Application switching
Application switching animation sample
The first app has a list, and the second app has a grid.
Clicking on the first app’s button will switch to the second app.
The first app will gradually become transparent until it disappears when switching, and the second app will enlarge to fill the screen.
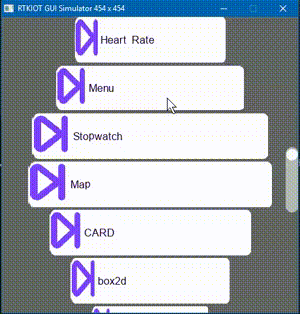
Source code
In the animation frame callback, implement the animation effect of app switching (scaling and fading).
When the animation is finished, shut down the app.
void app_watch_mune_win_ani_cb(void *args, gui_win_t *win)
{
// Calculate the progress percentage of the animation
float pro = gui_win_get_aniamtion_progress_percent(win);
// Adjust the scale of the window based on sine function of the progress percentage
// This will create a smooth scaling effect as the progress advances
gui_win_set_scale_rate(win, 1 + sinf(pro * M_PI / 2), 1 + sinf(pro * M_PI / 2));
// Ensure the scope property is set to 1 (used to indicate visibility)
gui_win_set_scope(win, 1);
// Set the opacity of the window's base GUI element, gradually decreasing as the animation progresses
// This creates a fading effect by setting opacity inversely proportional to the progress percentage
gui_win_set_opacity(win, (1.0f - pro) * UINT8_MAX);
// Check if the animation has reached its last frame
if (gui_win_is_animation_end_frame(win))
{
// Shutdown the GUI application
gui_app_shutdown(get_app_watch_ui());
}
}
#define MENU_WIN_NAME "menu win name"
void design_tab_menu(void *parent)
{
gui_win_t *win = gui_win_create(parent, MENU_WIN_NAME, 0, 0, 0, 0);
gui_win_set_animate(win, 2000, 0, app_watch_mune_win_ani_cb, 0);
gui_win_stop_animation(win);//aniamtion start to play until button click event
The next app’s animation starts playing on app starting up.
#include "gui_menu_cellular.h"
#include "math.h"
static void menu_win_ani_cb(void *args, gui_win_t *win)
{
float pro = gui_win_get_aniamtion_progress_percent(win);
gui_win_set_scale_rate(win, sinf(pro * (M_PI / 2 - 0.2f) + 0.2f),
sinf(pro * (M_PI / 2 - 0.2f) + 0.2f));
gui_win_set_opacity(win, (pro) * UINT8_MAX);
if (gui_win_is_animation_end_frame(win))
{
gui_win_set_scale_rate(win, 0, 0);//reset scale
}
}
#define APP_WATCH_WIN_NAME "menu win name"
static void menu_win_ani_cb_return(void *args, gui_win_t *win)
{
float pro = gui_win_get_aniamtion_progress_percent(win);
gui_win_set_opacity(win, (1.0f - pro) * UINT8_MAX);
gui_win_set_scale_rate(win, cosf(pro * M_PI / 2), cosf(pro * M_PI / 2));
if (gui_win_is_animation_end_frame(win))
{
GUI_APP_SHUTDOWN(APP_MENU)
}
}
static void app_wathc_win_ani_cb_return(void *args, gui_win_t *win)
{
float pro = gui_win_get_aniamtion_progress_percent(win);
gui_win_set_opacity(win, (pro) * UINT8_MAX);
gui_win_set_scale_rate(win, 1 + cosf(pro * M_PI / 2), 1 + cosf(pro * M_PI / 2));
if (gui_win_is_animation_end_frame(win))
{
/*Overwrite app watch's aniamtion as the shut down animation*/
extern void app_watch_mune_win_ani_cb(void *args, gui_win_t *win);
gui_win_set_animate(win, APP_SWAP_ANIMATION_DUR, 0, app_watch_mune_win_ani_cb,
0);
gui_win_stop_animation(win);
}
}
#define APP_MENU_WIN_NAME "APP_MENU_WIN_NAME"
static void app_menu_win_cb(gui_obj_t *this)//this widget, event code, parameter
{
gui_app_layer_buttom();
gui_app_startup(get_app_watch_ui());
gui_tabview_t *tabview = 0;
gui_obj_tree_get_widget_by_name(&(get_app_watch_ui()->screen), "tabview", (void *)&tabview);
if (tabview)
{
gui_tabview_jump_tab(tabview, -1, 0);
}
{
gui_win_t *win = 0;
gui_obj_tree_get_widget_by_name(&(get_app_watch_ui()->screen), APP_WATCH_WIN_NAME, (void *)&win);
if (win)
{
/*Overwrite app watch's aniamtion as the start animation*/
gui_win_set_animate(win, APP_SWAP_ANIMATION_DUR, 0, app_wathc_win_ani_cb_return,
0);
}
}
gui_win_t *win = 0;
gui_obj_tree_get_widget_by_name(&(GUI_APP_HANDLE(APP_MENU)->screen), APP_MENU_WIN_NAME,
(void *)&win);
if (win)
{
gui_win_set_animate(win, APP_SWAP_ANIMATION_DUR, 0, menu_win_ani_cb_return,
0);
}
}
static void app_menu_cb(void *obj, gui_event_t e, void *param)
{
gui_log("%d,%x\n", GUI_TYPE(gui_obj_t, obj)->type, param);
}
/*Define APP_MENU's entry func */
static void app_menu(gui_app_t *app)
{
/**
* @link https://docs.realmcu.com/Honeygui/latest/widgets/gui_menu_cellular.html#example
*/
gui_win_t *win = gui_win_create(GUI_APP_ROOT_SCREEN, APP_MENU_WIN_NAME, 0, 0, 0, 0);
gui_win_set_animate(win, APP_SWAP_ANIMATION_DUR, 0, menu_win_ani_cb,
0);//aniamtion start to play at app startup
The first app’s animation starts playing on click event.
In the button click callback, execute the next app startup.
static void menu_cb(gui_obj_t *obj)
{
// Retrieve the window object with the name specified by MENU_WIN_NAME from the root of the GUI tree
gui_win_t *win = 0;
gui_obj_tree_get_widget_by_name(gui_get_root(obj), MENU_WIN_NAME, (void *)&win);
// If the window object is found, enable its animation by setting the animate flag to 1
if (win)
{
win->animate->animate = 1;
}
GUI_APP_STARTUP(APP_MENU); // Start up the menu application(NEXT APP)
// Bring the next application layer to the top, ensuring it's displayed above the first app layer
gui_app_layer_top();
}