2.5D Soccer
2.5D soccer consists of 12 vertices and 20 faces, which can form an approximately 3D spherical shape. Among them, the vertices define the shape and position of the sphere, and the face is a plane formed by connecting multiple vertices, used to fill the surface of the sphere.
Usage
Create Widget
Use gui_soccer_create()
to create a 2.5D soccer. The addr
parameter is the image used to fill the white faces of the soccer ball.
Set Size
The default distance between the vertices of the soccer is 100. You can change the size of the soccer by using gui_soccer_set_size()
to modify the distance between the vertices.
Set Center
The default center of the soccer is ((dc->fb_width - this->scsize) / 2.0f)
, where this->scsize
is the distance between the vertices of the soccer.
You can change the center coordinates of the soccer by using gui_soccer_set_center()
.
Set Image Mode
The default image blending mode for the soccer is IMG_SRC_OVER_MODE
. You can change the image blending mode by using gui_soccer_set_mode()
.
Set Image Opacity
You can change the opacity of the image by using gui_soccer_set_opacity()
.
Set Image for Soccer Faces
You can set the image for the soccer ball faces by using gui_soccer_set_img()
.
Example
#include "gui_app.h"
#include "gui_soccer.h"
#include "ui_resource.h"
uint32_t *gui_soccer_array[] =
{
SOCCER_P0001_CALL_BIN,
SOCCER_P0002_SPORTS_BIN,
SOCCER_P0003_HEARTRATE_BIN,
SOCCER_P0004_SLEEP_BIN,
SOCCER_P0005_WEATHER_BIN,
SOCCER_P0006_ACTIVITIES_BIN,
SOCCER_P0007_STRESS_BIN,
SOCCER_P0008_SPO2_BIN,
SOCCER_P0009_MUSIC_BIN,
SOCCER_P0010_VOICE_BIN,
SOCCER_P0011_NOTIFICATION_BIN,
SOCCER_P0012_SETTINGS_BIN,
SOCCER_P0013_SPORT_CORECD_BIN,
SOCCER_P0014_MEASURE_BIN,
SOCCER_P0015_MOOD_BIN,
SOCCER_P0016_BREATHE_BIN,
SOCCER_P0017_ALARM_BIN,
SOCCER_P0018_PERIOD_BIN,
SOCCER_P0019_HOME_BIN,
SOCCER_P0020_MORE_BIN,
};
static void app_call_ui_design(gui_app_t *app);
static gui_app_t app_call =
{
.screen =
{
.name = "app_call",
.x = 0,
.y = 0,
},
.ui_design = app_call_ui_design,
.active_ms = 1000 * 5,
};
gui_app_t *get_call_app(void)
{
return &app_call;
}
static void app_call_ui_design(gui_app_t *app)
{
gui_img_create_from_mem(&(app->screen), "call", SOCCER_P0001_CALL_BIN, 100, 100, 100, 100);
}
static void app_soccer_cb(void *obj, gui_event_t e, void *param)
{
gui_soccer_t *soccer = (gui_soccer_t *)obj;
int index = soccer->press_face;
switch (soccer->press_face)
{
case 0:
gui_switch_app(gui_current_app(), get_call_app());
break;
default:
break;
}
}
GUI_APP_ENTRY(APP_SOCCER)
{
gui_soccer_t *soccer = gui_soccer_create(&(app->screen), "soccer", gui_soccer_array, 0, 0);
gui_soccer_set_center(soccer, 227, 227);
gui_soccer_on_click(soccer, app_soccer_cb, NULL);
gui_return_create(GUI_APP_ROOT_SCREEN, gui_app_return_array,
sizeof(gui_app_return_array) / sizeof(uint32_t *), win_cb, (void *)0);
}
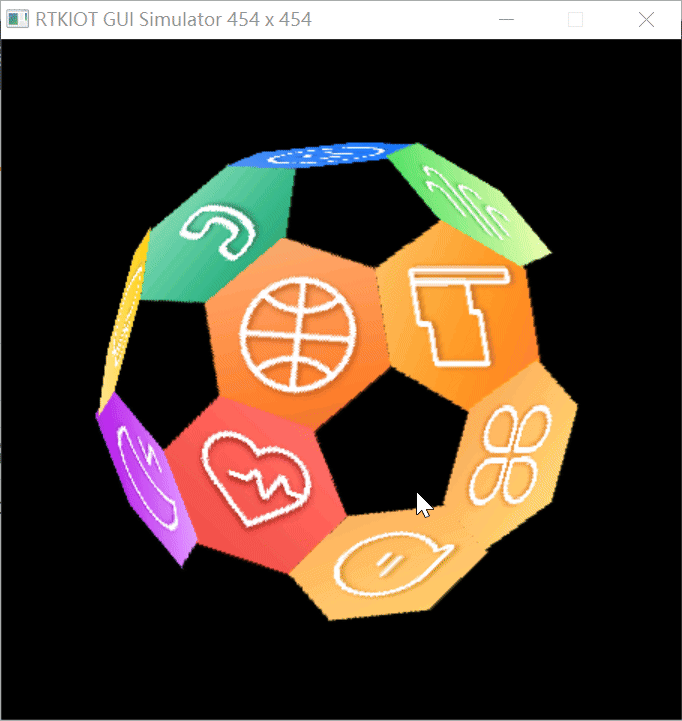
API
Functions
-
gui_soccer_t *gui_soccer_create(void *parent, const char *name, uint32_t *frame_array[], int16_t x, int16_t y)
soccer create, images can be loaded from filesystem or memory address
Example usage
{ gui_soccer_imgfile_t imgfile = { .flg_fs = true, .img_path.img_path_front = "Activity.bin", .img_path.img_path_back = "Weather.bin", .img_path.img_path_up = "HeartRate.bin", .img_path.img_path_down = "Clockn.bin", .img_path.img_path_left = "Music.bin", .img_path.img_path_right = "QuickCard.bin" }; gui_soccer_t *soccer4 = gui_soccer_create(parent, "soccer", &imgfile, 0, 0); gui_soccer_set_size(soccer4, 100); gui_soccer_set_center(soccer4, 200, 200); }
- Parameters:
parent – parent widget
name – widget name
addr – the image file data
x – left
y – top
- Returns:
gui_soccer_t* widget pointer
-
BLEND_MODE_TYPE gui_soccer_get_mode(gui_soccer_t *soccer)
get the soccer image’s blend mode
- Parameters:
soccer – the soccer widget pointer
- Returns:
the soccer image’s blend mode
-
void gui_soccer_set_mode(gui_soccer_t *soccer, BLEND_MODE_TYPE mode)
set the soccer image’s blend mode
- Parameters:
soccer – the soccer widget pointer
mode – the enumeration value of the mode is BLEND_MODE_TYPE
-
uint8_t gui_soccer_get_opacity(gui_soccer_t *soccer)
get the soccer image’s opacity
- Parameters:
soccer – the cube widget pointer
- Returns:
the soccer image’s opacity
-
void gui_soccer_set_opacity(gui_soccer_t *soccer, uint8_t opacity)
set the soccer image’s opacity
- Parameters:
soccer – the soccer widget pointer
opacity – the soccer image’s opacity
-
void gui_soccer_set_img(gui_soccer_t *soccer, uint32_t *frame_array[])
set soccer image
- Parameters:
cube – the soccer widget pointer
addr – the image file data
-
void gui_soccer_set_center(gui_soccer_t *this, float c_x, float c_y)
set center
- Parameters:
this – widget pointer
c_x – center x
c_y – center y
-
void gui_soccer_set_size(gui_soccer_t *this, float size)
set size
- Parameters:
this – widget pointer
size – scale size
-
void gui_soccer_set_slide_range(gui_soccer_t *this, float range)
set slide range
- Parameters:
this – widget pointer
range – slide range
-
void gui_soccer_on_click(gui_soccer_t *this, void *callback, void *parameter)
set on_click event
- Parameters:
this – widget pointer
callback – callback function
parameter – callback function parameter
-
gui_soccer_t *gui_soccer_create_ftl(void *parent, const char *name, uint32_t *frame_array[], int16_t x, int16_t y)
Creates and initializes a new GUI soccer instance (ftl address mode).
This function sets up a new soccer GUI widget. It initializes the necessary components and prepares it for display.
- Parameters:
parent – Pointer to the parent GUI component. This is typically a window or a container that will hold the soccer game widget.
name – A string representing the name of the GUI soccer widget. This can be used for identification purposes within the parent.
frame_array – Array of pointers to uint32_t, representing the frames of the soccer game animation or graphics (ftl address mode).
x – The x-coordinate where the soccer widget will be placed within the parent component’s coordinate system.
y – The y-coordinate where the soccer widget will be placed within the parent component’s coordinate system.
- Returns:
A pointer to the newly created
gui_soccer_t
instance, or NULL if the creation fails due to memory allocation issues or invalid parameters.
-
struct gui_quaternion_t
-
struct gui_soccer_t
SOCCER widget structure.