Tabview
The gui_tabview widget is the container to store and depends on user selection to change the tab for different functions. As in Figure-1, the “Green Area” represents the actual display region, and there’re five rectangles with the red dashed line as the whole gui_tabview area. And each rectangle is the gui_tab widget. The “idx” stands for the index on the X-axis, and the “idy” stands for the index on Y-axis.
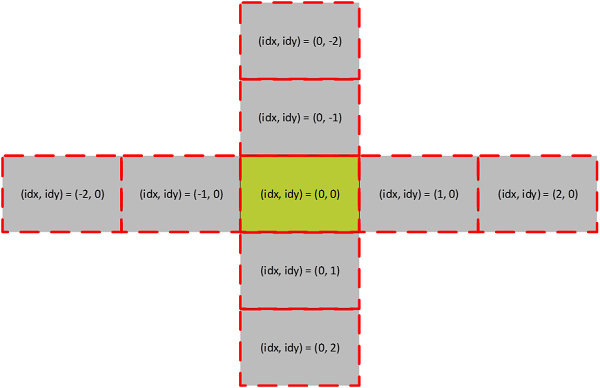
Usage
Create tabview widget
If developers need to construct a container to house tabs, they may utilize the gui_tabview_create(parent, filename, x, y, w, h) function to establish a tabview.
Switch the gui_tab
gui_tabview_jump_tab(parent_tabview, idx, idy) is used to set up a jump to a specified tab, and through this function, users can quickly locate the desired tab.
Set tabview style
Developers can use gui_tabview_set_style(this, style) to set the desired style of tabview. By default, the classic style is employed. The styles are illustrated in the subsequent enumeration.
typedef enum t_slide_style
{
CLASSIC = 0x0000,
REDUCTION = 0x0001,
FADE = 0x0002,
REDUCTION_FADE = 0x0003,
STACKING = 0x0004,
TAB_ROTATE = 0x0005,
TAB_CUBE = 0x0006,
TAB_PAGE = 0x0007,
} T_SLIDE_STYLE;
Set tabview loop
The Tabview loop function is a clever feature that allows users to easily navigate through all the tabs that have been created. By enabling the reverse loop display function using gui_tabview_loop_x(tabview, loop) for the x-axis and gui_tabview_loop_y(tabview, loop) for the y-axis, as shown in Figure-2, you will see a blue outer box indicating the visible area of the screen for the tab control. The direction of the arrow represents the direction of tab switching. When swiping left, once all tabs have moved to the left side of the visible area, another left swipe will cycle back to the leftmost tab that was created. Conversely, as shown in Figure-3, when swiping right again, the display will cycle from the rightmost tab.
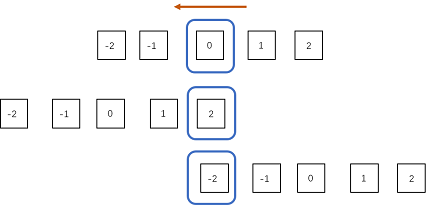
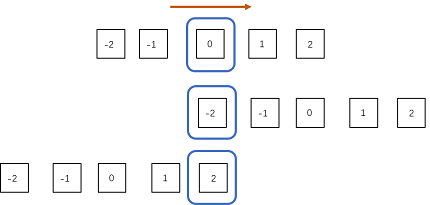
Example
Please refer to the page below:
API
Enums
Functions
-
gui_tabview_t *gui_tabview_create(void *parent, const char *name, int16_t x, int16_t y, int16_t w, int16_t h)
create a tabview widget, which can nest tabs.
- Parameters:
parent – the father widget it nested in.
filename – this tabview widget’s name.
x – the X-axis coordinate relative to parent widget
y – the Y-axis coordinate relative to parent widget
w – width
h – height
- Returns:
return the widget object pointer.
-
void gui_tabview_jump_tab(gui_tabview_t *parent_tabview, int8_t idx, int8_t idy)
jump to a specify tab
- Parameters:
parent_tabview – tabview pointer
idx – Horizontal index value
idy – Vertical index value
-
void gui_tabview_set_style(gui_tabview_t *this, T_SLIDE_STYLE style)
config slide effect
- Parameters:
this – tabview pointer
style – refer to T_SLIDE_STYLE
-
void gui_tabview_loop_x(gui_tabview_t *tabview, bool loop)
Config tabview loop_x sliding feature. The default setting is no looping.
- Parameters:
tabview – tabview pointer.
loop – Loops when set to true, does not loop when set to false.
-
void gui_tabview_loop_y(gui_tabview_t *tabview, bool loop)
Config tabview loop_y sliding feature. The default setting is no looping.
- Parameters:
tabview – tabview pointer.
loop – Loops when set to true, does not loop when set to false.
-
void gui_tabview_tp_disable(gui_tabview_t *tabview, bool disable_tp)
disable tp action for this tabview.
- Parameters:
tabview – tabview pointer.
disable_tp – true: disable tp action, false: enable tp action.
-
void gui_tabview_enable_pre_load(gui_tabview_t *this, bool enable)
enable tab widget cache
- Parameters:
this –
cache –
-
void gui_tabview_tab_change(gui_tabview_t *tabview, void *callback, void *parameter)
Register callback for tab change.
- Parameters:
tabview – tabview pointer.
callback – callback func.
parameter – callback parameter.
-
struct gui_tabview_tab_id_t
- #include <gui_tabview.h>
-
struct gui_index_t
- #include <gui_tabview.h>
-
struct gui_jump_t
- #include <gui_tabview.h>
-
struct gui_tabview_t
- #include <gui_tabview.h>
tabview structure
Public Members
-
uint16_t tab_cnt
-
int8_t tab_cnt_left
-
int8_t tab_cnt_right
-
int8_t tab_cnt_up
-
int8_t tab_cnt_down
-
gui_tabview_tab_id_t cur_id
-
gui_jump_t jump
-
T_SLIDE_STYLE style
-
int16_t release_x
-
int16_t release_y
-
uint8_t loop_x
-
uint8_t loop_y
-
bool enable_pre_load
-
bool tab_change_ready
-
bool tab_need_pre_load
-
bool tp_disable
-
bool initial
-
uint8_t *left_shot
-
uint8_t *center_shot
-
uint8_t *right_shot
-
uint8_t checksum
-
uint16_t tab_cnt