Page
The page widget is a container widget that allow developers to create a page containing other widgets along the y-axis. After creating a page, various other widgets can be added to it, such as button, image, and more. The page widget can extend beyond the screen display boundaries, and users can access additional widgets added to the page by swiping up or down.
Usage
Create Page Widget
Developers can utilize the gui_page_create()
function to establish a page widget. The page widget is a container oriented vertically, allowing the addition of other widgets to it. The height of the page widget is determined by the number of widgets added to it. As more widgets are added, its height increases.
Example
#include "root_image_hongkong/ui_resource.h"
#include <gui_img.h>
#include "gui_win.h"
#include "gui_text.h"
#include <draw_font.h>
#include "gui_button.h"
#include "gui_page.h"
extern void callback_prism(void *obj, gui_event_t e);
void page_tb_activity(void *parent)
{
gui_img_create_from_mem(parent, "page1", ACTIVITY_BIN, 0, 0, 0, 0);
gui_win_t *win_function = gui_win_create(parent, "win_function", 0, 0, 368, 448);
gui_obj_add_event_cb(win_function, (gui_event_cb_t)callback_prism, GUI_EVENT_TOUCH_LONG, NULL);
gui_page_t *pg1 = gui_page_create(parent, "page", 0, 0, 0, 0);
gui_img_t *img1 = gui_img_create_from_mem(pg1, "img1", BUTTON1_BIN, 0, 150, 0, 0);
gui_img_t *img2 = gui_img_create_from_mem(pg1, "img2", BUTTON2_BIN, 0, 230, 0, 0);
gui_img_t *img3 = gui_img_create_from_mem(pg1, "img3", BUTTON3_BIN, 0, 300, 0, 0);
gui_img_t *img4 = gui_img_create_from_mem(pg1, "img4", PLAYER_MUSIC_REWIND_ICON_BIN, 0, 380, 0, 0);
gui_img_t *img5 = gui_img_create_from_mem(pg1, "img5", PLAYER_MUSIC_WIND_ICON_BIN, 0, 460, 0, 0);
}
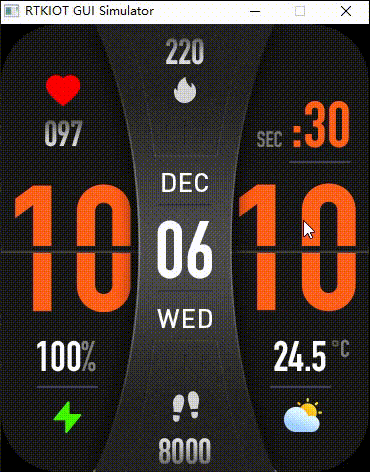
API
Enums
Functions
-
void gui_page_update(gui_obj_t *obj)
update the page widget.
- Parameters:
obj – widget pointer.
-
void gui_page_destroy(gui_obj_t *obj)
destroy the page widget.
- Parameters:
obj – widget pointer.
-
void gui_page_ctor(gui_page_t*, gui_obj_t *parent, const char *name, int16_t x, int16_t y, int16_t w, int16_t h)
construct a page widget.
- Parameters:
this – widget pointer.
parent – the father widget the page nested in.
filename – the page widget name.
x – the X-axis coordinate.
x – the Y-axis coordinate.
w – the width.
h – the hight.
-
gui_page_t *gui_page_create(void *parent, const char *name, int16_t x, int16_t y, int16_t w, int16_t h)
create a page widget.
Example usage
{ char* ptxt = "page_name"; parent = (void *)gui_page_create(parent, ptxt, x, y, w, h); }
- Parameters:
parent – the father widget the page nested in.
filename – the page widget name.
x – the X-axis coordinate.
x – the Y-axis coordinate.
w – the width.
h – the hight.
- Returns:
return the widget object pointer
-
gui_page_t *gui_page_create_horizontal(void *parent, const char *name, int16_t x, int16_t y, int16_t w, int16_t h)
create a page widget horizontal.
- Parameters:
parent – the father widget the page nested in.
filename – the page widget name.
x – the X-axis coordinate.
x – the Y-axis coordinate.
w – the width.
h – the hight.
- Returns:
return the widget object pointer
-
void gui_page_add_scroll_bar(gui_page_t*, void *bar_pic, IMG_SOURCE_MODE_TYPE src_mode)
- Parameters:
this – widget object pointer
bar_pic – bar picture address
src_mode – image source mode, 0 memory and 1 file system
-
void gui_page_set_offset(gui_page_t*, int offset)
- Parameters:
this – widget object pointer
offset – page offset
-
int gui_page_get_offset(gui_page_t*)
- Parameters:
this – widget object pointer
- Returns:
page offset
-
void gui_page_set_animate(gui_page_t*, uint32_t dur, int repeat_count, void *callback, void *p)
- Parameters:
o – widget object pointer
dur – Animation duration
repeat_count – Repeat play times, -1 means play on repeat forever
callback – animate frame callback
p – parameter
-
void gui_page_rebound(gui_page_t*, bool rebound)
config rebound
- Parameters:
this – widget object pointer
rebound – true: config rebound; false: not rebound;
-
void gui_page_rebound_horizontal(gui_page_t*, bool rebound)
config rebound
- Parameters:
this – widget object pointer
rebound – true: config rebound; false: not rebound;
-
void gui_page_center_alignment(gui_page_t *page, int align_height)
automatic center alignment
- Parameters:
page – widget pointer
align_height – page center aligned height
-
void gui_page_set_only_top_slide(gui_page_t *page, bool flag)
set only top slide flag
- Parameters:
page – widget pointer
flag – true:only top slide; false:all slide
-
struct gui_page_t
PAGE widget structure.
Public Members
-
gui_obj_t base
-
uint32_t current_id
-
uint32_t widget_count
-
uint32_t width
-
int yold
-
int16_t recode[5]
-
int speed
-
int target
-
int start_x
-
int start_y
-
int align_hight
-
int get_yend
-
gui_animate_t *animate
-
void (*ctor)(struct gui_page*, gui_obj_t *parent, const char *name, int16_t x, int16_t y, int16_t w, int16_t h)
-
bool release
-
bool press
-
bool gesture_flag
-
bool top_slide_only
-
uint8_t status
-
IMG_SOURCE_MODE_TYPE src_mode
-
uint8_t checksum
-
gui_obj_t base