STB Image
The image widget is the basic widget used to display image. You can set a left, right and center image, and the center image will be repeated to match the width of the object.STB image widgets support bmp, jpg, png, gif, etc.
In the STB image library, STB is not an acronym; it is actually a naming convention used to identify a series of single-file libraries developed by Sean T. Barrett. The STB image library (stb_image.h)
is one of these libraries and is used to load and store various common image formats.
Usage
Create widget
You can use gui_img_stb_create_from_mem(void *parent, const char *name, void *addr, uint32_t size, uint8_t type, int16_t x, int16_t y); to create an STB image widget from memory.Please confirm that the type and size are correct.
Set attribute
You can use gui_img_stb_set_attribute(gui_stb_img_t *img, void *addr, uint32_t size, uint8_t type, int16_t x, int16_t y); to set the attribute of an STB image widget, replace it with a new file and set a new coordinate.
Example
code
#include "root_image_hongkong/ui_resource.h"
#include "gui_obj.h"
#include "gui_app.h"
#include "gui_img.h"
#include "gui_img_stb.h"
static void app_home_ui_design(gui_app_t *app)
{
gui_stb_img_t *jpg = gui_img_stb_create_from_mem(&app->screen, "jpg", TEST_JPG, 0x6640, JPEG, 0, 0);
gui_stb_img_t *png = gui_img_stb_create_from_mem(&app->screen, "png", TEST_PNG, 0x2B00, PNG, 170, 170);
}
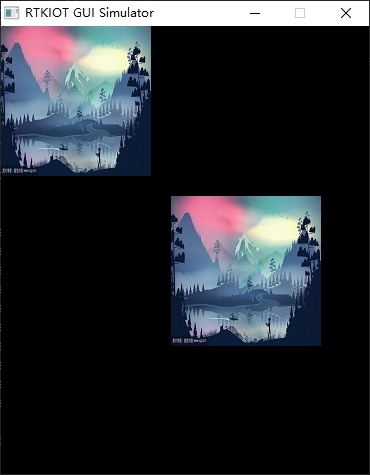
API
Functions
-
void gui_img_stb_set_attribute(gui_stb_img_t *this, void *addr, uint32_t size, GUI_FormatType type, int16_t x, int16_t y)
set stb image widget attribute
- Parameters:
this – stb image widget
addr – image address
size – image file size by Byte
type – image type
x – X-axis coordinate
y – Y-axis coordinate
-
void gui_img_stb_set_attribute_static(gui_stb_img_t *this, void *addr, uint32_t size, GUI_FormatType type, int16_t x, int16_t y)
set stb image widget attribute without free rgb data
- Parameters:
this – stb image widget
addr – image address
size – image file size by Byte
type – image type
x – X-axis coordinate
y – Y-axis coordinate
-
gui_stb_img_t *gui_img_stb_create_from_mem(void *parent, const char *name, void *addr, uint32_t size, GUI_FormatType input_type, GUI_FormatType output_type, int16_t x, int16_t y)
Creat a image widget with buffer.
Note
This widget is use to display image which needs to decode.
Note
The data of image can be static char or data transformed by BLE.
- Parameters:
parent – The father widget which the scroll text nested in.
name – The widget’s name.
addr – The data address of image.
size – The data size of image.The unit is bytes.
input_type – The input type of image. bmp 11, jpeg 12, png 13,
output_type – The output type of image. RGB565 RGB888,
x – The X-axis coordinate of the text box.
x – The Y-axis coordinate of the text box.
- Returns:
gui_stb_img_t*
-
void gui_img_stb_set_output_format(gui_stb_img_t *this, GUI_FormatType output)
set output_format
Note
RGB565 or RGB888
- Parameters:
this – stb image widget
output – output format
-
struct gui_gif_info_t
- #include <gui_img_stb.h>
stb img widget gif information structure
-
struct gui_stb_img_t
- #include <gui_img_stb.h>
stb img widget information structure
-
struct IODEV
- #include <gui_img_stb.h>