Qrcode
The qbcode widget is the widget used to create or display qrcode and barcode. You can set a border, location and size attribute for qbcode widget. Qbcode widget support text and binary data for qrcode encode, and support support text for barcode encode. The qrcode widget the QR Code Model 2 specification, supporting all versions (sizes) from 1 to 40, all 4 error correction levels, and 4 character encoding modes. The widget support fout error correction level in a QR Code symbol, and the high level is set by default. Barcode support code128 format.
Usage
create qrcode widget
To create a QR code or barcode control, you can use the function gui_qbcode_t *gui_qbcode_create(void *parent, const char *name, int16_t x, int16_t y, int16_t w,int16_t h,T_QBCODE_DISPLAY_TYPE type,T_QBCODE_ENCODE_TYPE encodeType). Whether a QR code or barcode is created depends on the encodeType parameter. The encodeType enumeration types are as follows:
typedef enum
{
QRCODE_ENCODE_TEXT,
QRCODE_ENCODE_BINARY,
BARCODE_ENCODE_TEXT,
} T_QBCODE_ENCODE_TYPE;
config qrcode size and border
void gui_qbcode_config(gui_qbcode_t *qbcode, uint8_t *data, uint32_t data_len, uint8_t border_size)
qrcode encode notes
The encoded data for qrcode can exceeded the max bytes by default, which can modify the max version is 15; You can modify the version according to the document: https://www.qrcode.com/zh/about/version.html;
Example
sample code
#include "gui_qbcode.h"
#define QRCODE_WIDTH 320
#define QRCODE_HEIGHT 320
#define DISPLAY_TYPE QRCODE_DISPLAY_IMAGE //QRCODE_DISPLAY_SECTION
#define ENCODED_TYPE QRCODE_ENCODE_TEXT // QRCODE_ENCODE_BINARY
char* str = "123455678901234567890123455678901234567890123455678901234567890123455678901234567890123455678901234567890";
gui_qbcode_t * qrcode = gui_qbcode_create(parent,
"qrcode",
454/2 - QRCODE_WIDTH/2,
454/2 - QRCODE_HEIGHT/2,
QRCODE_WIDTH,
QRCODE_HEIGHT,
DISPLAY_TYPE,
ENCODED_TYPE);
gui_qbcode_config(qrcode, str, strlen(str), 3);
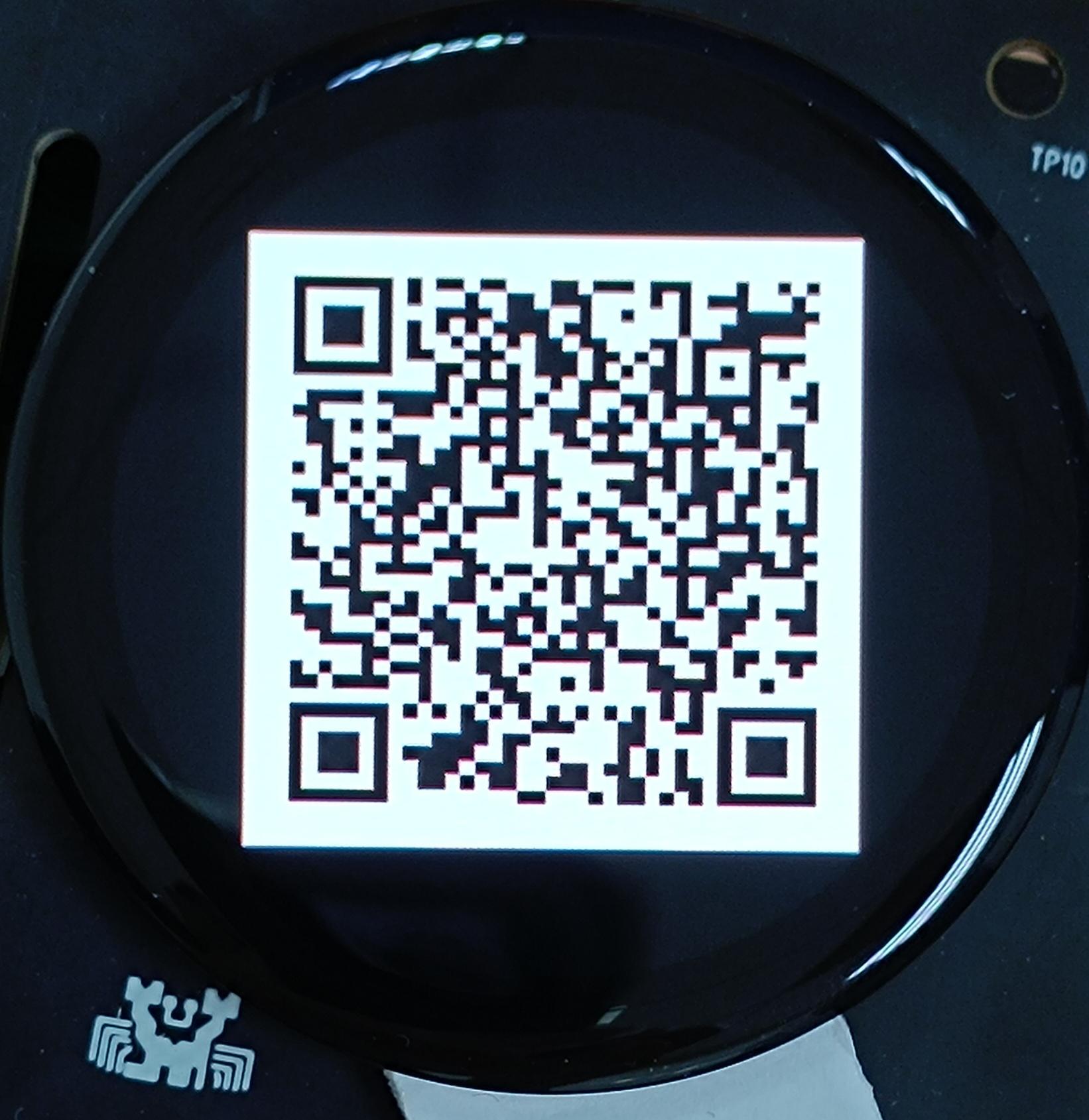
sample code
#define BARCODE_WIDTH (143 * 3)
#define BARCODE_HEIGHT 143
#define DISPLAY_TYPE BARCODE_DISPLAY_IMAGE //BARCODE_DISPLAY_SECTION
#define ENCODED_TYPE BARCODE_ENCODE_TEXT
char* str = "1234567890";
gui_qbcode_t * qrcode = gui_qbcode_create(parent,
"qrcode",
454/2 - BARCODE_WIDTH/2,
454/2 - BARCODE_HEIGHT/2,
BARCODE_WIDTH,
BARCODE_HEIGHT,
DISPLAY_TYPE,
ENCODED_TYPE);
gui_qbcode_config(qrcode, str, strlen(str) + 1, 10);
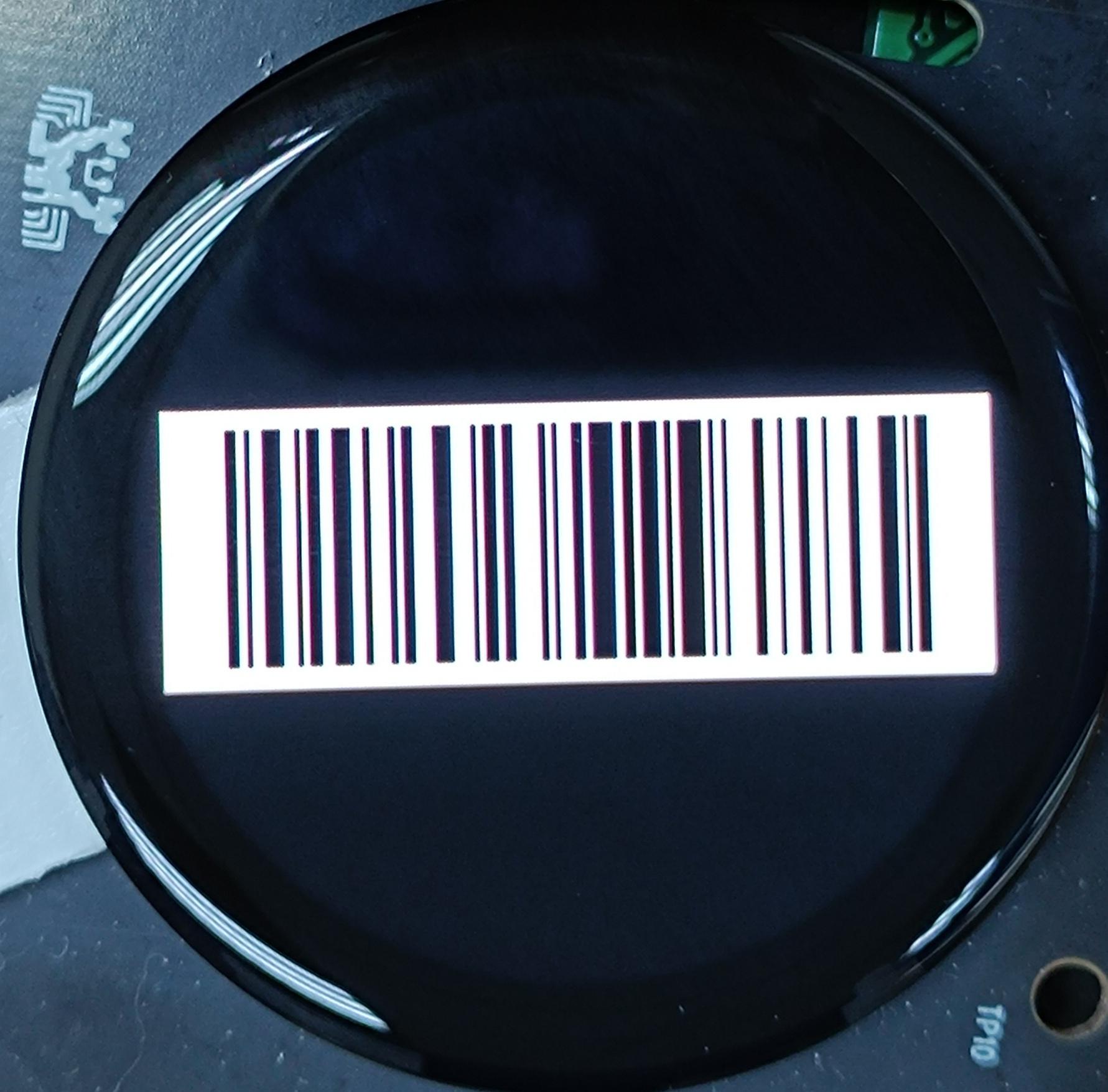
API
Enums
Functions
-
gui_qbcode_t *gui_qbcode_create(void *parent, const char *name, int16_t x, int16_t y, int16_t w, int16_t h, T_QBCODE_DISPLAY_TYPE type, T_QBCODE_ENCODE_TYPE encodeType)
Create a qrcode widget.
Note
this function just create a qrcode object, qbcode data and param should be config by gui_qbcode_config() api.
- Parameters:
parent – the father widget
filename – this qrcode widget’s name.
x – the X-axis coordinate relative to parent widget
y – the Y-axis coordinate relative to parent widget
w – qrcode image display width including border
h – qrcode image display height including border
type – QRCODE_DISPLAY_SECTION (gui in real-time) or QRCODE_DISPLAY_IMAGE(save in psRAM by default) or BARCODE_DISPLAY_SECTION or BARCODE_DISPLAY_IMAGE.
encodeType – QRCODE_ENCODE_TEXT or QRCODE_ENCODE_BINARY or BARCODE_ENCODE_TEXT supported.
- Returns:
gui_qbcode_t* success, NULL failed.
-
void gui_qbcode_config(gui_qbcode_t *qbcode, uint8_t *data, uint32_t data_len, uint8_t border_size)
config qbcode data and border param for a qbcode object.
- Parameters:
qbcode – a qbcode object pointer.
data – input data encoded for qbcode
data_len – input data length for encode data
border_size – qrode border size, can be 1, 2, 3… by qbcode size, default white color border;
- Returns:
null
-
struct gui_qbcode_t
- #include <gui_qbcode.h>
qrcode widget structure