LE Manager
This document aims to guide developers on how to use the Bluetooth LE manager.
The chapter LE Manager Introduction gives an overview of the LE manager. The chapter LE Extended Advertising describes the LE extended advertising manager and LE extended advertising data manager.
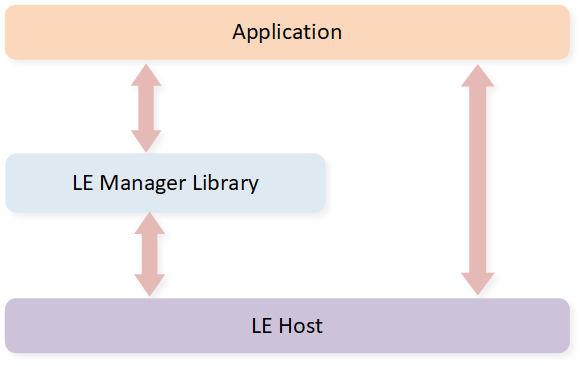
The LE manager is developed based on the interfaces provided by the LE Host. The purpose of developing this manager is to facilitate users in developing complex LE applications.
LE Manager Introduction
This chapter gives an overview of the LE manager, including:
LE Manager Sub-module Introduction
LE manager includes several sub-modules shown in the Sub-module Name column. The header file corresponding to the sub-module is displayed in the Header Files column.
Sub-module Name |
Header Files |
GAP Interface Usage Restrictions |
---|---|---|
LE Extended Advertising Manager |
|
Cannot use |
LE Extended Advertising Data Manager |
|
Cannot use |
LE Scan Manager |
|
Cannot use |
GATT Service Manager |
|
Cannot use |
GATT Client Manager |
|
Cannot use |
Bond Manager |
|
Cannot use |
LE Extended Advertising Manager and LE Extended Advertising Data Manager
The LE extended advertising manager is introduced in the chapter LE Extended Advertising Manager.
The LE extended advertising data manager is introduced in the chapter LE Extended Advertising Data Manager.
The LE extended advertising data manager is implemented based on the interface provided by the LE extended advertising manager. The interfaces exported by the LE extended advertising manager and the LE extended advertising data manager can be used simultaneously.
LE Scan Manager
When an application has multiple modules that need to use scan, it can use this module to independently manage scan. Each application module can independently manage scan policies and actions by scan handle, and has an independent callback to receive and filter reported advertising packets.
GATT Service Manager
The GATT service manager is a management module encapsulated by
ble_mgr.lib
based onprofile_server_ext.h
.The purpose of this module is to facilitate service development, it has the following advantages:
GATT Client Manager
The GATT client manager is a management module encapsulated by
ble_mgr.lib
based onprofile_client_ext.h
.The purpose of this module is to facilitate client development, it has the following advantages:
The GATT client manager implements the discovery service process internally, so the APP only needs to register the Service UUID.
The Service table storage found is organized with a unified management structure within the GATT client manager, and also provides unified sample code for the APP to store the Service table.
The GATT client manager implements the ATT Sequential protocol internally and caches requests, so the APP implementing the client is not restricted by the protocol.
Bond Manager
This module is used to manage bond information, serving as a replacement for the bond module in the GAP layer.
LE Manager Library Introduction
The functions of LE manager are provided by the ble_mgr.lib
.
The library directory and header files directory are shown as follows.
IC Type |
Library Directory |
Header Files Directory |
---|---|---|
RTL87x3E |
|
|
RTL87x3D |
|
|
RTL87x3EP |
|
If APP wants to use the LE manager, the ble_mgr.lib
shall be included in the project as shown in the following figure.
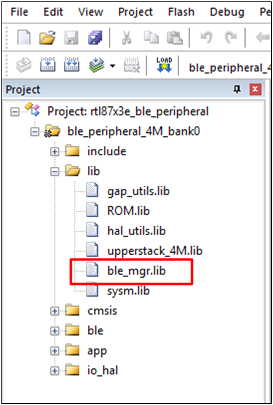
LE Manager Initialization
The initialization sample code is shown below:
void app_ble_mgr_lib_init(void)
{
BLE_MGR_PARAMS param = {0};
param.ble_ext_adv.enable = true;
param.ble_ext_adv.adv_num = 1;
#if (BAP_BROADCAST_SINK | BAP_SCAN_DELEGATOR)
param.ble_scan.enable = true;
#endif
param.ble_conn.enable = true;
param.ble_conn.link_num = APP_MAX_BLE_LINK_NUM;
ble_mgr_init(¶m);
}
ble_mgr_init()
is used to initialize the ble_mgr.lib
.
GAP message and callback handling functions are shown below:
void app_handle_gap_msg(T_IO_MSG *p_gap_msg)
{
T_LE_GAP_MSG gap_msg;
uint8_t conn_id;
memcpy(&gap_msg, &p_gap_msg->u.param, sizeof(p_gap_msg->u.param));
ble_mgr_handle_gap_msg(p_gap_msg->subtype, &gap_msg);
......
}
T_APP_RESULT app_gap_callback(uint8_t cb_type, void *p_cb_data)
{
T_APP_RESULT result = APP_RESULT_SUCCESS;
T_LE_CB_DATA *p_data = (T_LE_CB_DATA *)p_cb_data;
ble_mgr_handle_gap_cb(cb_type, p_cb_data);
......
}
ble_mgr_handle_gap_msg()
is used to handle GAP messages.
ble_mgr_handle_gap_cb()
is used to handle GAP callback messages.
LE Extended Advertising
This chapter describes the LE extended advertising manager which is a new feature introduced in Bluetooth core spec 5.0.
The constants and function prototypes are defined in inc\ble_mgr\ble_ext_adv.h
.
This module is designed to make it easy for applications to manage the operation of multiple advertisements.
This module is encapsulated on the basis of gap_adv.h
and gap_ext_adv.h
.
When using this module, in order to prevent some unnecessary conflicts, it is recommended that applications shall not directly call the interface in
gap_ext_adv.h
and gap_adv.h
.
LE Extended Advertising Manager
This chapter will be introduced according to the following several parts:
Advertising manager initialization will be introduced in chapter Advertising Manager Initialization.
Advertising parameter initialization will be introduced in chapter Advertising Parameter Initialization.
Enable or disable advertising will be introduced in chapter Enable or Disable Advertising.
Handle callbacks of advertising will be introduced in chapter Handle Callbacks of Advertising.
Advertising parameter update after Bluetooth Host ready will be introduced in chapter Advertising Parameter Update.
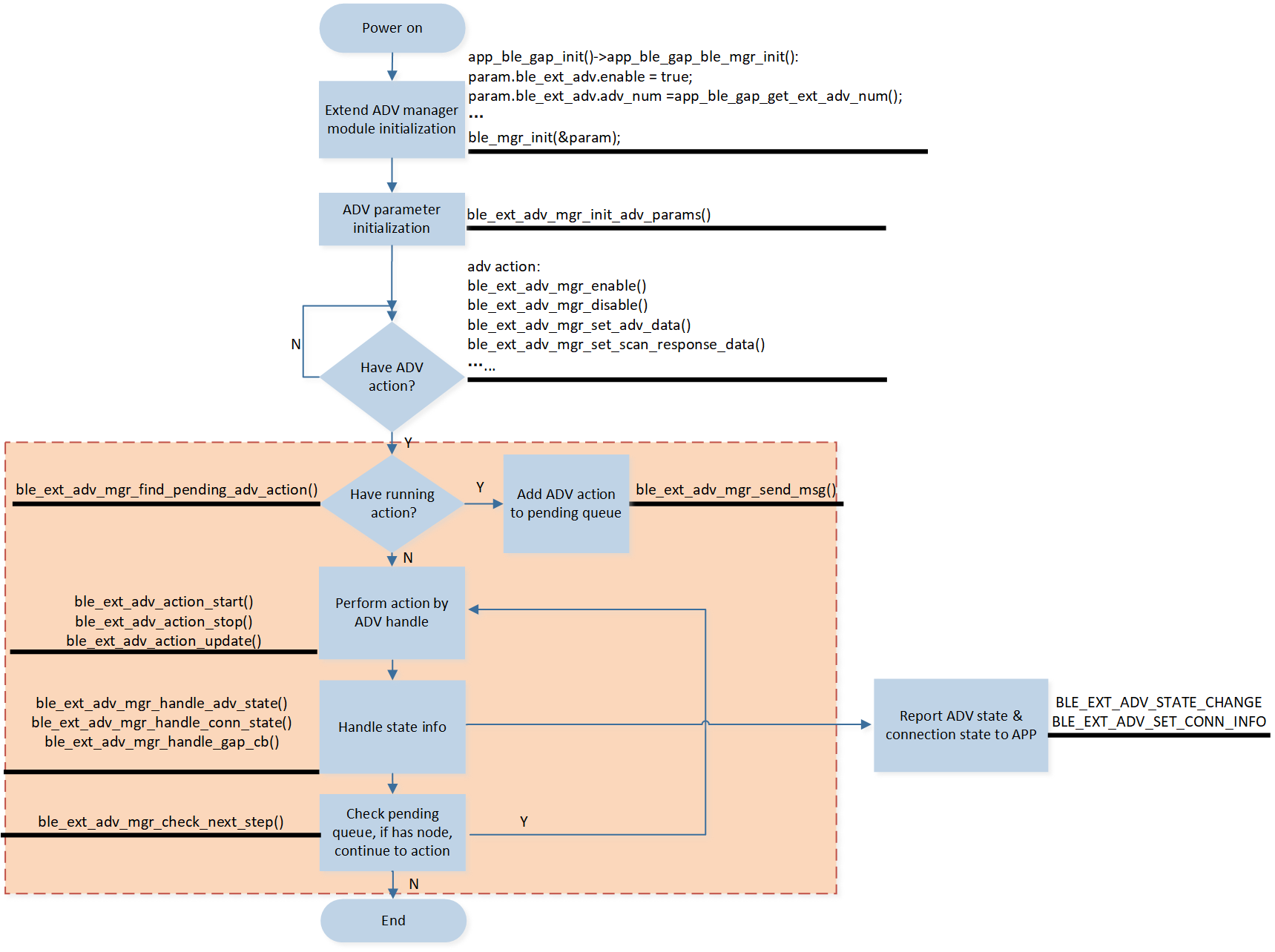
The dotted box in the figure above is implemented by Bluetooth LE manager library, the APP may not care about it.
Each advertisement has its own advertising handle and is assigned a separate advertising set to manage its own broadcast-related parameters:
First call
ble_mgr_init()
to allocate storage for different broadcasts.Then create advertising handle and set advertising parameters and advertising data for the broadcast.
Then APP can enable or disable the broadcast.
Advertising Manager Initialization
This module shall be initialized before it can be used. The initialization steps are as follows:
Please call ble_mgr_init()
to initialize the LE advertising manager.
void app_ble_gap_ble_mgr_init(void)
{
BLE_MGR_PARAMS param = {0};
param.ble_ext_adv.enable = true;
param.ble_ext_adv.adv_num = app_ble_gap_get_ext_adv_num();
param.ble_conn.enable = true;
uint8_t supported_max_le_link_num = le_get_max_link_num();
param.ble_conn.link_num = ((MAX_BLE_LINK_NUM <= supported_max_le_link_num) ? MAX_BLE_LINK_NUM :
supported_max_le_link_num);
if (extend_app_cfg_const.ama_support || extend_app_cfg_const.xiaowei_support ||
extend_app_cfg_const.bisto_support || extend_app_cfg_const.xiaoai_support)
{
param.ble_adv_data.enable = true;
param.ble_adv_data.update_scan_data = true;
param.ble_adv_data.adv_interval = (extend_app_cfg_const.multi_adv_interval * 8) / 5;
app_ble_gap_gen_scan_rsp_data(&scan_rsp_data_len, scan_rsp_data);
param.ble_adv_data.scan_rsp_len = scan_rsp_data_len;
param.ble_adv_data.scan_rsp_data = scan_rsp_data;
}
param.ble_scan.enable = true;
ble_mgr_init(¶m);
}
void app_ble_gap_init(void)
{
......
app_ble_gap_ble_mgr_init();
}
int main(void)
{
......
app_ble_gap_init();
......
}
param.ble_ext_adv.enable = true;
means enable LE extended advertising.param.ble_ext_adv.adv_num = app_ble_gap_get_ext_adv_num();
means the advertising handle number.If APP wants to add a new advertising, the parameter
param.ble_ext_adv.adv_num
shall be +1. The maximum advertising set number is 10.
Advertising Parameter Initialization
APP can use ble_ext_adv_mgr_init_adv_params()
to initialize advertising parameters.
This API is used to create advertising handle and initialize advertising parameters, advertising data, scan response data and random address.
void app_ble_common_adv_init(void)
{
T_LE_EXT_ADV_LEGACY_ADV_PROPERTY adv_event_prop = LE_EXT_ADV_LEGACY_ADV_CONN_SCAN_UNDIRECTED;
uint16_t adv_interval_min = 0xA0;
uint16_t adv_interval_max = 0xB0;
T_GAP_LOCAL_ADDR_TYPE own_address_type = GAP_LOCAL_ADDR_LE_RANDOM;
if (le_common_adv.use_static_addr_type)
{
own_address_type = GAP_LOCAL_ADDR_LE_RANDOM;
}
else
{
own_address_type = GAP_LOCAL_ADDR_LE_PUBLIC;
}
T_GAP_REMOTE_ADDR_TYPE peer_address_type = GAP_REMOTE_ADDR_LE_PUBLIC;
uint8_t peer_address[6] = {0, 0, 0, 0, 0, 0};
T_GAP_ADV_FILTER_POLICY filter_policy = GAP_ADV_FILTER_ANY;
uint8_t data_len = sizeof(app_cfg_nv.bud_local_addr) + sizeof(app_cfg_const.company_id);
app_ble_gap_gen_scan_rsp_data(&scan_rsp_data_len, scan_rsp_data);
uint8_t le_random_addr[6] = {0};
app_ble_rand_addr_get(le_random_addr);
ble_ext_adv_mgr_init_adv_params(&le_common_adv.adv_handle, adv_event_prop, adv_interval_min,
adv_interval_max, own_address_type, peer_address_type, peer_address,
filter_policy, 23 + data_len, app_ble_common_adv_data,
scan_rsp_data_len, scan_rsp_data, le_random_addr);
ble_ext_adv_mgr_register_callback(app_ble_common_adv_callback, le_common_adv.adv_handle);
}
static void app_ble_gap_bt_cback(T_BT_EVENT event_type, void *event_buf, uint16_t buf_len)
{
T_BT_EVENT_PARAM *param = event_buf;
switch (event_type)
{
case BT_EVENT_READY:
{
if (app_cfg_const.rtk_app_adv_support || app_cfg_const.tts_support)
{
/* init here to avoid app_cfg_nv.bud_local_addr no mac info (due to factory reset) */
app_ble_common_adv_init();
}
}
break;
default:
break;
}
}
T_LE_EXT_ADV_LEGACY_ADV_PROPERTY adv_event_prop = LE_EXT_ADV_LEGACY_ADV_CONN_SCAN_UNDIRECTED;
Set adv_event_prop, the value please refer to
T_LE_EXT_ADV_LEGACY_ADV_PROPERTY
.uint16_t adv_interval_min = 0xA0; uint16_t adv_interval_max = 0xB0;
Set advertising interval:
adv_interval_min: Minimum advertising interval for undirected and low duty directed advertising. In units of 0.625 ms, range: 0x000020 to 0xFFFFFF.
adv_interval_max: Maximum advertising interval for undirected and low duty directed advertising. In units of 0.625 ms, range: 0x000020 to 0xFFFFFF.
T_GAP_LOCAL_ADDR_TYPE own_address_type = GAP_LOCAL_ADDR_LE_RANDOM;
Set own_address_type, the value please refer to
T_GAP_LOCAL_ADDR_TYPE
.T_GAP_REMOTE_ADDR_TYPE peer_address_type = GAP_REMOTE_ADDR_LE_PUBLIC;
Set peer_address_type (only used for directed advertising).
T_GAP_ADV_FILTER_POLICY filter_policy = GAP_ADV_FILTER_ANY;
Set filter_policy, the value please refer to
T_GAP_ADV_FILTER_POLICY
.ble_ext_adv_mgr_init_adv_params()
Set advertising parameters into Bluetooth Host and create advertising handle.
ble_ext_adv_mgr_register_callback()
Register advertising callback, each advertisement has its own advertising callback. Advertising state and connection state will be reported by this callback.
Enable or Disable Advertising
ble_ext_adv_mgr_enable()
is used to enable advertising.If
ble_ext_adv_mgr_enable()
returnsGAP_CAUSE_SUCCESS
, it means the Bluetooth Host has already received this command and is ready to execute it, and when this command execution is complete, the Bluetooth Host will sendBLE_EXT_ADV_MGR_ADV_ENABLED
to the callback functions registered for advertising.bool app_ble_common_adv_start(uint16_t duration_10ms) { if (le_common_adv.state == BLE_EXT_ADV_MGR_ADV_DISABLED) { if (ble_ext_adv_mgr_enable(le_common_adv.adv_handle, duration_10ms) == GAP_CAUSE_SUCCESS) { return true; } else { return false; } } else { APP_PRINT_TRACE0("app_ble_common_adv_start: Already started"); return true; } }
ble_ext_adv_mgr_disable()
is used to disable advertising.If
ble_ext_adv_mgr_disable()
returnsGAP_CAUSE_SUCCESS
, it means the Bluetooth Host has already received this command and is ready to execute. When this command execution is complete, the Bluetooth Host will sendBLE_EXT_ADV_MGR_ADV_DISABLED
to the callback functions registered for advertising.bool app_ble_common_adv_stop(int8_t app_cause) { APP_PRINT_INFO0("app_ble_common_adv_stop"); if (ble_ext_adv_mgr_disable(le_common_adv.adv_handle, app_cause) == GAP_CAUSE_SUCCESS) { return true; } else { return false;s } }
Handle Callbacks of Advertising
Two events will be notified: BLE_EXT_ADV_STATE_CHANGE
and BLE_EXT_ADV_SET_CONN_INFO
.
-
This message is used to notify the application that the final desired advertising state of the registered advertising set. There are two advertising state events that may be sent to the advertising set callback function:
BLE_EXT_ADV_MGR_ADV_ENABLED
andBLE_EXT_ADV_MGR_ADV_DISABLED
. -
This message is used to notify the application of the connection information for LE link that was established with the advertising set.
static void app_ble_common_adv_callback(uint8_t cb_type, void *p_cb_data)
{
T_BLE_EXT_ADV_CB_DATA cb_data;
memcpy(&cb_data, p_cb_data, sizeof(T_BLE_EXT_ADV_CB_DATA));
switch (cb_type)
{
case BLE_EXT_ADV_STATE_CHANGE:
{
le_common_adv.state = cb_data.p_ble_state_change->state;
if (le_common_adv.state == BLE_EXT_ADV_MGR_ADV_ENABLED)
{
}
else if (le_common_adv.state == BLE_EXT_ADV_MGR_ADV_DISABLED)
{
switch (cb_data.p_ble_state_change->stop_cause)
{
case BLE_EXT_ADV_STOP_CAUSE_APP:
break;
case BLE_EXT_ADV_STOP_CAUSE_CONN:
break;
case BLE_EXT_ADV_STOP_CAUSE_TIMEOUT:
if ((app_cfg_const.bud_role == REMOTE_SESSION_ROLE_SECONDARY) && le_common_adv.use_static_addr_type)
{
/*secondary ear ota need modify the random address and start advertising,
when advertising timeout, the random address shall be set back to le_rws_random_addr*/
uint8_t rand_addr[6] = {0};
app_ble_rand_addr_get(rand_addr);
app_ble_common_adv_set_random(rand_addr);
}
break;
default:
break;
}
}
}
break;
case BLE_EXT_ADV_SET_CONN_INFO:
APP_PRINT_TRACE4("app_ble_common_adv_callback: BLE_EXT_ADV_SET_CONN_INFO conn_id 0x%x, adv_handle %d, local_addr_type %d, local_bd %b",
cb_data.p_ble_conn_info->conn_id,
cb_data.p_ble_conn_info->adv_handle,
cb_data.p_ble_conn_info->local_addr_type,
TRACE_BDADDR(cb_data.p_ble_conn_info->local_addr));
break;
default:
break;
}
return;
}
Advertising Parameter Update
ble_ext_adv_mgr_set_adv_data()
is used to update advertising data.void app_ble_common_adv_bud_role_update(T_REMOTE_SESSION_ROLE role) { /*app_ble_common_adv_data update bud role*/ if (app_ble_common_adv_data[20] != role) { app_ble_common_adv_data[20] = role; ble_ext_adv_mgr_set_adv_data(le_common_adv.adv_handle, sizeof(app_ble_common_adv_data), app_ble_common_adv_data); } else { //bud role not change } }
ble_ext_adv_mgr_set_random()
is used to update random addr.T_GAP_CAUSE app_adv_update_randomaddr(uint8_t *random_address) { return ble_ext_adv_mgr_set_random(adv_handle, random_address); }
ble_ext_adv_mgr_set_scan_response_data()
is used to update scan response data.T_GAP_CAUSE app_adv_update_scanrspdata(uint8_t *p_scan_data, uint16_t scan_data_len) { return ble_ext_adv_mgr_set_scan_response_data(adv_handle, scan_data_len, p_scan_data); }
Update advertising parameters:
LE Extended Advertising Data Manager
If APP expects to use the public address to advertise multiple connectable advertisings. It is recommended to use the LE extended advertising data manager.
This manager only starts advertising with one advertising handle, and this manager will add new advertising data to the queue and regularly update advertising data to achieve different advertisements.
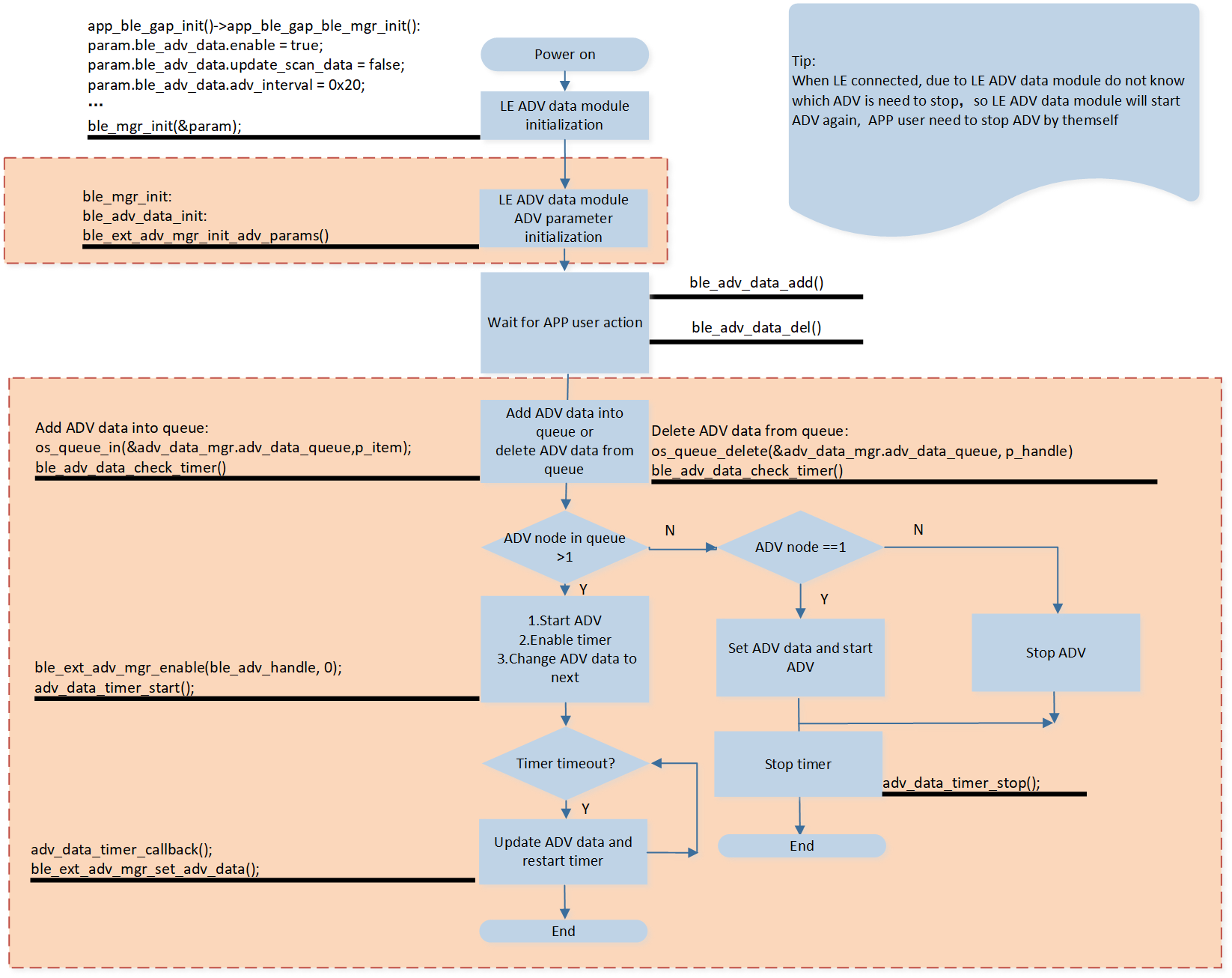
The dotted box in the figure above is implemented by the LE manager library, APP may not care about it.
Limitations:
Only one advertising interval can be set, shared by multiple advertising data.
For example, if the advertising interval is 50ms, only one kind of advertising data needs to be sent, the interval is 50ms. If two kinds of advertising data need to be sent, the interval is 100ms, and so on.
adv_event_prop
cannot be specified, the default value isLE_EXT_ADV_LEGACY_ADV_CONN_SCAN_UNDIRECTED
.Advertising address can only set public address, other address type is invalid.
Advertising Data Initialization
Call ble_mgr_init()
to initialize the LE advertising data manager.
void app_ble_gap_ble_mgr_init(void)
{
BLE_MGR_PARAMS param = {0};
param.ble_ext_adv.enable = true;
param.ble_ext_adv.adv_num = app_ble_gap_get_ext_adv_num();
......
if (extend_app_cfg_const.ama_support || extend_app_cfg_const.xiaowei_support ||
extend_app_cfg_const.bisto_support || extend_app_cfg_const.xiaoai_support)
{
param.ble_adv_data.enable = true;
param.ble_adv_data.update_scan_data = true;
param.ble_adv_data.adv_interval = (extend_app_cfg_const.multi_adv_interval * 8) / 5;
app_ble_gap_gen_scan_rsp_data(&scan_rsp_data_len, scan_rsp_data);
param.ble_adv_data.scan_rsp_len = scan_rsp_data_len;
param.ble_adv_data.scan_rsp_data = scan_rsp_data;
}
......
ble_mgr_init(¶m);
}
void app_ble_gap_init(void)
{
......
app_ble_gap_ble_mgr_init();
}
int main(void)
{
......
app_ble_gap_init();
......
}
param.ble_adv_data.enable = true;
It means enabling the LE advertising data manager.
param.ble_adv_data.update_scan_data = true;
It means the LE advertising data manager updates scan response data.
param.ble_adv_data.adv_interval = (extend_app_cfg_const.multi_adv_interval * 8) / 5;
It means the LE advertising data manager sets the advertising interval.
Enable or Disable Advertising Data
The function ble_adv_data_enable()
is used to enable the advertising data manager,
and the function ble_adv_data_disable()
is used to disable the advertising data manager.
The function ble_adv_data_add()
is used to add the advertising data and scan response
data into the data queue. Then, the corresponding advertising packet will be transmitted over the air.
bool le_xm_xiaoai_adv_start(uint16_t timeout_sec)
{
......
if (ble_adv_data_add(&p_le_xm_xiaoai_adv_handle, sizeof(xm_xiaoai_adv_data),
(uint8_t *)&xm_xiaoai_adv_data,
sizeof(xm_xiaoai_scan_rsp_data), (uint8_t *)&xm_xiaoai_scan_rsp_data))
{
app_xiaoai_device_start_adv_timer(timeout_sec);
return true;
}
......
}
The function ble_adv_data_del()
is used to delete the advertising data and scan response
data from the data queue. Then, the corresponding advertising packet will not be transmitted over the air.
bool le_xm_xiaoai_adv_stop(void)
{
......
if (ble_adv_data_del(p_le_xm_xiaoai_adv_handle))
{
p_le_xm_xiaoai_adv_handle = NULL;
return true;
}
......
}