Timeout
This sample uses ENHTIM to generate a timeout interrupt every second.
To facilitate observation, connect the P0_0 pin to LED0 on the EVB board.
When the timer reaches 1 second, an interrupt will be triggered. In the interrupt handler function, the program will toggle the level of the P0_0 pin, changing the output polarity of the GPIO, thereby making LED0 blink once every second.

Enhance-Timer timeout diagram
Users can modify pin information, timing, and more through different macro configurations. For detailed macro configurations, refer to Configurations.
Requirements
For requirements, please refer to the Requirements.
Wiring
Connect P0_0 to LED0 on the EVB.
The LED driver circuit is shown below.
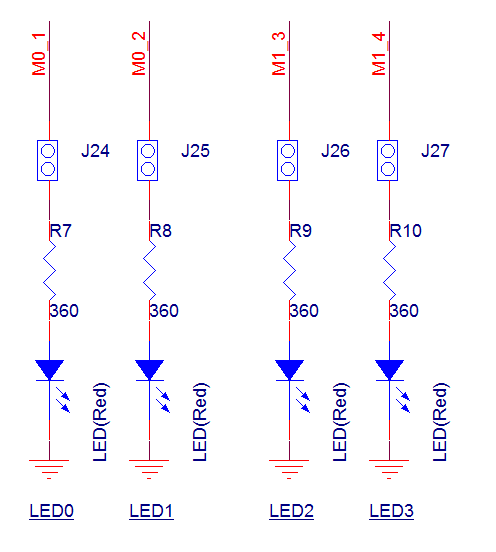
LED Driver Circuit Diagram
Configurations
The following macro can be configured to modify the pin definition.
#define OUTPUT_PIN P0_0 #define GPIO_PIN GPIO_GetPin(OUTPUT_PIN) #define GPIO_PORT GPIO_GetPort(OUTPUT_PIN)
The following macro can be configured to modify the timing.
#define ENHTIM_UINT 40 /*< 40M source clock is divided to get unit: 1us */ #define ENHTIM_ING 1000000 /*< Set this macro to 1000000, with a timer duration of 1s. the unit for this macro is in microseconds (us). */ #define ENHTIM_PERIOD ENHTIM_ING * ENHTIM_UINT
Building and Downloading
For building and downloading, please refer to the Building and Downloading.
Experimental Verification
When the EVB starts, observe the following log within the Debug Analyzer
Start enhtim interrupt test!
After enabling ENHTIM, when each timer interval reaches 1 second, it will trigger the ENHTIM interrupt. Within the interrupt function, the output level of P0_0 is toggled, and LED0 can be observed to blink once every second.
Code Overview
This section mainly introduces the code and process description for initialization and corresponding function implementation in the sample.
Source Code Directory
The directory for project file and source code are as follows:
Project directory:
sdk\samples\peripheral\enhtimer\enhtim_interrupt\proj
Source code directory:
sdk\samples\peripheral\enhtimer\enhtim_interrupt\src
Initialization
The initialization flow for peripherals can refer to Initialization Flow in General Introduction.
Call
Pad_Config()
andPinmux_Config()
to configure the PAD and PINMUX of the corresponding pins.void board_gpio_init(void) { Pad_Config(OUTPUT_PIN, PAD_PINMUX_MODE, PAD_IS_PWRON, PAD_PULL_NONE, PAD_OUT_ENABLE, PAD_OUT_HIGH); Pinmux_Config(OUTPUT_PIN, DWGPIO); }
Call
RCC_PeriphClockCmd()
to enable the GPIO clock.Initialize the GPIO peripheral:
Define the
GPIO_InitTypeDef
typeGPIO_InitStruct
, and callGPIO_StructInit()
to pre-fillGPIO_InitStruct
with default values.Modify the
GPIO_InitStruct
parameters as needed. The initialization parameter configurations for GPIO are shown in the table below. CallGPIO_Init()
to initialize the GPIO peripheral.
GPIO Hardware Parameters |
Setting in the |
GPIO |
---|---|---|
GPIO pin |
|
|
GPIO direction |
||
GPIO interrupt |
Call
RCC_PeriphClockCmd()
to enable the ENHTIM clock.Initialize the ENHTIM peripheral:
Define the
ENHTIM_InitTypeDef
typeENHTIM_InitStruct
, and callENHTIM_StructInit()
to pre-fillENHTIM_InitStruct
with default values.Modify the
ENHTIM_InitStruct
parameters as needed. The initialization parameter configurations for ENHTIM are shown in the table below. CallENHTIM_Init()
to initialize the ENHTIM peripheral.Call
NVIC_Init()
to configure the NVIC. For NVIC-related configurations, refer to Interrupt Configuration.Call
ENHTIM_ClearINTPendingBit()
andENHTIM_INTConfig()
to clear the ENHTIM interrupt and enable the ENHTIM interrupt.
ENHTIM Hardware Parameters |
Setting in the |
ENHTIM |
---|---|---|
Counter mode |
||
PWM mode |
||
Count value |
|
Call
ENHTIM_Cmd()
to enable the TIM peripheral.
Functional Implementation
After enabling the ENHTIM peripheral, ENHTIM starts timing. When the timer of ENH_TIM0 reaches its specified timing, it triggers an interrupt, and the program enters the interrupt handling function Enhanced_Timer0_Handler
.
Clear the interrupt flag of ENH_TIM0 and disable ENH_TIM0.
Check the current state of the LED, and toggle the level of LED0.
void Enhanced_Timer0_Handler()
{
ENHTIM_ClearINTPendingBit(ENHTIMER_NUM, ENHTIM_INT_TIM);
ENHTIM_Cmd(ENHTIMER_NUM, DISABLE);
if (!LED_Status)
{
GPIO_WriteBit(GPIO_PORT, GPIO_PIN, (BitAction)(1));
LED_Status = 1;
}
else
{
GPIO_WriteBit(GPIO_PORT, GPIO_PIN, (BitAction)(0));
LED_Status = 0;
}
//Add user code here
ENHTIM_Cmd(ENHTIMER_NUM, ENABLE);
}