Text widget
The text widget is the basic widget used to display text, which can be used to output text in different fonts, different colors, and different sizes to the screen. In order to draw text, the font file can be standard .ttf file or customized .bin file.
Features
Text widgets can support the following features.
UTF-8 support
Multi language support
Text typesetting support
Word wrap and texts scrolling
Anti-aliasing
Multi fonts support
Multi font sizes support
Thirty-two bit true color support
Custom animation effects support
Standrads TTF file support[1]
Self-developed font files support
Usage
Using functions to load font files and display text
Initialize the font file
In order to draw text, font files containing glyph information need to be loaded into the system.
The font file can be standard .ttf file or customized .bin file. The font file can be initialized ahead of time to avoid having to set the font type for each text widget.
To initialize the new version customized bin font file, you need to use gui_font_mem_init(font_bin_addr) .
To initialize the standrad TTF file to draw text, you need to use gui_font_stb_init(font_ttf_addr) .
All customized bin font files are available from RTK technicians.
FONT_BIN
,FONT_TTF
are all address of the files stored in flash.
Create text widget
To create a text widget, you can use gui_text_create(parent, filename, x, y, w, h), The coordinates on the screen and text box size have been identified after create. These attributes also can be modified whenever you want.
Note that text box size should be larger than the string to be shown, out-of-range text will be hidden.
Set text attributes
Set text
To add some texts or characters to a text widget and set text attributes with: gui_text_set(this, text, text_type, color, length, font_size)
Note that text length must be the same as the set character length, text frontsize should must be the same as the type size
Font type
Text widget support the type setting. You can use this function to set type.Type is bin/ttf file address. gui_text_type_set(this, type).
Text content
This interface can be used to set the content that needs to be displayed by the text widget. gui_text_content_set(this, text, length).
Text encoding
The text widget supports both UTF-8 encoding and UTF-16 encoding input formats, and this interface can be used to change the decoding method. gui_text_encoding_set(this, charset).
Convert to img
By using this interface, the text in the text widget will be converted into an image, stored in memory, and rendered using the image. It also supports image transformations such as scaling and rotation. This only applies to bitmap fonts. gui_text_convert_to_img(this, font_img_type). Because the content and font size information of the text widget is needed, it should be called after set text.If the content, font size, position and other attributes of the text have been modified, you need to reuse this interface for conversion.
Text input
Text widget support the input setting. You can use this function to set input gui_text_input_set(this, inputable).
Text click
Text widget support click. You can use this function to add the click event for text gui_text_click(this, event_cb, parameter).
Text mode
Text widget support seven typesetting modes, to set text typesetting mode with: gui_text_mode_set(this, mode).
All typesetting modes are as follows.
Type |
Line Type |
X Direction |
Y Direction |
Widget |
---|---|---|---|---|
|
Single-line |
Left |
Top |
Text widget. Default. |
|
Single-line |
Center |
Top |
Text widget. |
|
Single-line |
Right |
Top |
Text widget. |
|
Multi-line |
Left |
Top |
Text widget. |
|
Multi-line |
Center |
Top |
Text widget. |
|
Multi-line |
Right |
Top |
Text widget. |
|
Multi-line |
Left |
Mid |
Text widget. |
|
Multi-line |
Center |
Mid |
Text widget. |
|
Multi-line |
Right |
Mid |
Text widget. |
|
Single-line |
Right to Left |
Top |
Scroll text widget. |
|
Multi-line |
Left |
Bottom to Top |
Scroll text widget. |
|
Multi-line |
Right |
Top to Bottom |
Scroll text widget. |
|
Multi-line |
Left |
Top to Bottom |
Text widget. |
|
Multi-line |
Right |
Bottom to Top |
Text widget. |
typedef enum
{
/* TOP */
LEFT = 0x00,
CENTER = 0x01,
RIGHT = 0x02,
MULTI_LEFT = 0x03,
MULTI_CENTER = 0x04,
MULTI_RIGHT = 0x05,
/* MID */
MID_LEFT = 0x10,
MID_CENTER = 0x11,
MID_RIGHT = 0x12,
/* SCROLL */
SCROLL_X = 0x30,
SCROLL_Y = 0x31,
SCROLL_Y_REVERSE = 0x32,
/* VERTICAL */
VERTICAL_LEFT = 0x40,
VERTICAL_RIGHT = 0x41,
} TEXT_MODE;
Text move
You can use this function gui_text_move(this, x, y) to move text to a specified location, but x and y cannot be larger than w and h of the text
Set animate
Using this function gui_text_set_animate(o, dur, repeat_count, callback, p) to set the animation and implement the animation effect in the corresponding callback function
Example
Example Multiple text widget
An example of the multiple text widget is shown below.
Example code
#include "string.h"
#include "gui_obj.h"
#include "guidef.h"
#include "gui_text.h"
#include "draw_font.h"
#include "gui_app.h"
#include "rtk_gui_resource.h"
static char chinese[6] =
{
0xE4, 0xB8, 0xAD,
0xE6, 0x96, 0x87
};
static void app_launcher_ui_design(gui_app_t *app)
{
gui_font_mem_init(HARMONYOS_SIZE24_BITS1_FONT_BIN);
gui_font_mem_init(HARMONYOS_SIZE16_BITS4_FONT_BIN);
gui_font_mem_init(HARMONYOS_SIZE32_BITS1_FONT_BIN);
gui_font_mem_init(SIMKAI_SIZE24_BITS4_FONT_BIN);
void *screen = &app->screen;
gui_text_t *text1 = gui_text_create(screen, "text1", 10, 10, 100, 50);
gui_text_set(text1, chinese, GUI_FONT_SRC_BMP, APP_COLOR_WHITE, strlen(chinese), 24);
gui_text_type_set(text1, HARMONYOS_SIZE24_BITS1_FONT_BIN);
gui_text_mode_set(text1, LEFT);
gui_text_t *text2 = gui_text_create(screen, "text2", 0, 50, 300, 50);
gui_text_set(text2, "english", GUI_FONT_SRC_BMP, APP_COLOR_RED, 7, 16);
gui_text_type_set(text2, HARMONYOS_SIZE16_BITS4_FONT_BIN);
gui_text_mode_set(text2, LEFT);
char *string = "TEXT_WIDGET";
gui_text_t *text3 = gui_text_create(screen, "text3", 0, 90, 300, 50);
gui_text_set(text3, string, GUI_FONT_SRC_BMP, APP_COLOR_BLUE, strlen(string), 32);
gui_text_type_set(text3, HARMONYOS_SIZE32_BITS1_FONT_BIN);
gui_text_mode_set(text3, CENTER);
gui_text_t *text4 = gui_text_create(screen, "text4", 0, 150, 100, 200);
gui_text_set(text4, "ABCDEFGHIJKLMNOPQRSTUVWXYZ", GUI_FONT_SRC_BMP, gui_rgb(0, 0xff, 0xff), 24, 24);
gui_text_type_set(text4, SIMKAI_SIZE24_BITS4_FONT_BIN);
gui_text_mode_set(text4, MULTI_CENTER);
}
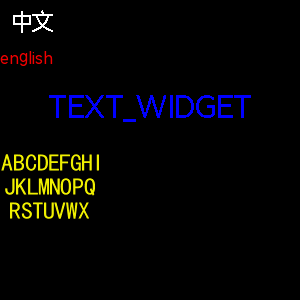
Example Animate text widget
An example of the animate text widget is shown below.
Example code
#include "root_image_hongkong/ui_resource.h"
#include "string.h"
#include "gui_obj.h"
#include "guidef.h"
#include "gui_text.h"
#include "draw_font.h"
void change_text_cb(gui_text_t *obj)
{
if (obj->animate->current_frame > 0 && obj->animate->current_frame < 50)
{
gui_text_move(obj, 50, 150);
obj->utf_8 = "123456789";
}
else if (obj->animate->current_frame > 50 && obj->animate->current_frame < 100)
{
gui_text_move(obj, 200, 150);
obj->utf_8 = "987654321";
}
else
{
gui_text_move(obj, 125, 50);
obj->utf_8 = "abcdefghi";
}
}
void page_tb_activity(void *parent)
{
gui_font_mem_init(SIMKAI_SIZE24_BITS4_FONT_BIN);
gui_text_t *text = gui_text_create(parent, "text", 0, 0, 100, 200);
gui_text_set(text, "ABCDEFGHI", GUI_FONT_SRC_BMP, APP_COLOR_RED, 9, 24);
gui_text_type_set(text, SIMKAI_SIZE24_BITS4_FONT_BIN);
gui_text_mode_set(text, MULTI_CENTER);
gui_text_set_animate(text, 5000, 15, change_text_cb, text);
}
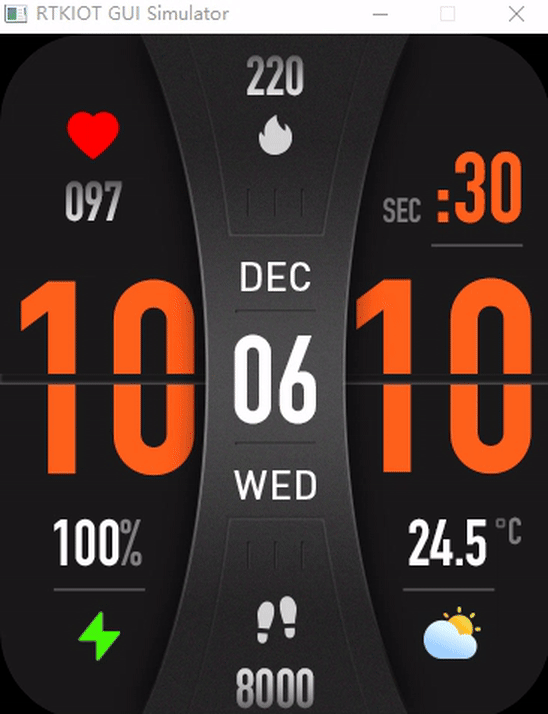
API
Enums
-
enum TEXT_MODE
text mode enum start
Values:
-
enumerator LEFT
-
enumerator CENTER
-
enumerator RIGHT
-
enumerator MULTI_LEFT
-
enumerator MULTI_CENTER
-
enumerator MULTI_RIGHT
-
enumerator MID_LEFT
-
enumerator MID_CENTER
-
enumerator MID_RIGHT
-
enumerator SCROLL_X
-
enumerator SCROLL_Y
-
enumerator SCROLL_Y_REVERSE
-
enumerator VERTICAL_LEFT
-
enumerator VERTICAL_RIGHT
-
enumerator LEFT
-
enum TEXT_CHARSET
text mode enum end
text encoding format enum
Values:
-
enumerator UTF_8
-
enumerator UTF_16
-
enumerator UTF_16LE
-
enumerator UNICODE_ENCODING
-
enumerator UTF_16BE
-
enumerator UTF_8
Functions
-
void gui_text_click(gui_text_t *this_widget, gui_event_cb_t event_cb, void *parameter)
set textbox click event cb .
- Parameters:
this_widget – text widget
event_cb – cb function
parameter – cb parameter
-
void gui_text_set(gui_text_t *this_widget, void *text, FONT_SRC_TYPE text_type, gui_color_t color, uint16_t length, uint8_t font_size)
set the string in a text box widget.
Note
The font size must match the font file!
- Parameters:
this_widget – the text box widget pointer.
text – the text string.
text_type – text type.
color – the text’s color.
length – the text string’s length.
font_size – the text string’s font size.
- Returns:
void
-
void gui_text_set_animate(void *o, uint32_t dur, int repeat_count, void *callback, void *p)
set animate
- Parameters:
o – text widget
dur – durtion. time length of the animate
repeat_count – 0:one shoot -1:endless
callback – happens at every frame
p – callback’s parameter
-
void gui_text_mode_set(gui_text_t *this_widget, TEXT_MODE mode)
set text mode of this_widget text widget
Note
if text line count was more than one, it will display on the left even if it was set lft or right
- Parameters:
this_widget – the text widget pointer.
mode – there was three mode: LEFT, CENTER and RIGHT.
-
void gui_text_input_set(gui_text_t *this_widget, bool inputable)
set inputable
- Parameters:
this_widget – he text box widget pointer.
inputable – inputable
-
void gui_text_move(gui_text_t *this_widget, int16_t x, int16_t y)
move the text widget
- Parameters:
this_widget – the text box widget pointer.
x – the X-axis coordinate of the text box.
y – the Y-axis coordinate of the text box.
-
void gui_text_size_set(gui_text_t *this_widget, uint8_t height, uint8_t width)
set font size or width and height
Note
if use freetype, width and height is effective, else height will be applied as font size
- Parameters:
this_widget – the text widget pointer.
height – font height or font size.
width – font width(only be effective when freetype was used).
-
void gui_text_font_mode_set(gui_text_t *this_widget, FONT_SRC_MODE font_mode)
set text font mode
- Parameters:
this_widget – the text widget pointer
font_mode – font source mode
-
void gui_text_type_set(gui_text_t *this_widget, void *font_source, FONT_SRC_MODE font_mode)
set font type
Note
The type must match the font size!
- Parameters:
this_widget – the text widget pointer
font_source – the addr of .ttf or .bin
font_mode – font source mode
-
void gui_text_encoding_set(gui_text_t *this_widget, TEXT_CHARSET charset)
set font encoding
Note
utf-8 or unicode
- Parameters:
this_widget – the text widget pointer
encoding_type – encoding_type
-
void gui_text_content_set(gui_text_t *this_widget, void *text, uint16_t length)
set text content
- Parameters:
this_widget – the text widget pointer
text – the text string.
length – the text string’s length
-
void gui_text_convert_to_img(gui_text_t *this_widget, GUI_FormatType font_img_type)
to draw text by img, so that text can be scaled
- Parameters:
this_widget – the text widget pointer
font_img_type – color format
-
gui_text_t *gui_text_create(void *parent, const char *name, int16_t x, int16_t y, int16_t w, int16_t h)
create a text box widget.
Note
The area of the text box should be larger than that of the string to be shown, otherwise, part of the text will be hidden.
- Parameters:
parent – the father widget which the text nested in.
filename – the widget’s name.
x – the X-axis coordinate of the text box.
y – the Y-axis coordinate of the text box.
w – the width of the text box.
h – the hight of the text box.
- Returns:
return the widget object pointer
-
struct gui_text_t
- #include <gui_text.h>
text widget structure
Public Members
-
gui_color_t color
-
uint16_t len
-
uint16_t font_len
-
int16_t char_width_sum
-
int16_t char_height_sum
-
int16_t char_line_sum
-
TEXT_CHARSET charset
-
FONT_SRC_TYPE font_type
-
FONT_SRC_MODE font_mode
-
uint8_t font_height
-
uint8_t inputable
-
uint8_t checksum
-
bool refresh
-
gui_animate_t *animate
-
void *content
-
void *data
-
void *path
address or path
-
int16_t offset_x
-
int16_t offset_y
-
gui_color_t color
-
struct gui_text_line_t
- #include <gui_text.h>
text line structure