Window
The widget provides a virtual area for the developer to place application-required widgets. The developer can depend on the requirement to create the space relative to the screen. For example, Figure-1 creates an area the same as the screen dimension, and the developer can create a space with different sizes, as in Figure-2.
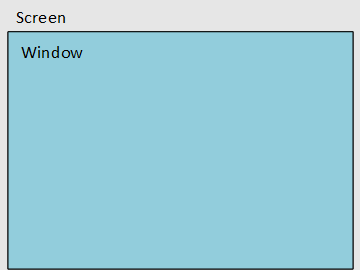
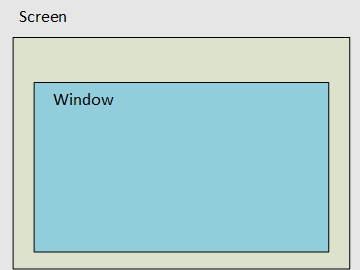
And the following widgets will take the Window’s widget left-top corner as the initial coordinates in the following figure.
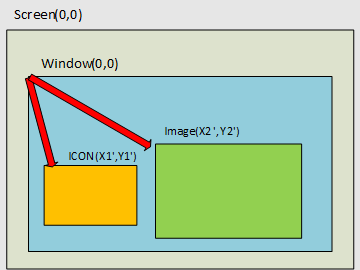
Usage
Create Widget
Create a win widget using this API gui_win_create()
.
Set Animation
Using this API gui_win_set_animate()
to set the animation and implement the animation effect in the corresponding callback function.
Register Callback Function
Register the left/right/up/down slide event of the win widget using the following APIs: gui_win_left()
, gui_win_right()
, gui_win_up()
, gui_win_down()
.
And you can also register the press/release/long press/click event of the win widget by follow APIs: gui_win_press()
, gui_win_release()
, gui_win_long()
, gui_win_click()
.
Set ‘hold_tp’ State
Set the ‘hold_tp’ state by gui_win_hold_tp()
.
Get Progress
Using gui_win_get_animation_progress_percent()
to get the animation progress percentage.
Set Scale Rate
Set the scale rate for the window both horizontally and vertically by gui_win_set_scale_rate()
.
Enable/Disable Scope
Enable or disable the scope of the win widget using gui_win_set_scope()
.
Set Opacity
Set the opacity value for the win widget with gui_win_set_opacity()
.
Animation Check
Check if the animation is at its end frame by gui_win_is_animation_end_frame()
.
Start Animation
Use gui_win_start_animation()
to start the animation.
Stop Animation
Stop the animation with gui_win_stop_animation()
.
Prepare
Win widget preparation in gui_win_prepare()
.
Append An Animation
Using gui_win_append_animate()
to append an animation to a GUI window.
API
Functions
-
gui_win_t *gui_win_create(void *parent, const char *name, int16_t x, int16_t y, int16_t w, int16_t h)
create a window widget.
- Parameters:
parent – the father widget the window nested in.
filename – the window widget name.
x – the X-axis coordinate.
x – the Y-axis coordinate.
w – the width.
h – the hight.
- Returns:
return the widget object pointer
-
void gui_win_set_animate(gui_win_t *_this, uint32_t dur, int repeat_count, gui_animate_callback_t callback, void *p)
set animate.
- Parameters:
_this – widget object pointer.
dur – animation duration.
repeat_count – repeat play times, -1 means play on repeat forever.
callback – animate frame callback.
p – parameter.
-
void gui_win_left(gui_win_t *_this, void *callback, void *parameter)
register a callback function for the left slide event of the win widget.
- Parameters:
_this – win pointer.
callback – callback func.
parameter – callback parameter.
-
void gui_win_right(gui_win_t *_this, void *callback, void *parameter)
register a callback function for the right slide event of the win widget.
- Parameters:
_this – win pointer.
callback – callback func.
parameter – callback parameter.
-
void gui_win_up(gui_win_t *_this, void *callback, void *parameter)
register a callback function for the up slide event of the win widget.
- Parameters:
_this – win pointer.
callback – callback func.
parameter – callback parameter.
-
void gui_win_down(gui_win_t *_this, void *callback, void *parameter)
register a callback function for the down slide event of the win widget.
- Parameters:
_this – win pointer.
callback – callback func.
parameter – callback parameter.
-
void gui_win_press(gui_win_t *_this, gui_event_cb_t callback, void *parameter)
register a callback function for the press event of the win widget.
- Parameters:
_this – win pointer.
callback – callback func.
parameter – callback parameter.
-
void gui_win_release(gui_win_t *_this, gui_event_cb_t callback, void *parameter)
register a callback function for the release event of the win widget.
- Parameters:
_this – win pointer.
callback – callback func (void *obj, gui_event_t e, void *param).
parameter – callback parameter.
-
void gui_win_long(gui_win_t *_this, void *callback, void *parameter)
register a callback function for a long press event of the win widget.
- Parameters:
_this – win pointer.
callback – callback func.
parameter – callback parameter.
-
void gui_win_click(gui_win_t *_this, gui_event_cb_t callback, void *parameter)
register a callback function for a click event of the win widget.
- Parameters:
_this – win pointer.
callback – callback func (void *obj, gui_event_t e, void *param).
parameter – callback parameter.
-
void gui_win_hold_tp(gui_win_t *_this, bool hold_tp)
set the hold_tp state.
- Parameters:
_this – win pointer.
hold_tp – a boolean value to set the state to true or false.
-
float gui_win_get_animation_progress_percent(gui_win_t *win)
get the animation progress percentage.
- Parameters:
win – pointer to the window structure that contains the animation.
- Returns:
the current animation progress percentage.
-
void gui_win_set_scale_rate(gui_win_t *win, float scale_rate_horizontal, float scale_rate_vertical)
set the scale rate for the window both horizontally and vertically.
- Parameters:
win – pointer to the window structure.
scale_rate_horizontal – the horizontal scale rate.
scale_rate_vertical – the vertical scale rate.
-
void gui_win_set_scope(gui_win_t *win, bool enable)
enable or disable the scope for the window.
- Parameters:
win – pointer to the window structure.
enable – a boolean value to enable or disable the scope.
-
void gui_win_set_opacity(gui_win_t *win, unsigned char opacity_value)
set the opacity value for the window.
- Parameters:
win – pointer to the window structure.
opacity_value – the desired opacity value to set.
-
bool gui_win_is_animation_end_frame(gui_win_t *win)
check if the animation is at its end frame.
- Parameters:
win – pointer to the window structure that contains the animation.
- Returns:
true if the end_frame is not 0, false otherwise.
-
void gui_win_start_animation(gui_win_t *win)
start the animation by setting the animate field to 1.
- Parameters:
win – pointer to the window structure that contains the animation. If win or win->animate is NULL, the function will log an error message.
-
void gui_win_stop_animation(gui_win_t *win)
stop the animation by setting the animate field to 0.
- Parameters:
win – pointer to the window structure that contains the animation. If win or win->animate is NULL, the function will log an error message.
-
void gui_win_prepare(gui_obj_t *obj)
window widget prepare.
- Parameters:
obj – pointer.
-
void gui_win_append_animate(gui_win_t *win, uint32_t dur, int repeat_count, void *callback, void *p, const char *name)
append an animation to a GUI window.
This function appends an animation to the specified GUI window. The animation will run for the specified duration, repeat the specified number of times, and call the provided callback function at each frame.
Note
The
callback
function should match the expected signature for animation callbacks in the GUI library being used. Thep
parameter allows the passing of additional data to the callback function.- Parameters:
win – pointer to the GUI window object.
dur – duration of the animation in milliseconds.
repeat_count – number of times the animation should repeat.
callback – function to be called at each frame.
p – user data to be passed to the callback function.
name – aniamte name.
-
void gui_win_move(gui_win_t *win, int x, int y)
Move the GUI window to the specified coordinates.
This function repositions a GUI window to the specified (x, y) coordinates on the screen.
- Parameters:
win – Pointer to the GUI window that needs to be moved.
x – The new x-coordinate for the window.
y – The new y-coordinate for the window.
-
struct gui_win_t
window structure