Tab
The tab widget enables you to create an unlimited number of tabs in both the x and y axes of the screen.Before integrating the tab widget, you must first establish a tabview widget to house the tabs.The direction of the generated tabs is denoted by idx and idy.
For an introduction to idx and idy, please refer to:
Usage
Create tab widget
gui_tab_create(parent, x, y, w, h, idx, idy) is used to create a tab widget, where idx and idy represent the direction of the created tab.
Set tab style
If you wish to exhibit various switching effects when switching tabs, you can utilize the gui_tab_set_style(this, style) setting. By default, the classic style is employed. The styles are illustrated in the subsequent enumeration.
typedef enum t_slide_style
{
CLASSIC = 0x0000,
REDUCTION = 0x0001,
FADE = 0x0002,
REDUCTION_FADE = 0x0003,
STACKING = 0x0004,
TAB_ROTATE = 0x0005,
TAB_CUBE = 0x0006,
TAB_PAGE = 0x0007,
} T_SLIDE_STYLE;
Example
Example for tab
The tab switching style can be customized, and developers can set the tab switching style using the function gui_tabview_set_style(this, style). Available styles include REDUCTION
, CLASSIC
, FADE
, REDUCTION_FADE
, TAB_ROTATE
, TAB_CUBE
, and TAB_PAGE
. The FADE
style adjusts the tab’s opacity during the switch, while the REDUCTION_FADE
style changes both the size and opacity of the tab during the transition. In this example, the first three tabs are set to the TAB_CUBE
style, and the last three tabs are set to the REDUCTION
style, as shown in the animation below. Developers can modify the tab switching style by calling gui_tab_set_style(this, style) for different tabs.
#include <gui_tabview.h>
#include "gui_card.h"
#include <gui_obj.h>
#include <gui_win.h>
#include <gui_text.h>
#include <gui_curtain.h>
#include "root_image_hongkong/ui_resource.h"
#include <gui_app.h>
#include "gui_tab.h"
#include "app_hongkong.h"
#include "gui_win.h"
#include "gui_server.h"
#include "gui_components_init.h"
#include <stdio.h>
static void app_hongkong_ui_design(gui_app_t *app)
{
gui_log("app_hongkong_ui_design\n");
gui_tabview_t *tv = gui_tabview_create(&(app->screen), "tabview", 0, 0, 0, 0);
gui_win_t *win = gui_win_create(&(app->screen), "window", 0, 0, 0, 0);
gui_obj_add_event_cb(win, (gui_event_cb_t)kb_button_cb, GUI_EVENT_KB_UP_PRESSED, NULL);
gui_tabview_enable_pre_load(tv, true);
gui_tab_t *tb_clock = gui_tab_create(tv, "tb_clock", 0, 0, 0, 0, 0, 0);
gui_tab_t *tb_activity = gui_tab_create(tv, "tb_activity", 0, 0, 0, 0, 1, 0);
gui_tab_t *tb_heart = gui_tab_create(tv, "tb_heart", 0, 0, 0, 0, 2, 0);
gui_tab_t *tb_cube = gui_tab_create(tv, "tb_cube", 0, 0, 0, 0, 3, 0);
gui_tab_t *tb_weather = gui_tab_create(tv, "tb_weather", 0, 0, 0, 0, 5, 0);
gui_tab_t *tb_music = gui_tab_create(tv, "tb_music", 0, 0, 0, 0, 4, 0);
gui_tab_t *tb_ani = gui_tab_create(tv, "tb_ani", 0, 0, 0, 0, 6, 0);
page_tb_clock(gui_tab_get_rte_obj(tb_clock));
page_tb_activity(gui_tab_get_rte_obj(tb_activity));
page_tb_heart(gui_tab_get_rte_obj(tb_heart));
page_tb_cube(gui_tab_get_rte_obj(tb_cube));
page_tb_weather(gui_tab_get_rte_obj(tb_weather));
page_tb_music(gui_tab_get_rte_obj(tb_music));
gui_tab_set_style(tb_clock, TAB_CUBE);
gui_tab_set_style(tb_activity, TAB_CUBE);
gui_tab_set_style(tb_heart, TAB_CUBE);
gui_tab_set_style(tb_cube, REDUCTION);
gui_tab_set_style(tb_weather, REDUCTION);
gui_tab_set_style(tb_music, REDUCTION);
}

Example for tabview rotate
Unlike individual tab style changes, the tabview control allows you to set a uniform switching style for all tabs using the function gui_tabview_set_style(this, style). For example, you can set all tabs to styles such as REDUCTION
, CLASSIC
, FADE
, REDUCTION_FADE
, TAB_ROTATE
, TAB_CUBE
, or TAB_PAGE
. The following example demonstrates how to switch to the TAB_ROTATE
style, with CLASSIC
being the default style. Developers can use the gui_tabview_set_style(this, style) function to set the desired switching style for all tabs.
#include <gui_tabview.h>
#include "gui_card.h"
#include <gui_obj.h>
#include <gui_win.h>
#include <gui_text.h>
#include <gui_curtain.h>
#include "root_image_hongkong/ui_resource.h"
#include <gui_app.h>
#include "gui_tab.h"
#include "app_hongkong.h"
#include "gui_win.h"
#include "gui_server.h"
#include "gui_components_init.h"
#include <stdio.h>
static void app_hongkong_ui_design(gui_app_t *app)
{
gui_log("app_hongkong_ui_design\n");
gui_tabview_t *tv = gui_tabview_create(&(app->screen), "tabview", 0, 0, 0, 0);
gui_win_t *win = gui_win_create(&(app->screen), "window", 0, 0, 0, 0);
gui_obj_add_event_cb(win, (gui_event_cb_t)kb_button_cb, GUI_EVENT_KB_UP_PRESSED, NULL);
gui_tabview_set_style(tv, TAB_ROTATE);
gui_tabview_enable_pre_load(tv, true);
gui_tab_t *tb_clock = gui_tab_create(tv, "tb_clock", 0, 0, 0, 0, 0, 0);
gui_tab_t *tb_activity = gui_tab_create(tv, "tb_activity", 0, 0, 0, 0, 1, 0);
gui_tab_t *tb_heart = gui_tab_create(tv, "tb_heart", 0, 0, 0, 0, 2, 0);
gui_tab_t *tb_cube = gui_tab_create(tv, "tb_cube", 0, 0, 0, 0, 3, 0);
gui_tab_t *tb_weather = gui_tab_create(tv, "tb_weather", 0, 0, 0, 0, 5, 0);
gui_tab_t *tb_music = gui_tab_create(tv, "tb_music", 0, 0, 0, 0, 4, 0);
gui_tab_t *tb_ani = gui_tab_create(tv, "tb_ani", 0, 0, 0, 0, 6, 0);
page_tb_clock(gui_tab_get_rte_obj(tb_clock));
page_tb_activity(gui_tab_get_rte_obj(tb_activity));
page_tb_heart(gui_tab_get_rte_obj(tb_heart));
page_tb_cube(gui_tab_get_rte_obj(tb_cube));
page_tb_weather(gui_tab_get_rte_obj(tb_weather));
page_tb_music(gui_tab_get_rte_obj(tb_music));
}

Example for tabview loop
In a tabview, you can use the function gui_tabview_loop_x(tabview, loop) (refer to tabview) to determine whether the tabs should loop continuously in the x-direction. Similarly, gui_tabview_loop_y(tabview, loop) determines whether the tabs should loop continuously in the y-direction. The loop parameter is a boolean that specifies whether to enable the looping feature. If set to true, the tabs will loop continuously; if set to false, they will not.
#include <gui_tabview.h>
#include "gui_card.h"
#include <gui_obj.h>
#include <gui_win.h>
#include <gui_text.h>
#include <gui_curtain.h>
#include "root_image_hongkong/ui_resource.h"
#include <gui_app.h>
#include "gui_tab.h"
#include "app_hongkong.h"
#include "gui_win.h"
#include "gui_server.h"
#include "gui_components_init.h"
#include <stdio.h>
static void app_hongkong_ui_design(gui_app_t *app)
{
gui_log("app_hongkong_ui_design\n");
gui_tabview_t *tv = gui_tabview_create(&(app->screen), "tabview", 0, 0, 0, 0);
gui_win_t *win = gui_win_create(&(app->screen), "window", 0, 0, 0, 0);
gui_obj_add_event_cb(win, (gui_event_cb_t)kb_button_cb, GUI_EVENT_KB_UP_PRESSED, NULL);
gui_tabview_set_style(tv, TAB_CUBE);
gui_tabview_enable_pre_load(tv, true);
gui_tabview_loop_x(tv, true);
gui_tab_t *tb_clock = gui_tab_create(tv, "tb_clock", 0, 0, 0, 0, 0, 0);
gui_tab_t *tb_activity = gui_tab_create(tv, "tb_activity", 0, 0, 0, 0, 1, 0);
gui_tab_t *tb_heart = gui_tab_create(tv, "tb_heart", 0, 0, 0, 0, 2, 0);
gui_tab_t *tb_cube = gui_tab_create(tv, "tb_cube", 0, 0, 0, 0, 3, 0);
gui_tab_t *tb_weather = gui_tab_create(tv, "tb_weather", 0, 0, 0, 0, 5, 0);
gui_tab_t *tb_music = gui_tab_create(tv, "tb_music", 0, 0, 0, 0, 4, 0);
gui_tab_t *tb_ani = gui_tab_create(tv, "tb_ani", 0, 0, 0, 0, 6, 0);
page_tb_clock(gui_tab_get_rte_obj(tb_clock));
page_tb_activity(gui_tab_get_rte_obj(tb_activity));
page_tb_heart(gui_tab_get_rte_obj(tb_heart));
page_tb_cube(gui_tab_get_rte_obj(tb_cube));
page_tb_weather(gui_tab_get_rte_obj(tb_weather));
page_tb_music(gui_tab_get_rte_obj(tb_music));
}
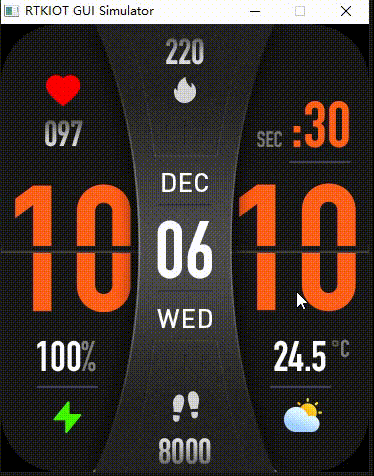
API
Functions
-
gui_tab_t *gui_tab_create(void *parent, const char *name, int16_t x, int16_t y, int16_t w, int16_t h, int16_t idx, int16_t idy)
create a tab widget, which should be nested in a tabview.
- Parameters:
parent – the father widget it nested in.
filename – this tab widget’s name.
x – the X-axis coordinate of the widget.
x – the Y-axis coordinate of the widget.
w – the width of the widget.
h – the hight of the widget.
idx – the X-axis index in a tabview.
idy – the Y-axis index in a tabview.
- Returns:
return the widget object pointer.
-
gui_obj_t *gui_tab_get_rte_obj(gui_tab_t *this)
get run time envriment obj
- Parameters:
this –
- Returns:
gui_obj_t*
-
void gui_tab_set_style(gui_tab_t *this, T_SLIDE_STYLE style)
set style of this tab
- Parameters:
this – tab widget pointer
style – slide style
-
void gui_tab_update_preload(gui_obj_t *obj)
when enable preload, call this API can update preload buffer
- Parameters:
obj –
-
void gui_tab_reduction_fade(gui_obj_t *obj, int16_t tab_x_gap, int16_t tab_y_gap)
- Parameters:
obj –
tab_x_gap –
tab_y_gap –
-
struct gui_tab_id_t
- #include <gui_tab.h>
tab widget ID structure
-
struct gui_tab_t
- #include <gui_tab.h>
tab widget structure
-
struct gui_tab_stacking_t
- #include <gui_tab.h>
tab widget stacking structure