LoopBack
This sample demonstrates the CAN loopback test to send and receive frame data. Use the CAN message buffer to send standard data frames, extended data frames, standard remote frames, extended remote frames, FD standard data frames, and FD extended data frame types respectively. Print the frame data received by the CAN message buffer on the Debug Analyzer.
Requirements
The sample supports the following development kits:
Hardware Platforms |
Board Name |
---|---|
RTL87x2G HDK |
RTL87x2G EVB |
For more requirements, please refer to Quick Start.
Wiring
Connect P3_2 to P3_4
Building and Downloading
This sample can be found in the SDK folder:
Project file: samples\peripheral\can\loopback\proj\rtl87x2g\mdk
Project file: samples\peripheral\can\loopback\proj\rtl87x2g\gcc
To build and run the sample, follow the steps listed below:
Open sample project file.
To build the target, follow the steps listed on the Generating App Image in Quick Start.
After a successful compilation, the app bin
app_MP_xxx.bin
will be generated in the directorymdk\bin
orgcc\bin
.To download app bin into EVB board, follow the steps listed on the MP Tool Download in Quick Start.
Press reset button on EVB board and it will start running.
Experimental Verification
Execute CAN initialization and read the bus status before and after initialization respectively.
[CAN] BUS state: 0, waiting... [CAN] BUS ON 1
Send Standard Data Frame, Extended Data Frame, Standard Remote Frame, Extended Remote Frame, FD Standard Data Frame, FD Extended Data Frame respectively, and print within the log tool.
[CAN] rx frame_type x [CAN] rx frame_id = xxx , ext_frame_id = xxx [CAN] rx_data [0] xxx ... [CAN] BUS RX done ...
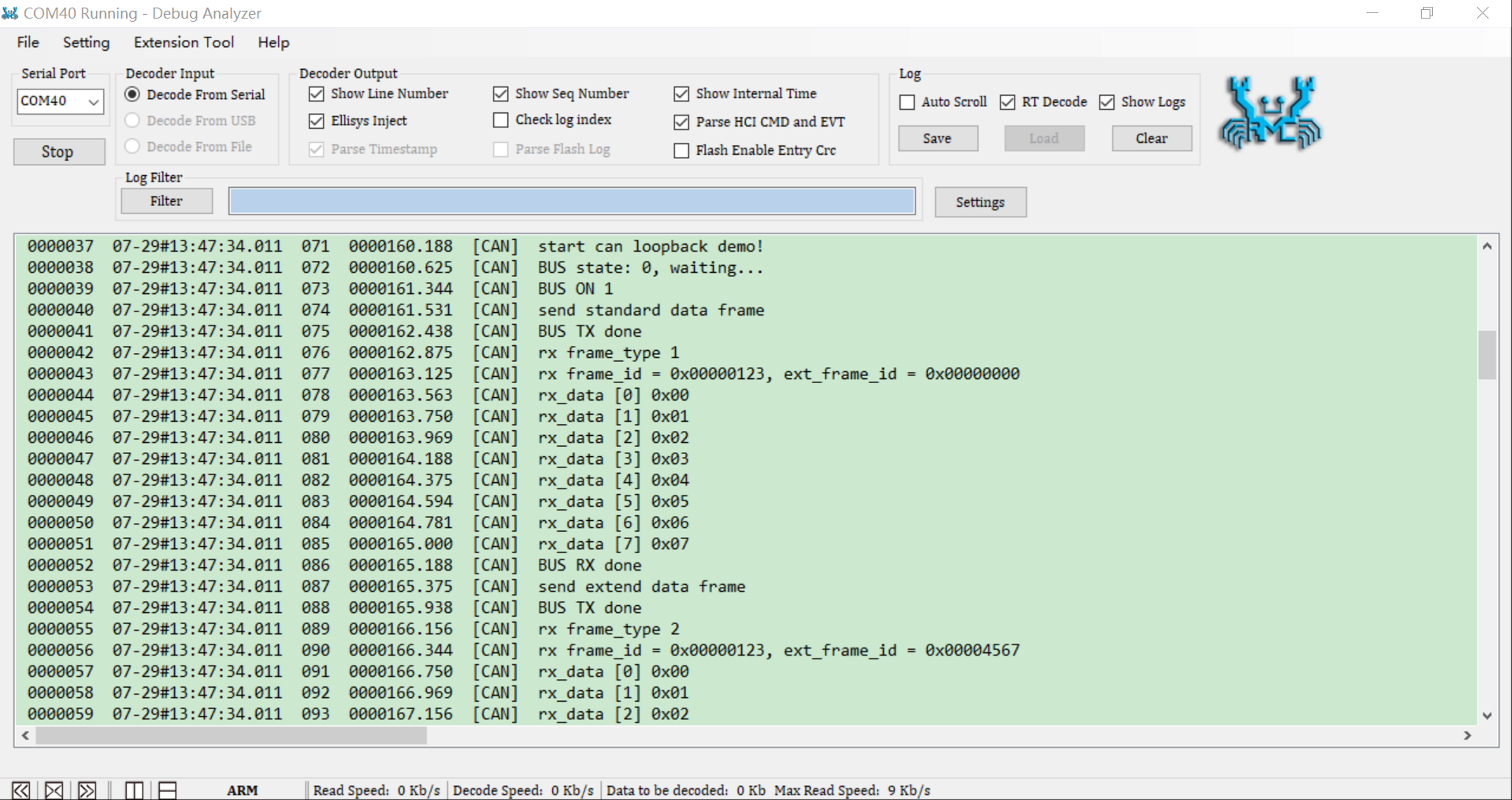
CAN log
Code Overview
This chapter will be introduced according to the following several parts:
Peripheral initialization will be introduced in chapter Initialization .
Functional implementation after initialization will be introduced in chapter Functional Implementation .
Source Code Directory
Project Directory:
sdk\samples\peripheral\can\loopback\proj
Source Code Directory:
sdk\samples\peripheral\can\loopback\src
Source files are currently categorized into several groups as below.
└── Project: loopback
└── secure_only_app
└── Device includes startup code
├── startup_rtl.c
└── system_rtl.c
├── CMSIS includes CMSIS header files
├── CMSE Library Non-secure callable lib
├── Lib includes all binary symbol files that user application is built on
└── rtl87x2g_io.lib
├── Peripheral includes all peripheral drivers and module code used by the application
├── rtl_rcc.c
├── rtl_pinmux.c
└── rtl_can.c
└── APP includes the ble_peripheral user application implementation
├── main_ns.c
└── io_can.c
Initialization
The initialization process includes can_driver_init
.
can_driver_init
contains the initialization of the CAN peripheral.
Enable PCC Clock.
Disable CAN auto re-transmission function.
Set CAN bus bit sync timing. Configure CAN speed to 500 kHz.
Enable CAN FD. Set CAN FD bit sync timing in the data field. Configure CAN FD speed to 5 MHz. Enable can_fd_auto_ssp function.
Configure the CAN test mode to loopback mode.
Enable the CAN peripheral and poll the CAN bus to be on.
Note
If the program is stuck at the point of waiting for the CAN bus to be on, please check if the CAN bus is connected correctly.
RCC_PeriphClockCmd(APBPeriph_CAN, APBPeriph_CAN_CLOCK, ENABLE);
init_struct.CAN_AutoReTxEn = DISABLE;
/* can speed = 40mhz / ((brp + 1)*(1 + tseg1 + 1 + tseg2 + 1)) */
/* Here config to 500khz. */
init_struct.CAN_BitTiming.b.can_brp = 3;
...
init_struct.CAN_FdEn = ENABLE;
init_struct.CAN_FdBitTiming.b.can_fd_brp = 0;
...
init_struct.CAN_TestModeSel = CAN_TEST_MODE_LOOP_BACK;
...
CAN_Cmd(ENABLE);
while (CAN_GetBusState() != CAN_BUS_STATE_ON)
...
Functional Implementation
Send a standard data frame, an extended data frame, a standard remote frame, an extended remote frame, an FD standard data frame, and an FD extended data frame in loopback mode.
Note
If sending several frames using the same message buffer ID, make sure to wait for the previous frame to be sent before sending the next frame.
Execute the loopback function to send and receive frames of the specified type in loopback mode.
Set the receive frame type for the rx message buffer. Use message buffer 15 as the loopback rx. Mask the rtr, ide, and id bit filters to receive all frames. Disable rx DMA. Disable auto reply. Configure the message buffer to rx mode using the set receive frame type. Poll the RAM status to be idle.
Set the tx frame type for the tx message buffer. Use the input parameters message buffer id, frame type, standard frame id, extended frame id to configure frame. Disable auto reply function. Configure the message buffer to tx mode using the set tx frame type. Poll the RAM status to be idle.
Poll tx done and rx done.
Get the rx message buffer information and read frame data from RAM.
CANError_TypeDef rx_error;
CANRxFrame_TypeDef rx_frame_type;
rx_frame_type.msg_buf_id = 15;
/* Set 0 to filter related bit, and set 1 to mask related filter. */
rx_frame_type.frame_rtr_mask = SET;
rx_frame_type.frame_ide_mask = SET;
rx_frame_type.frame_id_mask = CAN_FRAME_ID_MASK_MAX_VALUE;
rx_frame_type.rx_dma_en = RESET;
rx_frame_type.auto_reply_bit = RESET;
rx_error = CAN_SetMsgBufRxMode(&rx_frame_type);
while (CAN_GetRamState() != CAN_RAM_STATE_IDLE)
...
/* Set tx message buffer. */
CANError_TypeDef tx_error = CAN_NO_ERR;
CANTxFrame_TypeDef tx_frame_type;
tx_frame_type.msg_buf_id = buf_id;
tx_frame_type.frame_type = frame_type;
tx_frame_type.standard_frame_id = frame_id;
tx_frame_type.extend_frame_id = 0;
tx_frame_type.frame_brs_bit = CAN_BRS_NO_SWITCH_BIT_TIMING;
tx_frame_type.auto_reply_bit = DISABLE;
tx_error = CAN_SetMsgBufTxMode(&tx_frame_type, tx_data, data_len);
while (CAN_GetRamState() != CAN_RAM_STATE_IDLE)
...
/* Polling tx done. */
while (SET != CAN_GetMBnTxDoneFlag(tx_frame_type.msg_buf_id))
...
/* Polling rx done. */
while (SET != CAN_GetMBnRxDoneFlag(rx_frame_type.msg_buf_id))
...
/* Receive rx data. */
...
get_error = CAN_GetMsgBufInfo(rx_frame_type.msg_buf_id, &mb_info);
...
CAN_GetRamData(mb_info.data_length, rx_data);
CANDataFrameSel_TypeDef get_frame_type = CAN_CheckFrameType(mb_info.rtr_bit, mb_info.ide_bit, mb_info.edl_bit);
...