Mbed TLS
Mbed TLS is an open-source cryptographic library designed to be lightweight and easy to use. It provides developers with a comprehensive range of cryptographic primitives, protocols, and algorithms necessary for developing secure applications. These include support for modern SSL/TLS protocols, essential for encrypting internet traffic and ensuring secure communications between clients and servers. By focusing on security, efficiency, and ease of integration, Mbed TLS helps developers safeguard sensitive data against unauthorized access and cyber threats, all while simplifying the complexity of implementing cryptographic solutions.
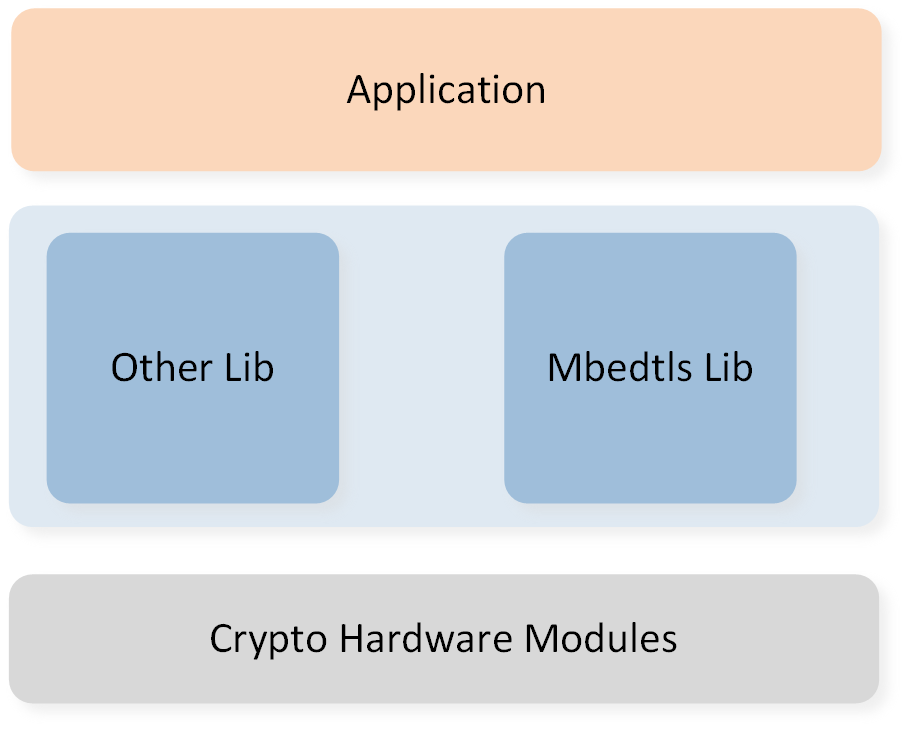
Mbed TLS Info
More information on Mbed TLS can be found on the Mbed TLS.
Features
Mbed TLS offers a robust selection of features including:
TLS/SSL Protocols: Secure communication protocols for encrypted network traffic.
X.509: Certificate handling and verification.
Random Number Generation: For cryptographic purposes.
Note
Hardware AES and hardware random number generation are exclusively supported by the default settings.
Due to memory constraints, some features are not supported, please refer to
mbedtls_config.h
.
Threading Primitives and Dynamic Memory
For the Mbed TLS component, dynamic memory allocation functions allow user configuration of application-specific allocation routines. When MBEDTLS_PLATFORM_MEMORY
is set, the application can call calloc()
and free()
as dynamic memory allocation functions.
Additionally, MBEDTLS_THREADING_ALT
is provided to enable multi-threading with FreeRTOS. This implementation leverages the CMSIS RTOS2 API to offer multi-threading support.
How to Integrate Mbed TLS into Application?
Mbed TLS has been integrated with SDK to provide secure communication and data encryption. SDK supports Mbed TLS for users, making it easier for developers to build secure IoT applications. To integrate Mbed TLS in application, follow these steps:
Setup the SDK Environment
Follow the instructions in the SDK documentation to set up your development environment.
Enable and Compile Mbed TLS
Open
sdk\board\evb\mbedtls\mbedtls.uvprojx
and users can enable Mbed TLS features inmbedtls\mbedtls_config.h
.Include Mbed TLS Headers
In your application code, include the Mbed TLS headers:
#include <mbedtls/platform.h> #include <mbedtls/net_sockets.h> #include <mbedtls/ssl.h> #include <mbedtls/ssl_ciphersuites.h> #include <mbedtls/entropy.h> #include <mbedtls/ctr_drbg.h> #include <mbedtls/x509_crt.h>
Initialize and Configure Mbed TLS
Initialize the Mbed TLS structures and configure the SSL/TLS settings as per your application’s requirements.
Example Usage
Here is a simple example to illustrate how to initialize and configure Mbed TLS for a secure connection:
#include <stdio.h>
#include <mbedtls/platform.h>
#include <mbedtls/net_sockets.h>
#include <mbedtls/ssl.h>
#include <mbedtls/ssl_ciphersuites.h>
#include <mbedtls/entropy.h>
#include <mbedtls/ctr_drbg.h>
#include <mbedtls/x509_crt.h>
int main(void)
{
int ret;
mbedtls_net_context server_fd;
mbedtls_ssl_context ssl;
mbedtls_ssl_config conf;
mbedtls_ssl_init(&ssl);
mbedtls_ssl_config_init(&conf);
mbedtls_net_init(&server_fd);
// Initialize mbed TLS structures
if((ret = mbedtls_ssl_setup(&ssl, &conf)) != 0)
{
printf("mbedtls_ssl_setup failed: %d\n", ret);
return -1;
}
// Connect to server
if((ret = mbedtls_net_connect(&server_fd, "example.com", "443", MBEDTLS_NET_PROTO_TCP)) != 0)
{
printf("mbedtls_net_connect failed: %d\n", ret);
return -1;
}
// Setup SSL configuration
mbedtls_ssl_set_bio(&ssl, &server_fd, mbedtls_net_send, mbedtls_net_recv, NULL);
// Perform TLS handshake
if((ret = mbedtls_ssl_handshake(&ssl)) != 0)
{
printf("mbedtls_ssl_handshake failed: %d\n", ret);
return -1;
}
// Your secure communication code here
// Cleanup and release resources
mbedtls_ssl_free(&ssl);
mbedtls_ssl_config_free(&conf);
mbedtls_net_free(&server_fd);
return 0;
}