Multi level
This widget can be used in GUI applications that require managing complex hierarchical structures, such as nested menu structures and layered display objects. With this widget, developers can flexibly manage and manipulate multi-level subwindows, and achieve the hiding and displaying of specific level and order objects, greatly enhancing the dynamism and interactivity of the interface.
Usage
Create widget
Creating function is gui_multi_level_t *gui_multi_level_create(void *parent, const char *widget_name, void (*ui_design)(gui_obj_t *)). This function creates and initializes a new instance of the gui_multi_level_t widget.
Jump to (level, index)
Function is gui_multi_level_jump(gui_multi_level_t *this, int level, int index). This function facilitates the jump operation to a specific level and index within the multi-level GUI structure.
Example
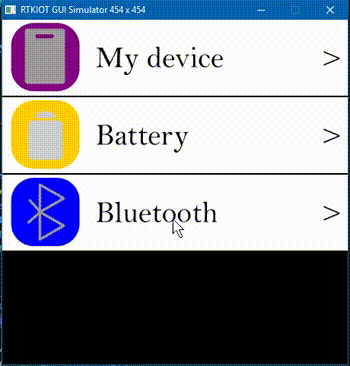
Create multi levels in 0~2 level, according to the nested structure.
GUI_APP_ENTRY(APP_SETTING)
{
gui_multi_level_t *m0 = gui_multi_level_create(GUI_APP_ROOT_SCREEN, 0, ui_design_0);//main
gui_multi_level_t *m1_0 = gui_multi_level_create(m0, 0, ui_design_1_0);//mydevice
gui_multi_level_t *m1_1 = gui_multi_level_create(m0, 0, ui_design_1_1);//battery
gui_multi_level_t *m1_2 = gui_multi_level_create(m0, 0, ui_design_1_2);//bluetooth
gui_multi_level_t *m2_0 = gui_multi_level_create(m1_0, 0, ui_design_2_0);//rename
gui_multi_level_t *m2_1 = gui_multi_level_create(m1_0, 0, ui_design_2_1);//storage
}
The ui_design_xx function will create the dispaly in the specific (level, index) Multi level window, when this window is displayed.
#define HIGHLIGHT_BLUE gui_rgb(0, 100, 255)
struct button_args
{
gui_multi_level_t *parent;
unsigned char index;
unsigned char level;
};
static void multi_levle_button_cb(gui_obj_t *this, void *event, struct button_args *args)
{
GUI_API(gui_multi_level_t).jump(args->parent, args->level, args->index);
}
static void ui_design_0(gui_obj_t *parent)
{
{
gui_button_t *button = gui_button_create(parent, 0, 0, 454, 100, MYDEVICE_BIN, MYDEVICEHL_BIN, 0, 0,
0);
static struct button_args args;
args.parent = (void *)parent;
args.level = 1;
args.index = 0;
GUI_API(gui_button_t).on_click(button, (gui_event_cb_t)multi_levle_button_cb, &args);
}
{
gui_button_t *button = gui_button_create(parent, 0, 102 * 1, 454, 100, BATTERY_BIN, BATTERYHL_BIN,
0, 0, 0);
static struct button_args args;
args.parent = (void *)parent;
args.level = 1;
args.index = 1;
GUI_API(gui_button_t).on_click(button, (gui_event_cb_t)multi_levle_button_cb, &args);
}
{
gui_button_t *button = gui_button_create(parent, 0, 102 * 2, 454, 100, BLUETOOTH_BIN,
BLUETOOTHHL_BIN, 0, 0, 0);
static struct button_args args;
args.parent = (void *)parent;
args.level = 1;
args.index = 2;
GUI_API(gui_button_t).on_click(button, (gui_event_cb_t)multi_levle_button_cb, &args);
}
gui_return_create(parent, gui_app_return_array,
sizeof(gui_app_return_array) / sizeof(uint32_t *), win_cb, (void *)0);
}
API
Functions
-
gui_multi_level_t *gui_multi_level_create(void *parent, const char *widget_name, void (*ui_design)(gui_obj_t*))
create a multi_level widget.
- Parameters:
parent – the father widget nested in.
widget_name – the widget name.
x – the X-axis coordinate.
y – the Y-axis coordinate.
ui_design – ui_design function pointer.
- Returns:
return the widget object pointer
-
void gui_multi_level_jump(gui_multi_level_t *this, int level, int index)
jump to a specific multi_level widget.
- Parameters:
this – one of the multi_level widgets in app.
level – specitf level. 0,1,2…
index – index in this level. 0,1,2…
Variables
-
void (*jump)(gui_multi_level_t *this, int levle, int index)
jump to a specitf multi_level widget.
- Param this:
one of the multi_level widgets in app.
- Param level:
specitf level. 0,1,2…
- Param index:
index in this level. 0,1,2…
-
struct gui_multi_level_t
- #include <gui_multi_level.h>
multi_level structure