Prism Mirror 3D Model
This widget supports loading 3D prism mirror models consisting of OBJ and MTL files and supports adding animation effects, which can be displayed on app interfaces. Please read before use: Prism Mirror Widget Usage Notes.
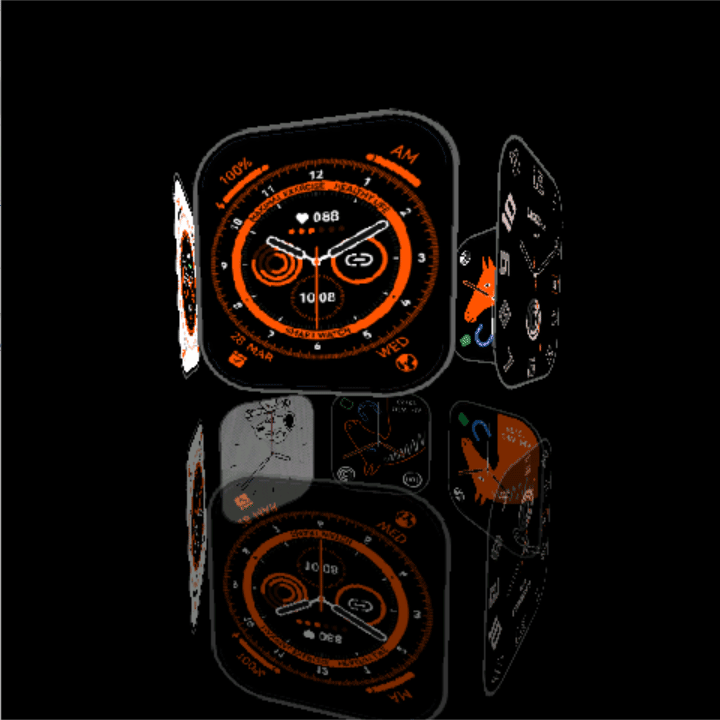
Run the Prism Mirror Widget on the Simulator
HoneyGUI Simulator is based on the scons tool and MinGW-w64 toolchain. It can be run and debugged in VScode. For specific environment setup and running instructions, please refer to the Get Started section.
After completing the environment setup for the HoneyGUI Simulator, when you start running it, you will see the default HoneyGUI project in the simulator.In menu_config.h
( your HoneyGUI dir/win32_sim/menu_config.h
), enable the macro definition CONFIG_REALTEK_BUILD_REAL_PRISM_MIRROR_3D.
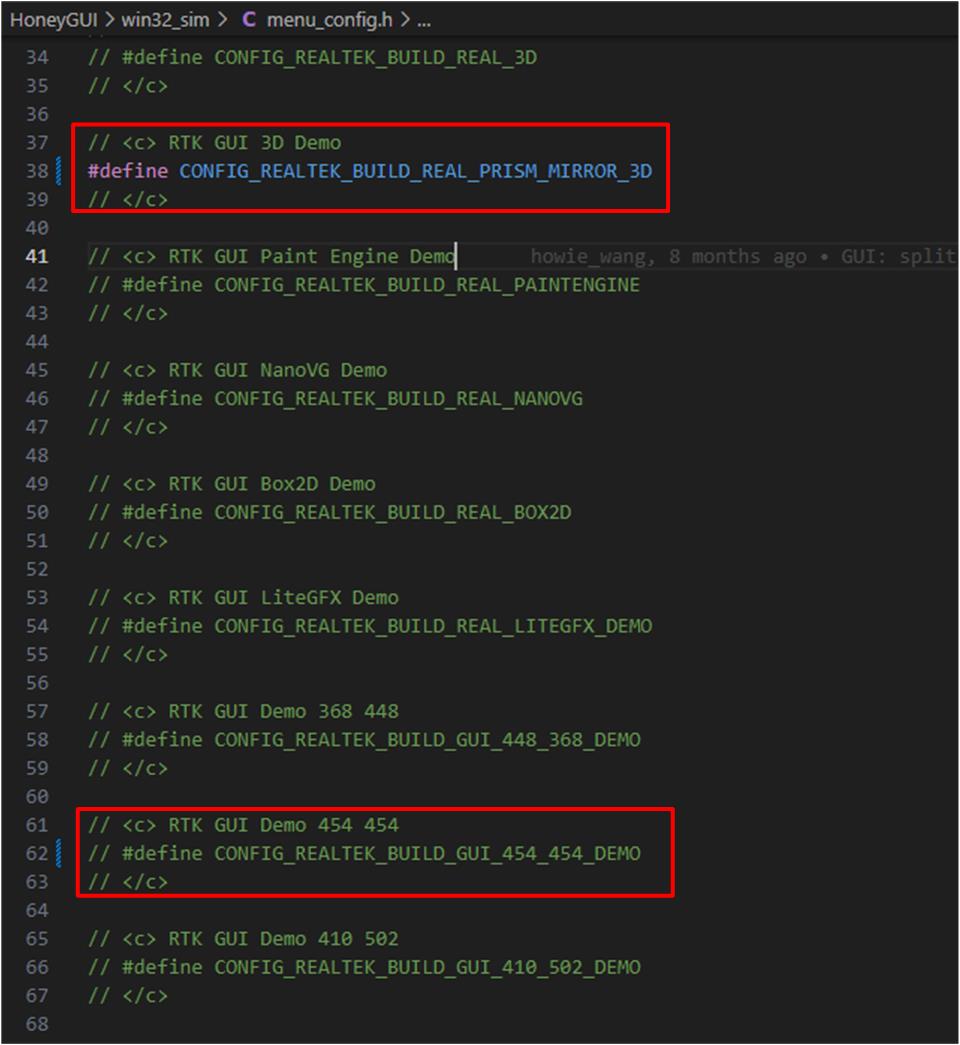
Run the Prism Mirror Widget on the Simulator
If you need to modify the screen size, open the file SConscript
under the directory your HoneyGUI dir/realgui/example/demo/
, and modify the values of DRV_LCD_WIDTH
and DRV_LCD_HEIGHT
to the desired pixel values.
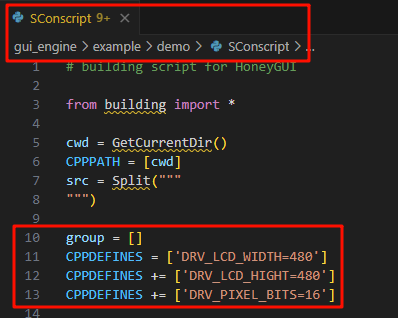
Simulator Changes Screen Size
GUI Load Prism Mirror Model
Add all necessary files for the prism model to a separate folder, including 6 PNG images, geometric information files for the 3D model (
.obj
), and corresponding material information files (.mtl
)OBJ file: Stores geometric data for the prism mirror model, including vertices, normals, texture coordinates, and faces.
MTL file: Describes the material properties of the prism mirror model, including color, glossiness, transparency, and texture mapping.
Image files: Textures used in the model.
Packaged executable file for generating prism info descriptor:
extract_desc.exe
andpng2c.py
.
Parse the prism mirror model and generate prism mirror information descriptor, using a script to process
extract_desc.exe
obj filesIn the prism mirror resource package directory, run the following command through the command line: extract_desc.exe prism.obj. Please modify
prism.obj
to the user’s custom file name as needed.Generate binary array of images and prism mirror information descriptor:
desc.txt
.
GUI Load Descriptor
Place the
desc.txt
file containing obj parsed data, mtl parsed data, and image data into the project directory, and load it ingui_prism_mirror3d_create()
.
Prism Mirror Widget Usage
Create Widget
Create a prism model using the gui_prism_mirror3d_create()
function. This function requires providing a parent object, name, descriptive data, as well as position and size parameters. The number of faces, automatic rotation, and input sensitivity of the prism can be specified through the optional configuration structure (prism_mirror3d_config_t
). The parameter desc
contains parsed data extracted from the script.
Parameters:
parent
: The parent object, the new prism model will be attached to this GUI component.name
: Used as a name to identify and manage 3D objects.desc
: Descriptor pointer, containing visualized parsing data.x
: The x coordinate in the parent component coordinate system.y
: The y coordinate in the parent component coordinate system.w
: The width of the widget.h
: The height of the widget.config
: A pointer to the configuration structure used to configure features such as face count and rotation.
Example:
gui_obj_t *parent = &(app->screen);
const char *name = "prism_demo";
uint16_t x = 100, y = 100, w = 300, h = 300;
prism_mirror3d_config_t config = {6, true, 0.05f};
gui_3d_description_t *desc = gui_get_3d_desc((void *)desc_addr);
gui_prism_mirror3d_t *prism_demo = gui_prism_mirror3d_create(parent, name, desc, x, y, w, h, &config);
Add Dynamic Effects
Use the function gui_prism_mirror3d_enter_animate()
to add dynamic effects to the prism mirror model, such as auto-rotation and interactive rotation. This function takes the created prism_mirror3d
as a parameter, and by default, the model will rotate around the y-axis automatically.
Parameters:
Prism-mirror3d
: A prism model object that has already been created.
Example:
if (prism_demo) {
gui_prism_mirror3d_enter_animate(prism_demo);
}
Add App Switch Effect
Use the function gui_prism_mirror3d_click_switch_app_add_event()
to add click event response effects for the prism mirror model, enabling app switching. The parameter callback
is the corresponding callback function.
Parameters:
Prism-mirror3d
: A prism model object that has already been created, waiting to add a click event.Callback
: A callback function used to handle the application switching logic after a click event.
Example:
void onSwitchAppCallback(gui_event_t e) {
//Handling application switching logic
}
gui_prism_mirror3d_click_switch_app_add_event(prism_demo, onSwitchAppCallback);
Set Size
Set the size of the prism model using gui_prism_mirror3d_det_scale
. Adjust the scaling factor to suit the requirements of the scene.
Parameters:
prism_mirror3d
: The prism mirror object.scale
: The scale factor.
Example:
gui_prism_mirror3d_set_scale(prism_demo, 1.0f);
Set Location
Use gui_prism_mirror3d_det_position
to set the position of the prism model. This function requires x, y. The z-coordinate is used to define the position of the model in 3D space.
Parameters:
prism_mirror3d
: The prism mirror object.x
: The X coordinate.y
: The Y coordinate.z
: The Z coordinate.
Example:
gui_prism_mirror3d_set_position(prism_demo, 0, 50, 0);
Set Orientation
Use gui_prism_mirror3d_set_rotation_angles()
to set the orientation of the prism model. This feature helps adjust the orientation of the model in the 3D environment.
Parameters:
prism_mirror3d
: The prism mirror object.x
: The rotation angle around the X axis.y
: The rotation angle around the Y axis.z
: The rotation angle around the Z axis.
Example:
gui_prism_mirror3d_set_rotation_angles(prism_demo, 0, 60, 0);
Set Original State
Use gui_prism_mirror3d_set_raw_state()
to set the original state of the 3D prism model. This function sets the initial position, camera position, rotation angle, and scaling ratio of the prism in the 3D world. Usually called immediately after the widget is initialized to define the initial display state.
Parameters:
prism-mirror3d
: The prism model object to be configured.world_position
: A floating-point array of length 3, specifying the x of the prism in the world coordinate system, y. Z coordinate.camera_position
: A floating-point array of length 3 that specifies the position of the camera relative to the prism, denoted as x, y. Z coordinate.rot_x
: The rotation angle (in degrees) around the X-axis.rot_y
: The rotation angle around the Y-axis (in degrees).rot_z
: The rotation angle (in degrees) around the Z-axis.scale
: The scaling ratio of a prism.
Example:
float raw_world_position[3] = {0, 25, 180};
float raw_camera_position[3] = {0, 5, 60};
gui_prism_mirror3d_set_raw_state(prism_demo, raw_world_position, raw_camera_position, 0, 0, 0, 13);
Set Target Status
Use gui_prism_mirror3d_set_target_state()
to define the target state that the 3D prism model will achieve in animation or interaction. This feature is particularly suitable for creating smooth transition effects, such as changing from one position to another.
Parameters:
prism-mirror3d
: The prism model object to be adjusted.world_position
: Target world coordinates x, y, z (float array).camera_position
: Array of target position coordinates for the camera, specifying the x, y, z coordinates relative to the prism.rot_x
: The rotation angle of the target around the X-axis.rot_y
: The rotation angle of the target around the Y-axis.rot_z
: The rotation angle of the target around the Z-axis.scale
: The target scaling ratio of a prism.
Example:
float target_world_position[3] = {0, 35, 162};
float target_camera_position[3] = {0, 0, 80};
gui_prism_mirror3d_set_target_state(prism_demo, target_world_position, target_camera_position, 0, 0, 0, 14);
These two functions enable the prism model to have appropriate views and states during initialization, and ensure that the given target state is achieved during animation or interaction, providing users with a smooth visual experience.
Prism Mirror Configuration
For customizing the behavior of the 3D prism mirror, the following adjustments have been made directly to the configuration settings within the code. This section describes the effective parameters used for disabling automatic rotation and setting touch sensitivity.
Automatic Rotation and Sensitivity Settings
The prism mirror is configured with specific parameters to control its basic properties. Here’s an overview of the settings applied:
Number of Faces: Defines the number of visible faces of the prism. This is set to 6 to have a hexagonal prism shape.
Automatic Rotation: By default, the prism mirror rotates automatically. In this configuration, automatic rotation is enabled. However, if you want to disable it, this should be done through additional logic in the application code if required, as the default setting here keeps it active.
Touch Sensitivity: This parameter controls how responsive the prism is to touch inputs. The sensitivity is set to 0.05f, which specifies a medium level of responsiveness, allowing for smooth user interaction.
Configuration Code Set:
prism_demo->conf.auto_rotation = true;
prism_demo->conf.face_nums = 6;
prism_demo->conf.sensitivity = 0.05f;
Configuration Explanation
Face Number: Adjusts the geometric complexity of the prism mirror, currently supports 6-prism effect.
Auto Rotation: Enable or disable the feature where the prism rotates automatically. A value of 1 means the feature is active.
Sensitivity: Controls how the prism responds to touch gestures. Setting this to 0.05f provides a well-balanced responsivenes
Prism Mirror Widget Usage Notes
If you use the current default 3D modeling effect, The default demo effect is a 480 by 480 square display design:
All image resources must be in PNG format.
Replace the demo hexagonal prism effect image. Please change the image name to “image1, image2, …, image6”.
Please obtain the conversion file required for the prism information descriptor from the following path:
File path:
HoneyGUI\realgui\example\demo\3d
, required files:extract_desc.exe
andpng2c.py
.File path:
HoneyGUI\realgui\example\demo\3d
, required files:extract_desc.exe
andpng2c.py
.
If using a display screen of other proportions, in order to achieve better visual effects, it is necessary to re model it using 3D software and export the corresponding OBJ file (reference: Prism Widget to Modify 3D Model). Create a description file that can be loaded into the GUI (reference: GUI Load Prism Mirror Model).
Prism Widget to Modify 3D Model
This routine uses Blender software as a demonstration.
Use 3D modeling software to open the file:
prism.obj
, switch view effects, shortcut key: English mode, press the top left corner of the keyboard ·, Select the view and press Bottom.
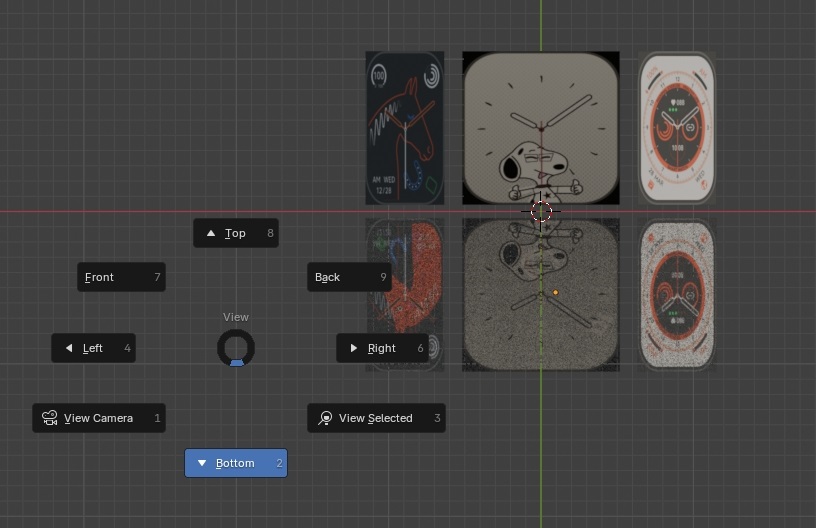
Modify Prism Mirror 3D View
In object mode, select all objects for scaling (taking the 410 * 502 screen as an example, with an aspect ratio of 1:1.224), pull up the object, and the custom scaling will appear in the lower left corner. Modify the y value to 1.224.
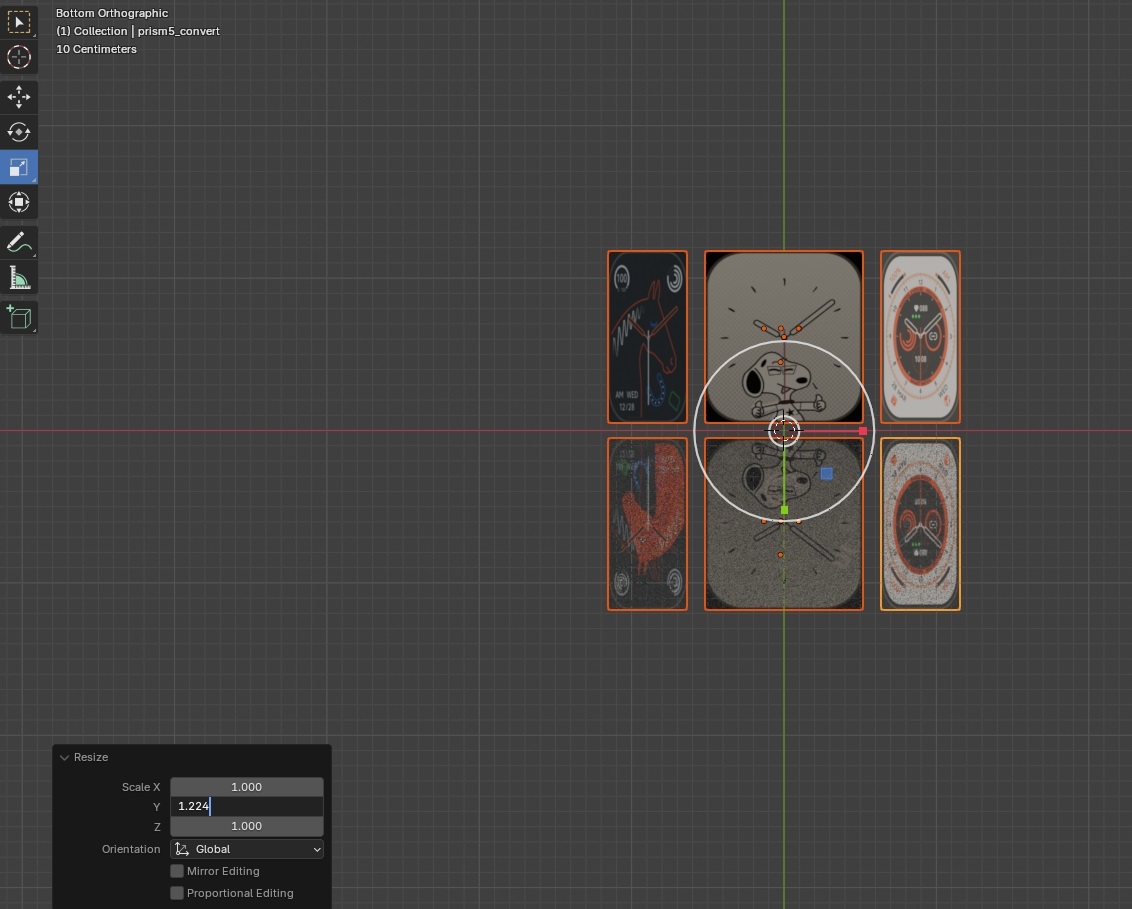
Scale Prism Mirror 3D
Export file
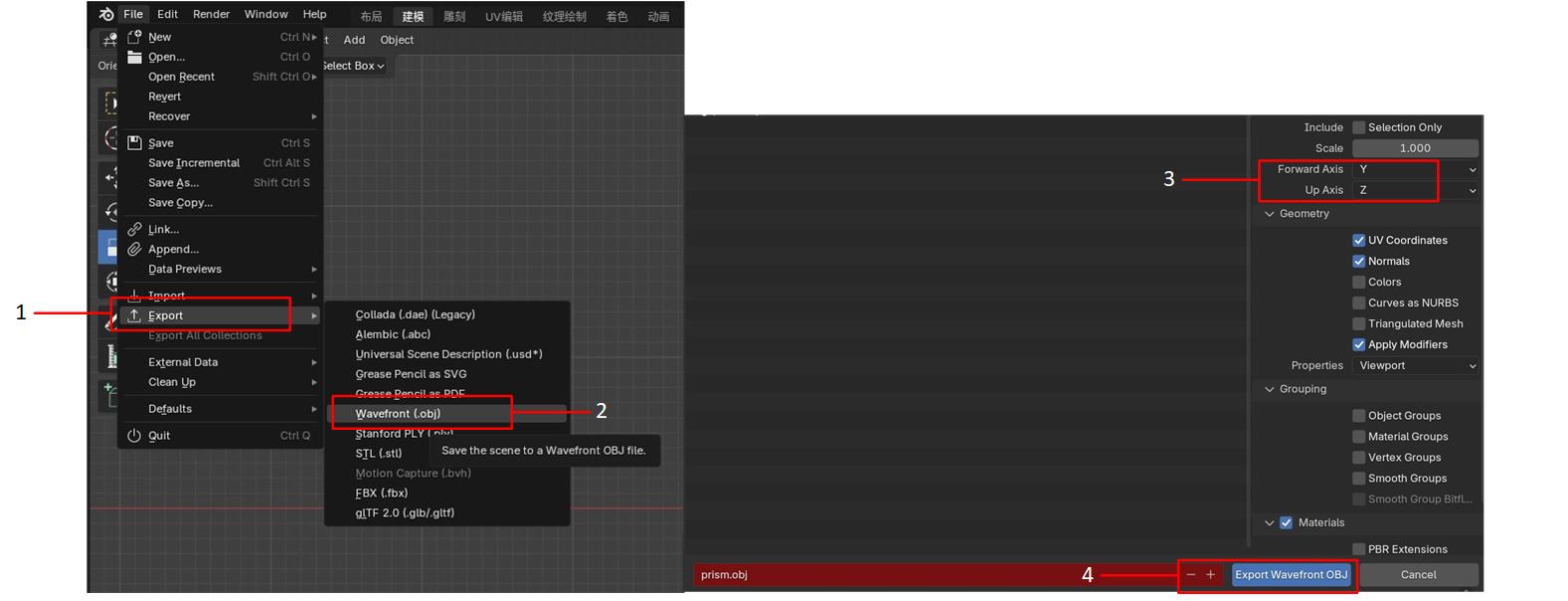
Export Prism Mirror 3D Object Document
Example
Prism Mirror
API
Functions
-
void gui_prism_mirror3d_enter_animate(gui_prism_mirror3d_t *parent)
Enters an animation for the prism mirror.
- Parameters:
parent – The parent prism mirror object.
-
void gui_prism_mirror3d_click_switch_app_add_event(gui_prism_mirror3d_t *prism_mirror3d, gui_event_cb_t callback)
Adds a click switch event to the prism mirror.
- Parameters:
prism_mirror3d – The prism mirror object.
callback – The callback for the event.
-
int16_t *gui_prism_mirror3d_get_enter_face(void)
Gets the currently active face the prism enters.
- Returns:
The active face index.
-
void gui_prism_mirror3d_set_animate(gui_prism_mirror3d_t *prism_mirror3d)
Sets the animation parameters for a prism mirror.
- Parameters:
prism_mirror3d – The prism mirror object.
-
void gui_prism_mirror3d_set_scale(gui_prism_mirror3d_t *prism_mirror3d, float scale)
Sets the scale for a prism mirror.
- Parameters:
prism_mirror3d – The prism mirror object.
scale – The scale factor.
-
void gui_prism_mirror3d_set_position(gui_prism_mirror3d_t *prism_mirror3d, int16_t x, int16_t y, int16_t z)
Sets the position for a prism mirror in 3D space.
- Parameters:
prism_mirror3d – The prism mirror object.
x – The X coordinate.
y – The Y coordinate.
z – The Z coordinate.
-
void gui_prism_mirror3d_set_rotation_angles(gui_prism_mirror3d_t *prism_mirror3d, int16_t x, int16_t y, int16_t z)
Sets the rotation angles for a prism mirror.
- Parameters:
prism_mirror3d – The prism mirror object.
x – The rotation angle around X axis.
y – The rotation angle around Y axis.
z – The rotation angle around Z axis.
-
void gui_prism_mirror3d_set_raw_state(gui_prism_mirror3d_t *prism_mirror3d, float world_position[3], float camera_position[3], float rot_x, float rot_y, float rot_z, float scale)
Sets the raw state of a 3D prism mirror object.
This function initializes or updates the raw state of the given 3D prism mirror object with specified world position, camera position, rotation angles, and scale factor. The raw state typically represents the current scene’s configuration.
- Parameters:
prism_mirror3d – Pointer to the 3D prism mirror object to be modified.
world_position – Array of 3 floats specifying the x, y, z world coordinates of the prism’s position.
camera_position – Array of 3 floats specifying the x, y, z coordinates for the camera’s position relative to the prism.
rot_x – Rotation angle around the x-axis, in degrees.
rot_y – Rotation angle around the y-axis, in degrees.
rot_z – Rotation angle around the z-axis, in degrees.
scale – Scaling factor applied to the prism.
-
void gui_prism_mirror3d_set_target_state(gui_prism_mirror3d_t *prism_mirror3d, float world_position[3], float camera_position[3], float rot_x, float rot_y, float rot_z, float scale)
Sets the target state of a 3D prism mirror object.
This function sets the target configuration for the 3D prism mirror object, allowing it to transition to a desired state defined by the given parameters. The target state typically represents the future or goal configuration for the scene.
- Parameters:
prism_mirror3d – Pointer to the 3D prism mirror object to be adjusted.
world_position – Array of 3 floats specifying the x, y, z world coordinates of the target position for the prism.
camera_position – Array of 3 floats specifying the x, y, z coordinates for the camera’s target position relative to the prism.
rot_x – Rotation angle around the x-axis, in degrees for target orientation.
rot_y – Rotation angle around the y-axis, in degrees for target orientation.
rot_z – Rotation angle around the z-axis, in degrees for target orientation.
scale – Target scaling factor for the prism adjustment.
-
gui_prism_mirror3d_t *gui_prism_mirror3d_create(gui_obj_t *parent, const char *name, void *desc_addr, uint16_t x, uint16_t y, uint16_t w, uint16_t h, prism_mirror3d_config_t *config)
Creates a new prism mirror 3D object.
This function initializes and creates a 3D prism object within the graphical user interface. It sets up the object’s basic properties such as its position, size, and initial transformation states.
- Parameters:
parent – Pointer to the parent GUI object. The created prism object will be a child of this parent, inheriting context and layout.
name – The name identifier for the prism object. This string is used to uniquely identify the object within the GUI environment.
desc_addr – Pointer to the description data for the prism. This can include detailed geometric or material properties for rendering.
x – The X coordinate for the position of the prism within the parent object.
y – The Y coordinate for the position of the prism within the parent object.
w – The width of the prism object.
h – The height of the prism object.
config – Pointer to a configuration structure (prism_mirror3d_config_t). This structure allows for customizing properties such as the number of faces, automatic rotation behavior, and input sensitivity. If NULL, default settings will be applied.
- Returns:
A pointer to the newly created prism mirror 3D object. Returns NULL if the creation fails due to memory allocation issues or invalid parameters.
-
struct prism_mirror3d_config_t
Public Members
-
uint8_t face_nums
Number of faces on the prism. This specifies how many faces the prism should have. A common 3D prism might have 6 faces including front, back, left, right, top, and bottom.
-
bool auto_rotation
Flag indicating whether automatic rotation is enabled. If set to true, the prism will continuously rotate without any user input, allowing for a constant animation effect.
-
float sensitivity
Sensitivity coefficient for user input. This value determines how responsive the prism’s rotation is to user interactions. Values less than 1 reduce the sensitivity, making the prism rotate slower in response to input. Higher values increase sensitivity, resulting in faster rotation.
-
uint8_t face_nums
-
struct gui_prism_transform_state_t
Structure to represent the transform state for a prism.
Public Members
-
gui_point_4d_t worldPosition
The position of the prism in the world coordinate system
-
gui_point_4d_t cameraPosition
The position of the camera observing the prism
-
float rot_x
Rotation around the X axis
-
float rot_y
Rotation around the Y axis
-
float rot_z
Rotation around the Z axis
-
float angle
Angle of rotation
-
float scale
Scale factor
-
gui_point_4d_t worldPosition
-
struct gui_prism_mirror3d_t
Structure to represent a 3D prism mirror widget.
Public Members
-
gui_3d_rect_t render_object
The 3D render object
-
int16_t face_flags_rotation
Flags defining the visibility of faces
-
gui_prism_transform_state_t raw_state
The raw transform state
-
gui_prism_transform_state_t target_state
The target transform state for animations
-
gui_prism_transform_state_t interpolated_state
The interpolated state during animations
-
gui_3d_rect_t render_object