj_enable_this_parent_short(gui_obj_t *obj)
enable all short click actions from parent object to the root object.
enable all long press actions from parent object to the root object.
- Parameters:
obj – the root of the widget tree.
-
void gui_obj_get_area(gui_obj_t *obj, int16_t *x, int16_t *y, int16_t *w, int16_t *h)
get the area of this_widget obj.
- Parameters:
obj – pointer to the GUI object.
x – the X-axis coordinate of the widget.
y – the Y-axis coordinate of the widget.
w – the width of the widget.
h – the hight of the widget.
-
bool gui_obj_point_in_obj_rect(gui_obj_t *obj, int16_t x, int16_t y)
judge the point if in range of this_widget obj rect.
- Parameters:
obj – widget object pointer.
x – the X-axis coordinate.
y – the Y-axis coordinate.
- Returns:
true.
- Returns:
false.
-
bool gui_obj_point_in_obj_circle(gui_obj_t *obj, int16_t x, int16_t y)
judge the point if in range of this_widget obj circle.
- Parameters:
obj – widget object pointer.
x – the X-axis coordinate.
y – the Y-axis coordinate.
- Returns:
true.
- Returns:
false.
-
uint8_t gui_obj_checksum(uint8_t seed, uint8_t *data, uint8_t len)
do crc check.
- Parameters:
seed – the initial value to start the checksum calculation.
data – pointer to the array of bytes for which the checksum is to be calculated.
len – the number of bytes in the array.
- Returns:
uint8_t.
-
gui_obj_t *gui_get_root(gui_obj_t *object)
print name by bfs order.
- Parameters:
object – widget pointer.
- Returns:
gui_obj_t * root.
-
void gui_obj_absolute_xy(gui_obj_t *obj, int *absolute_x, int *absolute_y)
calculate the absolute coordinates of a GUI object.
This function calculates the absolute (global) X and Y coordinates of a given GUI object based on its local position within the parent hierarchy.
Note
This function assumes that
obj
is a valid pointer and thatabsolute_x
andabsolute_y
are valid pointers to integers.- Parameters:
obj – pointer to the GUI object for which to calculate absolute coordinates.
absolute_x – pointer to an integer where the absolute X coordinate will be stored.
absolute_y – pointer to an integer where the absolute Y coordinate will be stored.
set the visibility of a GUI object.
This function sets the visibility of a given GUI object by adjusting its hidden state.
- Parameters:
obj – pointer to the GUI object that will be updated.
hidden – boolean flag indicating whether the object should be hidden (true) or shown (false).
-
const char *gui_widget_name(gui_obj_t *widget, const char *name)
set or retrieve the name of a GUI widget.
This function sets the name of a given GUI widget if the provided name is valid. It returns the current name of the widget.
- Parameters:
widget – pointer to the GUI widget whose name will be set or retrieved.
name – pointer to a string containing the new name for the widget. If the name is valid, it will be set as the widget’s name.
- Returns:
the current name of the widget.
-
void gui_update_speed(int *speed, int speed_recode[])
update touch pad speed vertical.
This function updates the current speed and records the speed change history.
- Parameters:
speed – pointer to the current speed, which will be updated by the function.
speed_recode – array to record speed changes, which will be updated by the function.
-
void gui_inertial(int *speed, int end_speed, int *offset)
inertial calculation.
This function performs inertial calculations based on the current speed, end speed, and offset.
- Parameters:
speed – pointer to the current speed, which will be updated by the function.
end_speed – target end speed.
offset – pointer to the offset, which will be updated by the function.
-
uint32_t gui_get_obj_count(void)
get widget count.
-
void gui_set_location(gui_obj_t *obj, uint16_t x, uint16_t y)
Set the location of a GUI object.
This function sets the X and Y coordinates of the specified GUI object.
- Parameters:
obj – Pointer to the GUI object to set location for.
x – The X coordinate to set.
y – The Y coordinate to set.
-
void gui_dom_create_tree_nest(const char *xml, gui_obj_t *parent_widget)
API to create a widget tree structure from an XML file and associate it with a parent widget.
- Parameters:
xml – The path to the XML file to be parsed.
parent_widget – The parent widget to which the tree structure is to be associated.
-
char *gui_dom_get_preview_image_file(const char *xml)
Extracts the preview image file path from an XML file.
This function parses the given XML file and attempts to find the preview image file path by looking for specific tags within the XML.
- Parameters:
xml_file – The path to the XML file to be parsed.
- Returns:
A string containing the path to the preview image file. If the XML file cannot be loaded or the preview image file path cannot be found, returns NULL.
-
void gui_update_speed_by_displacement(int *speed, int speed_recode[], int displacement)
Update the speed based on displacement.
This function updates the speed value based on the given displacement. It also uses a speed record array to achieve this.
- Parameters:
speed – Pointer to the speed variable to update.
speed_recode – Array holding the speed records.
displacement – The displacement value to consider for speed update.
-
void gui_obj_move(gui_obj_t *obj, int x, int y)
Move a widget object to specified coordinates.
This function moves the specified widget object to a new (x, y) coordinate position.
- Parameters:
obj – Pointer to the widget object to be moved.
x – The new x-coordinate for the widget object.
y – The new y-coordinate for the widget object.
-
void gui_obj_create_timer(gui_obj_t *obj, uint32_t interval, bool reload, void (*callback)(void*))
Set a timer for a GUI object.
This function sets a timer for the specified GUI object with a given interval. The timer can be configured to reload automatically or run only once. When the timer expires, the provided callback function is called.
- Parameters:
obj – Pointer to the GUI object to set the timer for.
interval – The interval in milliseconds for the timer.
reload – Boolean flag indicating whether the timer should reload automatically (true) or run only once (false).
callback – Pointer to the callback function to be called when the timer expires.
-
void gui_obj_delete_timer(gui_obj_t *obj)
-
void gui_obj_start_timer(gui_obj_t *obj)
-
void gui_obj_stop_timer(gui_obj_t *obj)