View
The view widget is a kind of container that makes switching more convenient. Any new view widget can be created in real time in response to an event(clicking and sliding in all four directions…) and multiple switching effects can be selected. During the switching process, there will be two views in the memory, and after the switching is completed, the undisplayed view will be automatically cleaned up, which can effectively reduce the memory consumption.
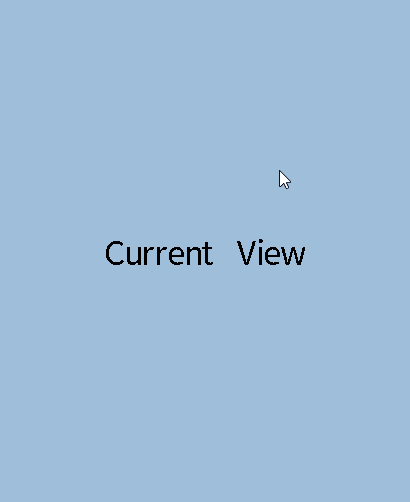
Usage
Register Descriptor of View
The gui_view_descriptor_register()
function can be used to register descriptor of view in the descriptor list for other view to read and use as a parameter to create the view, via passing in the descriptor’s address. The gui_view_descriptor
structure is defined as follows:
typedef struct gui_view_descriptor
{
const char *name;
gui_view_t **pView;
void (* on_switch_in)(gui_view_t *view); // callback function when view is switched in and created
void (* on_switch_out)(gui_view_t
*view); // callback function when view is switched out and destroyed
uint8_t keep : 1;
} gui_view_descriptor_t; // if keep is true, the view will not be destroyed when switch to other view and will be created when register view
Get Descriptor of View by Name
The gui_view_descriptor_get()
function can be used to get the view descriptor with the corresponding name
by passing in the string.
Create View Widget
The gui_view_create()
function can be used to establish a view widget.
Set Switch View Event
The gui_view_switch_on_event()
function can be used to set switch view event. Repeatable settings for a particular event will use the latest descriptor. Specific events include GUI_EVENT_TOUCH_CLICKED
、 GUI_EVENT_KB_SHORT_CLICKED
、 GUI_EVENT_TOUCH_MOVE_LEFT
、 GUI_EVENT_TOUCH_MOVE_RIGHT
and so on. The available switching styles include the following:
typedef enum
{
VIEW_STILL = 0x0000, ///< Overlay effect with new view transplate in
VIEW_TRANSPLATION = 0x0001, ///< Transplate from the slide direction
VIEW_REDUCTION = 0x0002, ///< Zoom in from the slide direction
VIEW_ROTATE = 0x0003, ///< Rotate in from the slide direction
VIEW_CUBE = 0x0004, ///< Rotate in from the slide direction like cube
VIEW_ANIMATION_NULL = 0x0005,
VIEW_ANIMATION_1, ///< Recommended for startup
VIEW_ANIMATION_2, ///< Recommended for startup
VIEW_ANIMATION_3, ///< Recommended for startup
VIEW_ANIMATION_4, ///< Recommended for startup
VIEW_ANIMATION_5, ///< Recommended for startup
VIEW_ANIMATION_6, ///< Recommended for shutdown
VIEW_ANIMATION_7, ///< Recommended for shutdown
VIEW_ANIMATION_8, ///< Recommended for shutdown
} VIEW_SWITCH_STYLE;
Switch View Directly
The gui_view_switch_direct()
function can be used to switch view directly, which can be used in conjunction with events or animations of the child widgets based on view. Note that the switching style is limited to the animation style and cannot be set to the sliding style.
Get Current View Pointer
The gui_view_get_current_view()
function can be used to get current view pointer, and can be used with gui_view_switch_direct()
to switch the current view.
Example
View
Below are three separate C files, each containing a descriptor for the view and the design function.
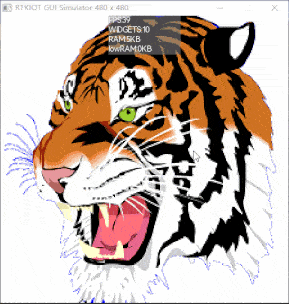
API
Defines
-
EVENT_NUM_MAX
Enums
-
enum VIEW_SWITCH_STYLE
Values:
-
enumerator VIEW_STILL
Overlay effect with new view transplate in.
-
enumerator VIEW_TRANSPLATION
Transplate from the slide direction.
-
enumerator VIEW_REDUCTION
Zoom in from the slide direction.
-
enumerator VIEW_ROTATE
Rotate in from the slide direction.
-
enumerator VIEW_CUBE
Rotate in from the slide direction like cube.
-
enumerator VIEW_ANIMATION_NULL
-
enumerator VIEW_ANIMATION_1
Recommended for startup.
-
enumerator VIEW_ANIMATION_2
Recommended for startup.
-
enumerator VIEW_ANIMATION_3
Recommended for startup.
-
enumerator VIEW_ANIMATION_4
Recommended for startup.
-
enumerator VIEW_ANIMATION_5
Recommended for startup.
-
enumerator VIEW_ANIMATION_6
Recommended for shutdown.
-
enumerator VIEW_ANIMATION_7
Recommended for shutdown.
-
enumerator VIEW_ANIMATION_8
Recommended for shutdown.
-
enumerator VIEW_STILL
Functions
-
gui_view_t *gui_view_create(void *parent, const gui_view_descriptor_t *descriptor, int16_t x, int16_t y, int16_t w, int16_t h)
Create a view widget.
- Parameters:
parent – The father widget it nested in.
descriptor – Pointer to a descriptor that defines the new view to switch to.
x – The X-axis coordinate relative to parent widget
y – The Y-axis coordinate relative to parent widget
w – Width
h – Height
- Returns:
return the widget object pointer.
-
void gui_view_descriptor_register(const gui_view_descriptor_t *descriptor)
Register view’s descriptor.
- Parameters:
descriptor – Pointer to a descriptor that defines the new view to switch to.
-
const gui_view_descriptor_t *gui_view_descriptor_get(const char *name)
Get target view’s descriptor by name.
- Parameters:
name – View descriptor’s name that can used to find target view.
-
void gui_view_switch_on_event(gui_view_t *_this, const gui_view_descriptor_t *descriptor, VIEW_SWITCH_STYLE switch_out_style, VIEW_SWITCH_STYLE switch_in_style, gui_event_t event)
Switches the current GUI view to a new view based on the specified event.
This function handles the transition between GUI views. It takes the current view context and switches it to a new view as described by the
descriptor
. The transition is triggered by a specified event and can be customized with different switch styles for the outgoing and incoming views.- Parameters:
_this – Pointer to the current GUI view context that is being manipulated.
descriptor – Pointer to a descriptor that defines the new view to switch to.
switch_out_style – Style applied to the outgoing view during the switch.
switch_in_style – Style applied to the incoming view during the switch.
event – The event that triggers the view switch.
-
void gui_view_switch_direct(gui_view_t *_this, const gui_view_descriptor_t *descriptor, VIEW_SWITCH_STYLE switch_out_style, VIEW_SWITCH_STYLE switch_in_style)
Switches directly the current GUI view to a new view through animation.
This function handles the transition between GUI views. It takes the current view context and switches it to a new view as described by the
descriptor
. The transition animation can be customized with different animation switch styles for the outgoing and incoming views.- Parameters:
_this – Pointer to the current GUI view context that is being manipulated.
descriptor – Pointer to a descriptor that defines the new view to switch to.
switch_out_style – Style applied to the outgoing view during the switch.
switch_in_style – Style applied to the incoming view during the switch.
-
gui_view_t *gui_view_get_current_view(void)
Get current view pointer.
- Returns:
return current view pointer.
-
struct gui_view_id_t
-
struct gui_view_t
Public Members
-
gui_obj_t base
-
int16_t release_x
-
int16_t release_y
-
gui_animate_t *animate
-
gui_view_id_t cur_id
-
VIEW_SWITCH_STYLE style
-
const struct gui_view_descriptor *descriptor
-
uint32_t view_switch_ready
-
uint32_t event
-
uint32_t moveback
-
uint32_t view_tp
-
uint32_t view_left
-
uint32_t view_right
-
uint32_t view_up
-
uint32_t view_down
-
uint32_t view_click
-
uint32_t view_touch_long
-
uint32_t view_button
-
uint32_t view_button_long
-
struct gui_view_on_event **on_event
-
uint8_t on_event_num
-
uint8_t checksum
-
gui_obj_t base
-
struct gui_view_descriptor_t
Public Members
-
const char *name
-
gui_view_t **pView
-
void (*on_switch_in)(gui_view_t *view)
-
void (*on_switch_out)(gui_view_t *view)
-
uint8_t keep
-
const char *name
-
struct gui_view_on_event_t
Public Members
-
const gui_view_descriptor_t *descriptor
-
VIEW_SWITCH_STYLE switch_out_style
-
VIEW_SWITCH_STYLE switch_in_style
-
gui_event_t event
-
const gui_view_descriptor_t *descriptor