User Command Interface
This document aims to guide users on how to use the user command interface.
The user commands are used by LE Central Sample, LE Scatternet Sample, and LE Central Extended Scan Sample. These samples needs to perform interactions by inputting commands through Data UART in PC (USB port in PC is connected to EVB via USB-to-serial port module).
The user command interface will be introduced according to the following several parts:
User command implementation will be introduced in the chapter Implementation of User Command.
Data UART connection will be introduced in the chapter Data UART Connection.
Implementation of User Command
Initialization
void app_main_task(void *p_param)
{
uint8_t event;
......
data_uart_init(evt_queue_handle, io_queue_handle);
user_cmd_init(&user_cmd_if, "central");
......
}
data_uart_init()
is used to initialize the Data UART.
user_cmd_init()
is used to initialize the user command module.
Data UART RX Handler
void app_handle_io_msg(T_IO_MSG io_msg)
{
uint16_t msg_type = io_msg.type;
uint8_t rx_char;
switch (msg_type)
{
......
case IO_MSG_TYPE_UART:
/* We handle user command information from Data UART in this branch. */
rx_char = (uint8_t)io_msg.subtype;
user_cmd_collect(&user_cmd_if, &rx_char, sizeof(rx_char), user_cmd_table);
break;
default:
break;
}
}
user_cmd_collect()
is used to collect command characters and execute the command.
Users can input a command through the serial port assistant tool, and the command is terminated by pressing ENTER.
Data UART TX Handler
Data UART TX is used to output information and logs.
void app_handle_conn_state_evt(uint8_t conn_id, T_GAP_CONN_STATE new_state, uint16_t disc_cause)
{
......
case GAP_CONN_STATE_CONNECTED:
{
le_get_conn_addr(conn_id, app_link_table[conn_id].bd_addr,
&app_link_table[conn_id].bd_type);
data_uart_print("Connected success conn_id %d\r\n", conn_id);
}
break;
......
}
data_uart_print()
is used to output the information through the Data UART.
Users can see the information in the serial port assistant tool.
How to Add Commands
The application shall define user command table.
The format of a command is as follows:
/** @brief Prototype of functions that can be called from command table. */
typedef T_USER_CMD_PARSE_RESULT(*T_USER_CMD_FUNC)(T_USER_CMD_PARSED_VALUE *p_parse_value);
/**
* @brief Command table entry.
*
*/
typedef struct
{
char *p_cmd;
char *p_option;
char *p_help;
T_USER_CMD_FUNC func;
} T_USER_CMD_TABLE_ENTRY;
The p_cmd field contains the command text used in the serial communication software.
The p_option field describes the format of a user command.
The p_help field provides a description of the command’s functionality.
The func field points to the execution function associated with the command, which would be executed when the command is invoked.
A sample of user command table is shown as below:
static T_USER_CMD_PARSE_RESULT cmd_conupdreq(T_USER_CMD_PARSED_VALUE *p_parse_value)
{
T_GAP_CAUSE cause;
uint8_t conn_id = p_parse_value->dw_param[0];
uint16_t conn_interval_min = p_parse_value->dw_param[1];
uint16_t conn_interval_max = p_parse_value->dw_param[2];
uint16_t conn_latency = p_parse_value->dw_param[3];
uint16_t supervision_timeout = p_parse_value->dw_param[4];
cause = le_update_conn_param(conn_id,
conn_interval_min,
conn_interval_max,
conn_latency,
supervision_timeout,
2 * (conn_interval_min - 1),
2 * (conn_interval_max - 1)
);
return (T_USER_CMD_PARSE_RESULT)cause;
}
const T_USER_CMD_TABLE_ENTRY user_cmd_table[] =
{
/************************** Common cmd *************************************/
{
"conupdreq",
"conupdreq [conn_id] [interval_min] [interval_max] [latency] [supervision_timeout]\n\r",
"LE connection param update request\r\n\
sample: conupdreq 0 0x30 0x40 0 500\n\r",
cmd_conupdreq
},
......
};
How to Use Commands
Taking conupdreq [conn_id] [interval_min] [interval_max] [latency] [supervision_timeout]
as an example, conupdreq
is the name of the command, followed by parameters separated with spaces.
Users can enter the command in serial port assistant tool in PC, and then press ENTER or click on Send to send it. The recommended serial port assistant tool is Tera Term.
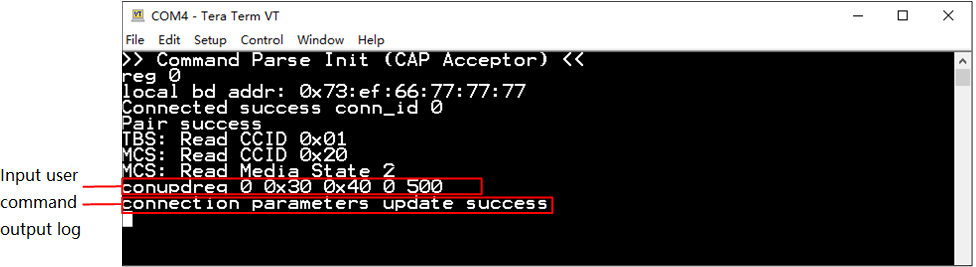
How to Show Commands
Input ?
to display all commands.
Input xxx ?
to display command format, such as conupdreq ?
.
Data UART Connection
LE Central Sample, LE Scatternet Sample, and LE Central Extended Scan Sample use P3_0 as the TX pin for Data UART, and use P3_1 as the RX pin for Data UART by default.
Each sample has a copy of board.h
file, which contains the definition of the Data UART pin.
#define DATA_UART_TX_PIN P3_0
#define DATA_UART_RX_PIN P3_1
Data UART pin can be configured according to the hardware environment.
Therefore, PC is connected to Data UART of EVB running LE Central Sample, LE Scatternet Sample, or LE Central Extended Scan Sample via USB-to-serial port module, as shown in the figure below:
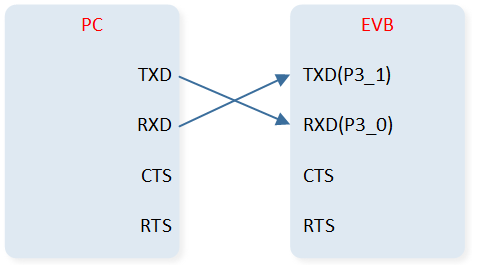
The baud rate of Data UART is set to 115,200, and other parameters for serial port assistant tool in PC can be set as shown in Figure:
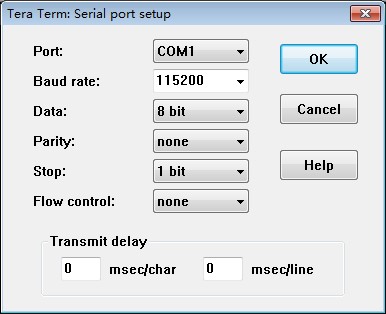