LPC
LPC Demo Code Support List
This chapter introduces the details of the LPC demo code.
LPC Demo for Comparator Function
The description of LPC demo code 1 is shown in the following table.
Demo 1 |
|
---|---|
Sample Purpose |
Demonstrate LPC comparator function. |
File Path |
|
Function Entry |
|
Hardware Connection |
|
Expected Result |
Adjust the DC voltage source repeatedly, when the input voltage on M0_0 is detected to be higher than 400mV for 4 times, the string lpc_rtc_handler: lpc_counter_value 4 will be printed on Debug Analyzer. |
LPC Demo for Voltage Detection (Input Below Threshold)
The description of LPC demo code 2 is shown in the following table.
Demo 2 |
|
---|---|
Sample Purpose |
Demonstrate LPC voltage detection function. |
File Path |
|
Function Entry |
|
Hardware Connection |
Connect M0_0 of EVB to an external DC voltage source. |
Expected Result |
Adjust the DC voltage source, when the input voltage on M0_0 is detected to be lower than 800mV for 6 times, the string lpc_handler: lpc_counter_value 6 will be printed on Debug Analyzer. |
LPC Demo for Voltage Detection (Input Over Threshold)
The description of LPC demo code 3 is shown in the following table.
Demo 3 |
|
---|---|
Sample Purpose |
Demonstrate LPC voltage detection function. |
File Path |
|
Function Entry |
|
Hardware Connection |
Connect M0_0 of EVB to an external DC voltage source. |
Expected Result |
Adjust the DC voltage source, when the input voltage on M0_0 is detected to be higher than 1200mV for 5 times, the string lpc_rtc_handler: lpc_counter_value 5 will be printed on Debug Analyzer. |
Functional Overview
LPC can be used for voltage detection and counting. When the LPC detects that the input voltage exceeds the set voltage threshold, the system will trigger the interrupt. LPC can be used to count the number of times the input voltage exceeds the set voltage threshold. When the count value exceeds the set value of the comparator, the interrupt will be triggered.
Program Examples
Comparator Function Operation Flow
LPC comparator function initialization flow is shown in the following figure.
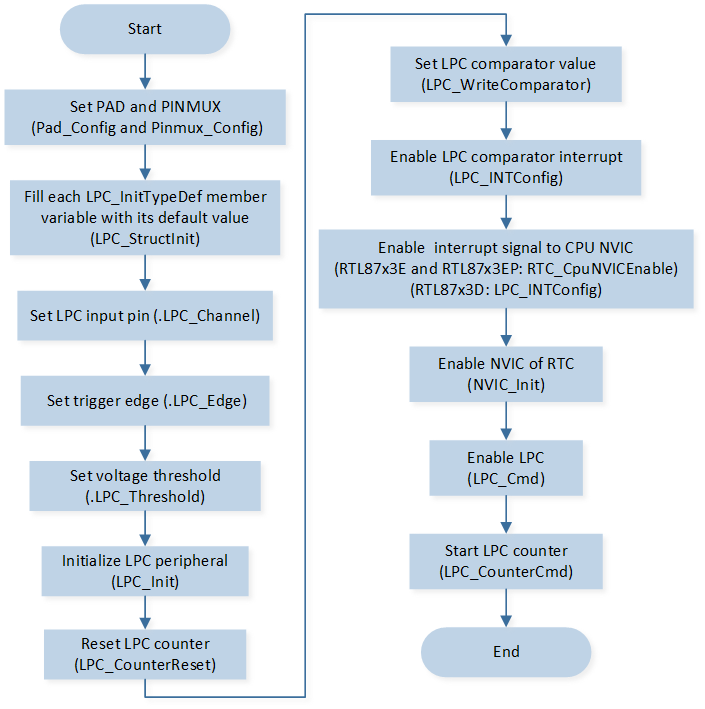
LPC Comparator Function Initialization Flow Chart
The codes below demonstrate the LPC comparator function initialization flow.
void driver_lpc_init(void)
{
LPC_InitTypeDef LPC_InitStruct;
LPC_StructInit(&LPC_InitStruct);
LPC_InitStruct.LPC_Channel = LPC_CAPTURE_PIN;
LPC_InitStruct.LPC_Edge = LPC_Vin_Over_Vth;
LPC_InitStruct.LPC_Threshold = LPC_400_mV;
LPC_Init(&LPC_InitStruct);
LPC_CounterReset();
LPC_WriteComparator(LPC_COMP_VALUE);
LPC_INTConfig(LPC_INT_COUNT_COMP, ENABLE);
#if (TARGET_RTL87X3E == 1 || CONFIG_SOC_SERIES_RTL8773E == 1)
RTC_CpuNVICEnable(ENABLE);
RamVectorTableUpdate(RTC_VECTORn, lpc_rtc_handler);
/* Config LPC interrupt */
NVIC_InitTypeDef NVIC_InitStruct;
NVIC_InitStruct.NVIC_IRQChannel = RTC_IRQn;
NVIC_InitStruct.NVIC_IRQChannelPriority = 3;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStruct);
#else
LPC_INTConfig(LPC_INT_VOLTAGE_COMP, ENABLE);
RamVectorTableUpdate(LPCOMP_VECTORn, lpc_rtc_handler);
/* Config LPC interrupt */
NVIC_InitTypeDef NVIC_InitStruct;
NVIC_InitStruct.NVIC_IRQChannel = LPCOMP_IRQn;
NVIC_InitStruct.NVIC_IRQChannelPriority = 3;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStruct);
#endif
LPC_Cmd(ENABLE);
LPC_CounterCmd(ENABLE);
}
LPC comparator function interrupt handle flow is shown in the figure below.
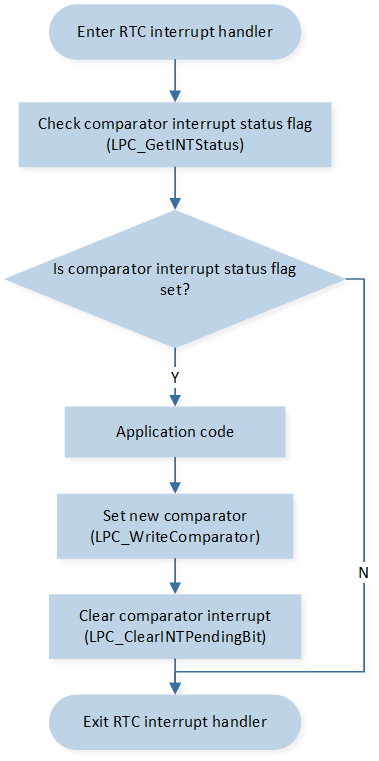
LPC Comparator Function Interrupt Handle Flow Chart
The codes below demonstrate the LPC comparator function interrupt handle flow.
void lpc_rtc_handler(void)
{
uint16_t lpc_counter_value = 0;
IO_PRINT_INFO0("lpc_rtc_handler");
/* LPC counter comparator interrupt */
if (LPC_GetINTStatus(LPC_INT_COUNT_COMP) == SET)
{
lpc_counter_value = LPC_ReadCounter();
IO_PRINT_INFO1("lpc_rtc_handler: lpc_counter_value %d", lpc_counter_value);
LPC_WriteComparator(lpc_counter_value + LPC_COMP_VALUE);
LPC_ClearINTPendingBit(LPC_INT_COUNT_COMP);
}
}
Voltage Detection Function Operation Flow
The codes below demonstrate the LPC voltage detection function initialization flow.
void driver_lpc_init(void)
{
LPC_InitTypeDef LPC_InitStruct;
LPC_StructInit(&LPC_InitStruct);
LPC_InitStruct.LPC_Channel = LPC_CAPTURE_PIN;
LPC_InitStruct.LPC_Edge = LPC_Vin_Below_Vth;
LPC_InitStruct.LPC_Threshold = LPC_800_mV;
LPC_Init(&LPC_InitStruct);
LPC_CounterReset();
LPC_WriteComparator(LPC_COMP_VALUE);
LPC_INTConfig(LPC_INT_COUNT_COMP, ENABLE);
#if (TARGET_RTL87X3E == 1 || CONFIG_SOC_SERIES_RTL8773E == 1)
RTC_CpuNVICEnable(ENABLE);
RamVectorTableUpdate(RTC_VECTORn, lpc_handler);
/* Config LPC interrupt */
NVIC_InitTypeDef NVIC_InitStruct;
NVIC_InitStruct.NVIC_IRQChannel = RTC_IRQn;
NVIC_InitStruct.NVIC_IRQChannelPriority = 3;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStruct);
#else
LPC_INTConfig(LPC_INT_VOLTAGE_COMP, ENABLE);
RamVectorTableUpdate(LPCOMP_VECTORn, lpc_handler);
/* Config LPC interrupt */
NVIC_InitTypeDef NVIC_InitStruct;
NVIC_InitStruct.NVIC_IRQChannel = LPCOMP_IRQn;
NVIC_InitStruct.NVIC_IRQChannelPriority = 3;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStruct);
#endif
LPC_Cmd(ENABLE);
LPC_CounterCmd(ENABLE);
}
The codes below demonstrate the LPC voltage detection function interrupt handle flow.
void lpc_handler(void)
{
if (LPC_GetINTStatus(LPC_INT_COUNT_COMP) == SET)
{
IO_PRINT_INFO1("lpc_handler: lpc_counter_value %d", LPC_ReadCounter());
LPC_CounterReset();
LPC_ClearINTPendingBit(LPC_INT_COUNT_COMP);//Add Application code here
//Add Application code here
}
}