Canvas
The canvas widget is the basic widget used to drawing graphics in nanovg.
Warning
Sufficient memory is needed to open a framebuffer.
Usage
Creat Canvas
gui_canvas_create()
creates a canvas.
Add Callback Function
gui_canvas_set_canvas_cb()
sets the callback function for drawing specific shapes.
Example
Rounded Rectangle
A simple example of drawing three rounded rectangles of different colors.
Three color refer to Colors’ RGB Data.
firebrick
olive drab
dodger blue
with 100 value opacity
#include "gui_canvas.h"
static void canvas_cb(gui_canvas_t *canvas)
{
nvgRoundedRect(canvas->vg, 10, 10, 348, 200, 30);
nvgFillColor(canvas->vg, nvgRGB(178,34,34));
nvgFill(canvas->vg);
nvgBeginPath(canvas->vg);
nvgRoundedRect(canvas->vg, 10, 220, 348, 200, 30);
nvgFillColor(canvas->vg, nvgRGB(107,142,35));
nvgFill(canvas->vg);
nvgBeginPath(canvas->vg);
nvgRoundedRect(canvas->vg, 110, 125, 148, 200, 30);
nvgFillColor(canvas->vg, nvgRGBA(30,144,255, 100));
nvgFill(canvas->vg);
}
static void app_ui_design(gui_app_t *app)
{
gui_canvas_t *canvas = gui_canvas_create(&(app->screen), "canvas", 0, 0, 0, 368, 448);
gui_canvas_set_canvas_cb(canvas, canvas_cb);
}
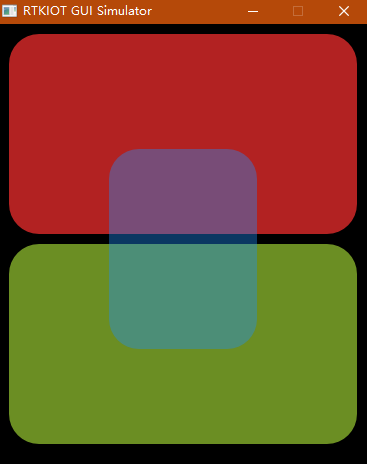
Arc Animation
An example of drawing an arc animation. arc_cb
will be triggered every frame.
#include "math.h"
#include "gui_canvas.h"
static void arc_cb(gui_canvas_t *canvas)
{
static float progress;
progress +=0.01;
nvgArc(canvas->vg, 368/2, 448/2, 150, 0, 3.14*(sinf(progress)+1), NVG_CCW);
nvgStrokeWidth(canvas->vg, 20);
nvgStrokeColor(canvas->vg, nvgRGB(178,34,34));
nvgStroke(canvas->vg);
}
static void app_ui_design(gui_app_t *app)
{
gui_canvas_t *canvas = gui_canvas_create(&(app->screen), "canvas", 0, 0, 0, 368, 448);
gui_canvas_set_canvas_cb(canvas, arc_cb);
}
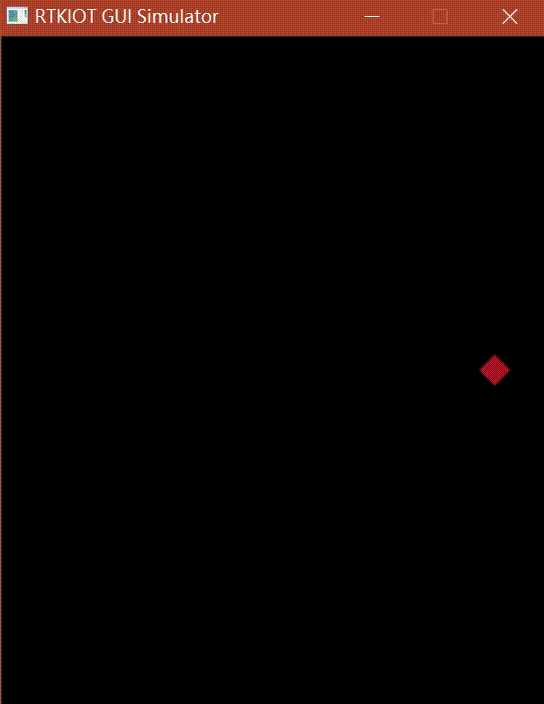
API
Nanovg API
Please refer to
RealGUI API
Defines
-
GUI_CANVAS_OUTPUT_PNG
-
GUI_CANVAS_OUTPUT_JPG
-
GUI_CANVAS_OUTPUT_RGBA
-
GUI_CANVAS_OUTPUT_RGB565
Typedefs
-
typedef void (*gui_canvas_render_function)(NVGcontext *vg)
Functions
-
gui_canvas_t *gui_canvas_create(void *parent, const char *name, void *addr, int16_t x, int16_t y, int16_t w, int16_t h)
create a canvas widget used to drawing graphics in nanovg.
- Parameters:
parent – the father widget nested in.
name – this_widget canvas widget’s name.
addr –
x – the X-axis coordinate relative to parent widget
y – the Y-axis coordinate relative to parent widget
w – width
h – height
- Returns:
gui_canvas_t*
-
void gui_canvas_set_canvas_cb(gui_canvas_t *this_widget, void (*cb)(gui_canvas_t *this_widget))
set the callback function for drawing specific shapes.
- Parameters:
this_widget – this_widget widget pointer
cb – the callback function for drawing specific shapes
-
const uint8_t *gui_canvas_output(int output_format, bool compression, int image_width, int image_height, gui_canvas_render_function renderer)
Renders a canvas and outputs it in the specified format.
This function renders a canvas using the provided renderer function and outputs the result in one of the specified formats. The output can be optionally compressed, depending on the format.
- Parameters:
output_format – The format of the output image. Should be one of the following:
GUI_CANVAS_OUTPUT_PNG
GUI_CANVAS_OUTPUT_JPG
GUI_CANVAS_OUTPUT_RGBA
GUI_CANVAS_OUTPUT_RGB565
compression – Whether to apply compression to the output (applicable for RGBA/RGB565 format).
image_width – The width of the output image.
image_height – The height of the output image.
renderer – A function pointer to the rendering function that takes an NVGcontext. This function is responsible for all drawing operations.
- Returns:
A pointer to the buffer containing the output image data. The format of the data depends on the specified output_format. The user is responsible for managing the memory associated with this buffer.
-
void gui_canvas_output_buffer(int format, bool compression, int image_width, int image_height, gui_canvas_render_function renderer, uint8_t *target_buffer)
Renders a canvas and outputs the result to a target buffer in the specified format.
This function uses the provided renderer function to render a canvas, and then writes the output image to the provided target buffer in the specified format. The output can be optionally compressed, depending on the format.
- Parameters:
format – The format of the output image. Should be one of the following:
GUI_CANVAS_OUTPUT_PNG
GUI_CANVAS_OUTPUT_JPG
GUI_CANVAS_OUTPUT_RGBA
GUI_CANVAS_OUTPUT_RGB565
compression – Whether to apply compression to the output (applicable for RGBA/RGB565 format).
image_width – The width of the output image.
image_height – The height of the output image.
renderer – A function pointer to the rendering function that takes an NVGcontext. This function is responsible for all drawing operations.
target_buffer – A pointer to the buffer where the rendered image data will be written. The buffer must be pre-allocated and large enough to hold the image data in the specified format.
-
NVGcontext *gui_canvas_output_buffer_blank(int format, bool compression, int image_width, int image_height, uint8_t *target_buffer)
Initializes a blank output buffer for the canvas.
This function initializes a NanoVG context with a blank output buffer and returns a pointer to the context. The buffer can be configured with the specified format, compression option, width, and height.
- Parameters:
format – The format of the output buffer.
compression – Boolean flag indicating whether compression should be used.
image_width – The width of the image.
image_height – The height of the image.
target_buffer – Pointer to the target buffer where the output will be stored.
- Returns:
A pointer to the initialized NanoVG context.
-
void gui_canvas_output_buffer_blank_close(NVGcontext *vg)
Closes the canvas and frees resources.
This function closes the NanoVG context created by gui_canvas_output_buffer_blank
- Parameters:
vg – Pointer to the NanoVG context to be closed.
-
struct gui_canvas_t
canvas structure