SVG
The SVG widget allows you to display an image in SVG format.
SVG images are vector graphics based on XML language implementation, which can be used to describe 2D vector graphics.
SVG images do not need to be converted into
bin
format files using ImageConverter tools, but can be directly packaged in theroot
directory.
Usage
Creat a svg widget
You can use gui_svg_create_from_mem(parent, name, addr, size, x, y, w, h) to create an SVG widget from memory, or use gui_svg_create_from_file(parent, name, filename, x, y, w, h) to create from file.
This w/h
are the width and height of the SVG widget, not SVG image. This size
is the data size of the SVG image that must be filled in.
SVG rotation
Using the gui_svg_rotation(svg, degrees, c_x, c_y) the image will be rotated by an angle of degree
. The (c_x, c_y)
is relative to the origin of the SVG widget, not the origin of the screen.
SVG scale
If you need the object size to be updated to transformed size set gui_svg_scale(svg, scale_x, scale_y).
SVG translate
The image will be translated by using the gui_svg_translate(svg, t_x, t_y).
Opacity
If you want the image to fade or opacity set gui_svg_set_opacity(svg, opacity_value).
Example
Creat a simple SVG
#include "gui_svg.h"
#include "root_image_hongkong/ui_resource.h"
void page_tb_svg(void *parent)
{
gui_svg_t* svg1=gui_svg_create_from_mem(parent,"svg1",ACTIVITY_SVG,5184,0,0,100,100);
}
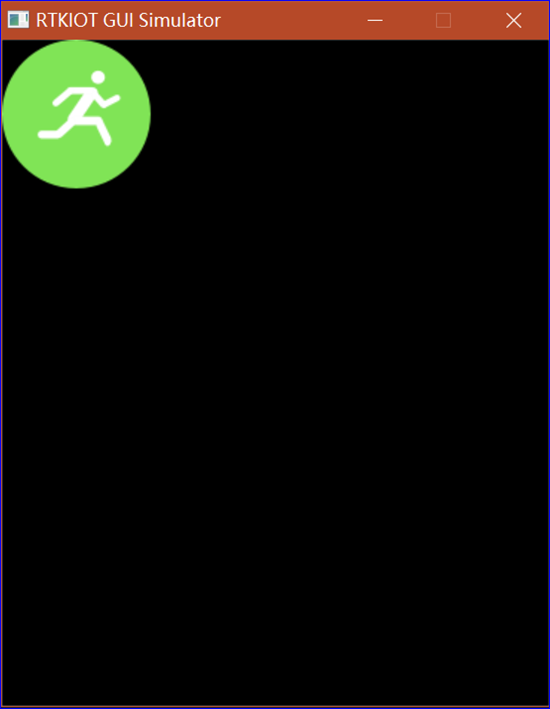
SVG rotation
#include "gui_svg.h"
#include "root_image_hongkong/ui_resource.h"
void page_tb_svg(void *parent)
{
gui_svg_t* svg1=gui_svg_create_from_mem(parent,"svg1",ACTIVITY_SVG,5184,0,0,100,100);
gui_svg_rotation(svg1,90,50,50);
}

SVG scale
#include "gui_svg.h"
#include "root_image_hongkong/ui_resource.h"
void page_tb_svg(void *parent)
{
gui_svg_t* svg1=gui_svg_create_from_mem(parent,"svg1",ACTIVITY_SVG,5184,0,0,100,100);
gui_svg_scale(svg1,2,1);
}
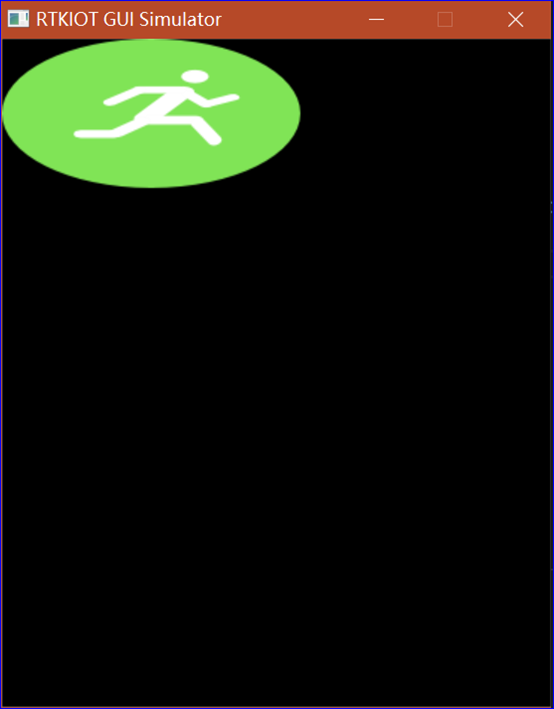
SVG translate
#include "gui_svg.h"
#include "root_image_hongkong/ui_resource.h"
void page_tb_svg(void *parent)
{
gui_svg_t* svg1=gui_svg_create_from_mem(parent,"svg1",ACTIVITY_SVG,5184,0,0,100,100);
gui_svg_translate(svg1,100,100);
}
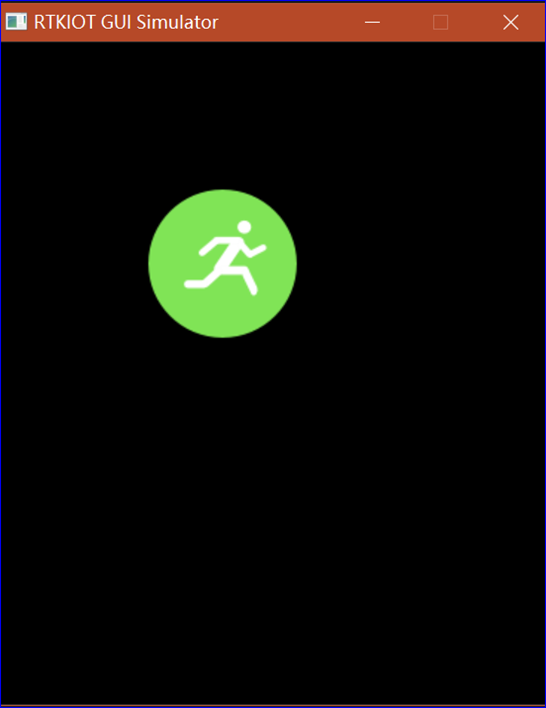
API
Functions
-
gui_svg_t *gui_svg_create_from_file(void *parent, const char *name, const char *filename, int16_t x, int16_t y, int16_t w, int16_t h)
create a svg widget from file,which should be display an SVG image.
- Parameters:
parent – the father widget nested in
name – this svg widget’s name.
filename – this svg image’s filename
x – the X-axis coordinate relative to parent widget
y – the Y-axis coordinate relative to parent widget
w – width
h – height
- Returns:
gui_svg_t*
-
gui_svg_t *gui_svg_create_from_mem(void *parent, const char *name, uint8_t *addr, uint32_t size, int16_t x, int16_t y, int16_t w, int16_t h)
create a svg widget from memory,which should be display an SVG image
- Parameters:
parent – the father widget nested in
name – this svg widget’s name.
addr – change svg address
size – the size of svg
x – the X-axis coordinate relative to parent widget
y – the Y-axis coordinate relative to parent widget
w – width
h – height
- Returns:
gui_svg_t*
-
void gui_svg_rotation(gui_svg_t *svg, float degrees, float c_x, float c_y)
set svg rotation angle
- Parameters:
svg – the svg widget pointer
degrees – rotation angle
c_x – the X-axis coordinate of the rotation center
c_y – the Y-axis coordinate of the rotation center
-
void gui_svg_scale(gui_svg_t *svg, float scale_x, float scale_y)
scale the svg
- Parameters:
svg – the svg widget pointer
scale_x – scale in the X-axis direction
scale_y – scale in the Y-axis direction
-
struct gui_svg_t
- #include <gui_svg.h>
svg structure